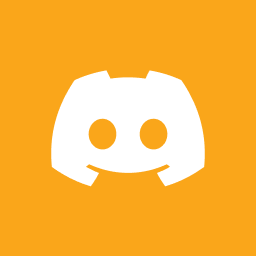
Hi there!
I am currently trying to use the Dart SDK but am quite new to Dart, Flutter and Appwrite. I got some programming experience though.
Do I need to manually create and refresh JWT tokens, or is that automatically done by the SDK? The documentation shows nothing about createJWT
and setJWT
.
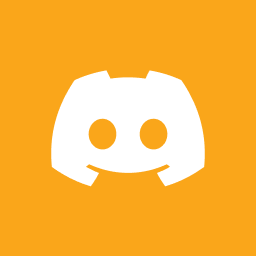
I wonder if I wrote this here now in vain:
import 'dart:developer';
import 'package:appwrite/appwrite.dart';
import 'package:appwrite/models.dart';
import 'package:notes_tasks/appwrite/account.dart';
import 'client.dart';
final Database database = Database(client);
class Database extends Databases {
static const databaseId = '6566589714f646a1b072';
static const notesCollectionId = '656658c1d82904d25cde';
static Database? _instance;
Database._private(Client client) : super(client);
factory Database(Client client) {
_instance ??= Database._private(client);
return _instance!;
}
Future<T> wrap<T>(Future<T> Function() closure) async {
try {
return await closure();
} catch (e) {
inspect(e);
try {
var jwt = await account.createJWT();
client.setJWT(jwt.jwt);
return await closure();
} catch (e) {
inspect(e);
// TODO: Rather check error type string.
if (e is AppwriteException && e.code == 401) {
// TODO: Create and send a Bloc event, listen to it in view and redirect to login screen.
rethrow;
}
throw Exception('Unexpected error while refreshing JWT.'
'Check the database configuration.');
}
}
}
@override
Future<DocumentList> listDocuments({
String databaseId = databaseId,
required String collectionId,
List<String>? queries,
}) async {
return wrap(() => super.listDocuments(
databaseId: databaseId,
collectionId: collectionId,
queries: queries,
));
}
@override
Future<Document> getDocument({
String databaseId = databaseId,
required String collectionId,
required String documentId,
List<String>? queries,
}) {
return wrap(() => super.getDocument(
databaseId: databaseId,
collectionId: collectionId,
documentId: documentId,
queries: queries,
));
}
}
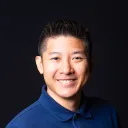
For auth, have you looked and done this? https://appwrite.io/docs/products/auth/quick-start
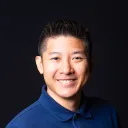
btw, if you're interested, we streamed building a flutter app from scratch here: https://www.youtube.com/@FreshFullStack/videos
The video where we start on mobile is here: https://www.youtube.com/watch?v=4cahiFt_bAg
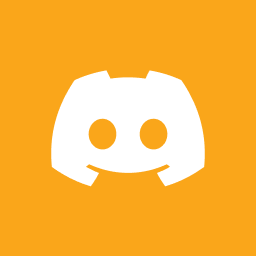
Great, thanks for the videos.
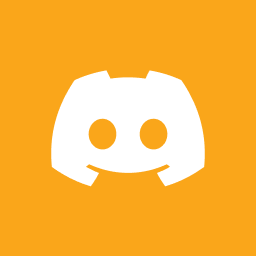
Yes, I did that. I have a registration and login form. So no manual JWT token handling is required, once logged in?
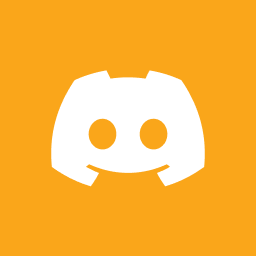
One thing I just noticed is, that I can make DB requests without logging into the account.
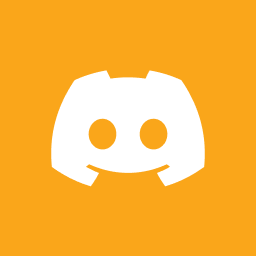
I will watch the second video, maybe things get clearer

If you can make requests to the DB without logging in it's probably because you've allowed Any
one to access the collection in this case. Checkout appwrite's permissions https://appwrite.io/docs/products/databases/permissions

Steven do you have a link for the mobile app code repo?
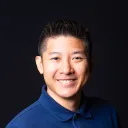
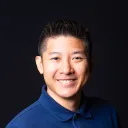
the SDK automatically handles sessions in the Client (including persisting it). Maybe that's what's going on?

Thanks. I was hoping to learn something from how you've handled jwt expiry when fetching images for a user
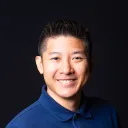
our photos are public so no need for JWT 😅

Yes, realised from the code. I'll think of a way when I get the time to work on my project. Still keeping an eye on the repo (honour of second star is all mine). Thanks anyways
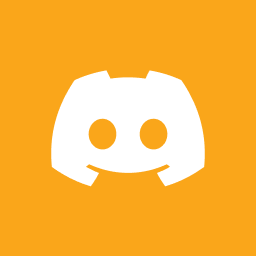
@Ernest I only allowed a specific user access. Thats why I am puzzeled

As Steven said above it's probably due to the session cache. To verify that it's the cache, you can clear the app's data (if you're developing on android) then try to access the db
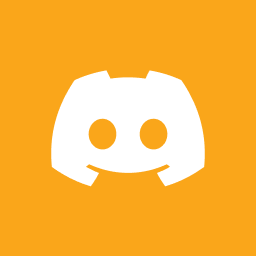
I'll try out. So far I used the Linux client. I will have another look.
Recommended threads
- App with randomly scheduled notification...
**Hey everyone! 👋** Building a Flutter app that sends users 1-3 location-based challenges per week based on their preferences. ## The flow: 1. User selects ho...
- Type generation without $id parameter
I'm trying for the first time appwrite on 2 projects and after I've tested appwrite types generation with the cli I'm a little bit confused why it doesn't inclu...
- Implementing Offline Login Caching with ...
Hi, I'm evaluating Appwrite for my Flutter app and need clarification on handling offline login sessions. My app requires users to log in, with the session ca...
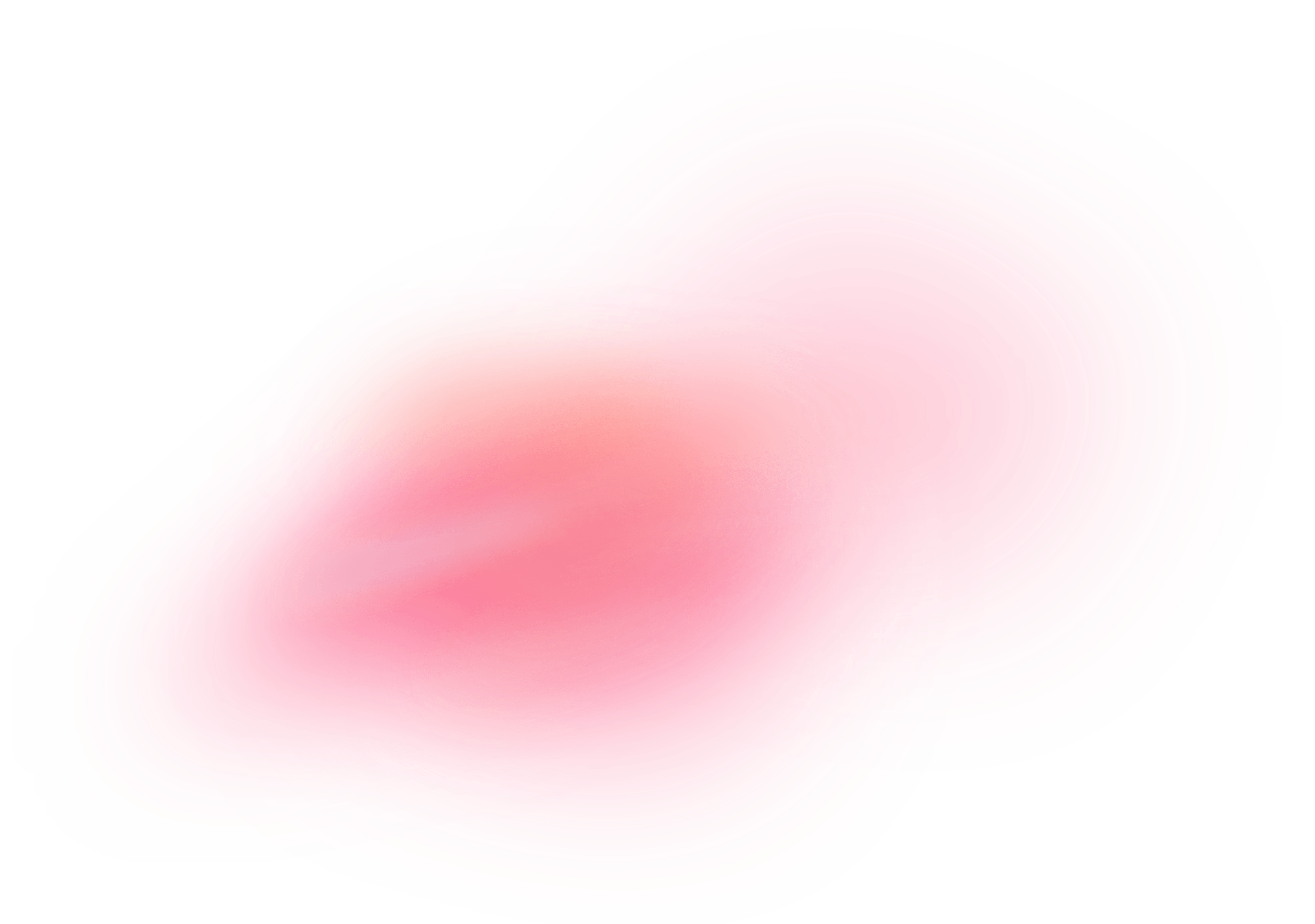