Learn how to setup your first SvelteKit project powered by Appwrite.
Create project
Head to the Appwrite Console.
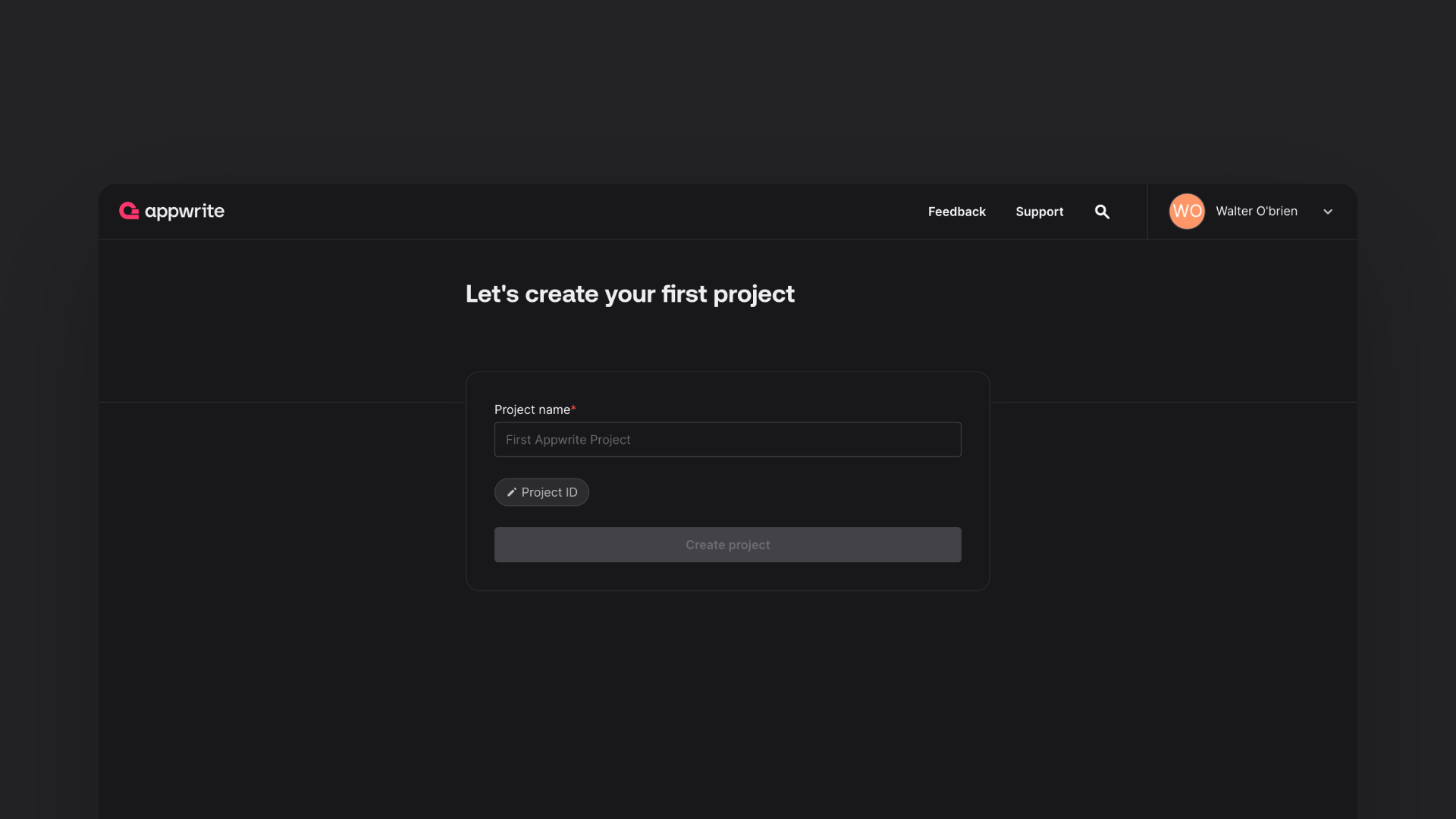
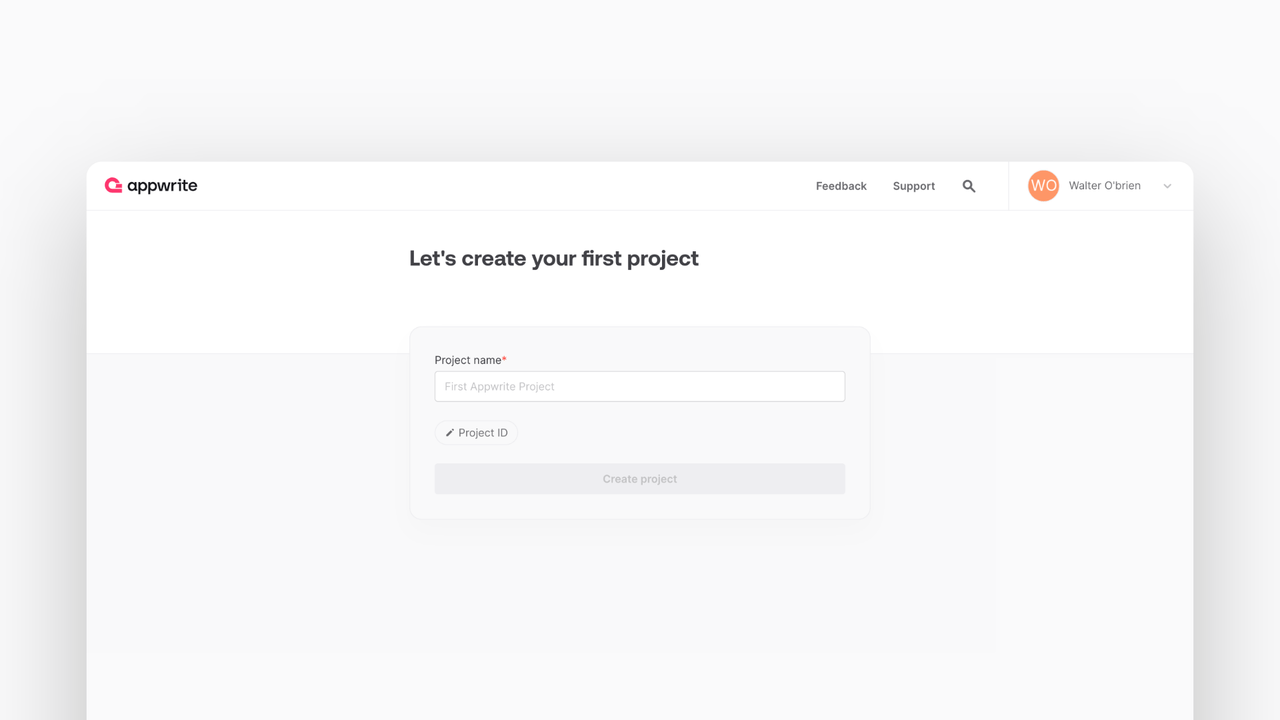
If this is your first time using Appwrite, create an account and create your first project.
Then, under Add a platform, add a Web app. The Hostname should be localhost.
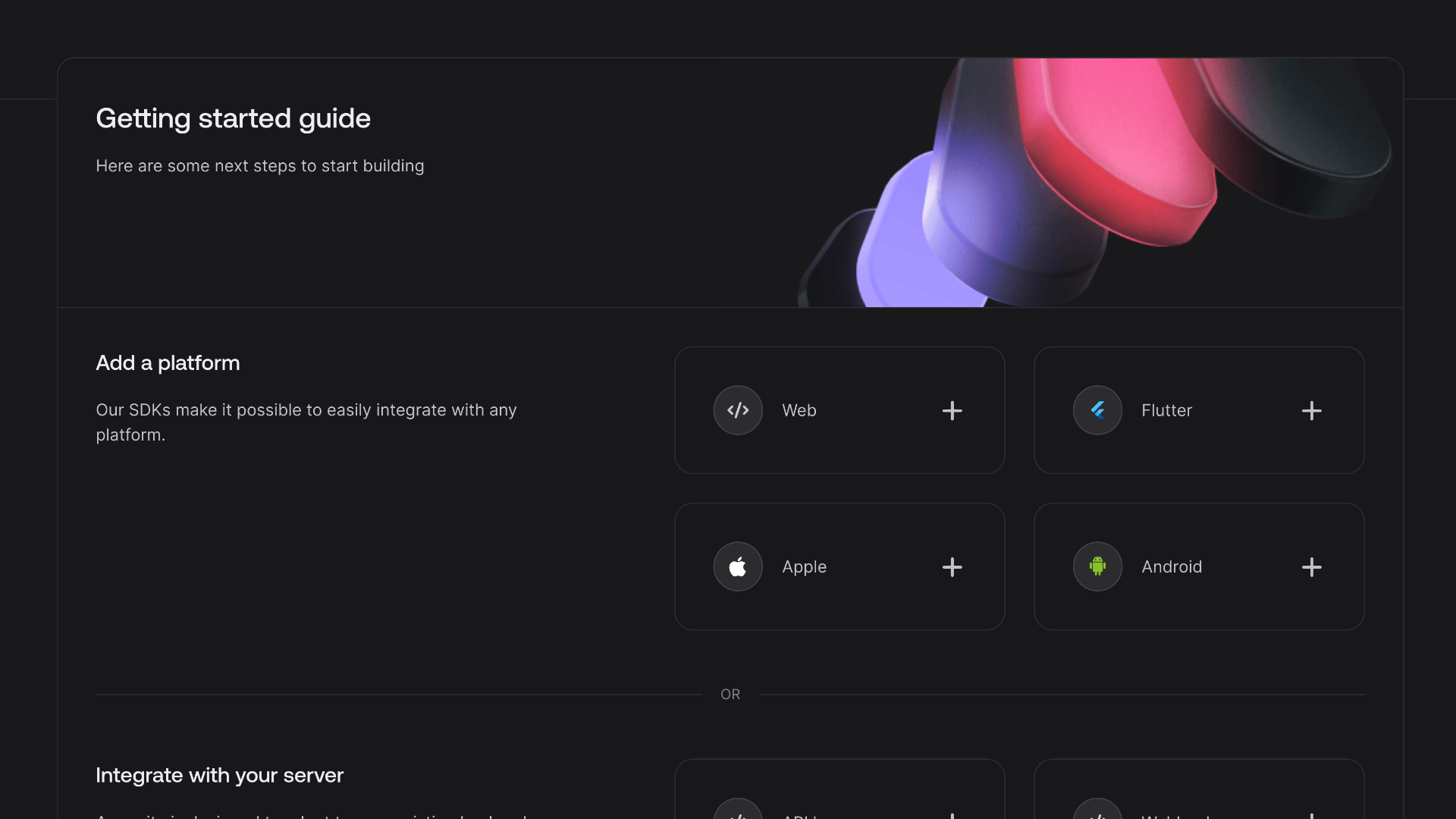
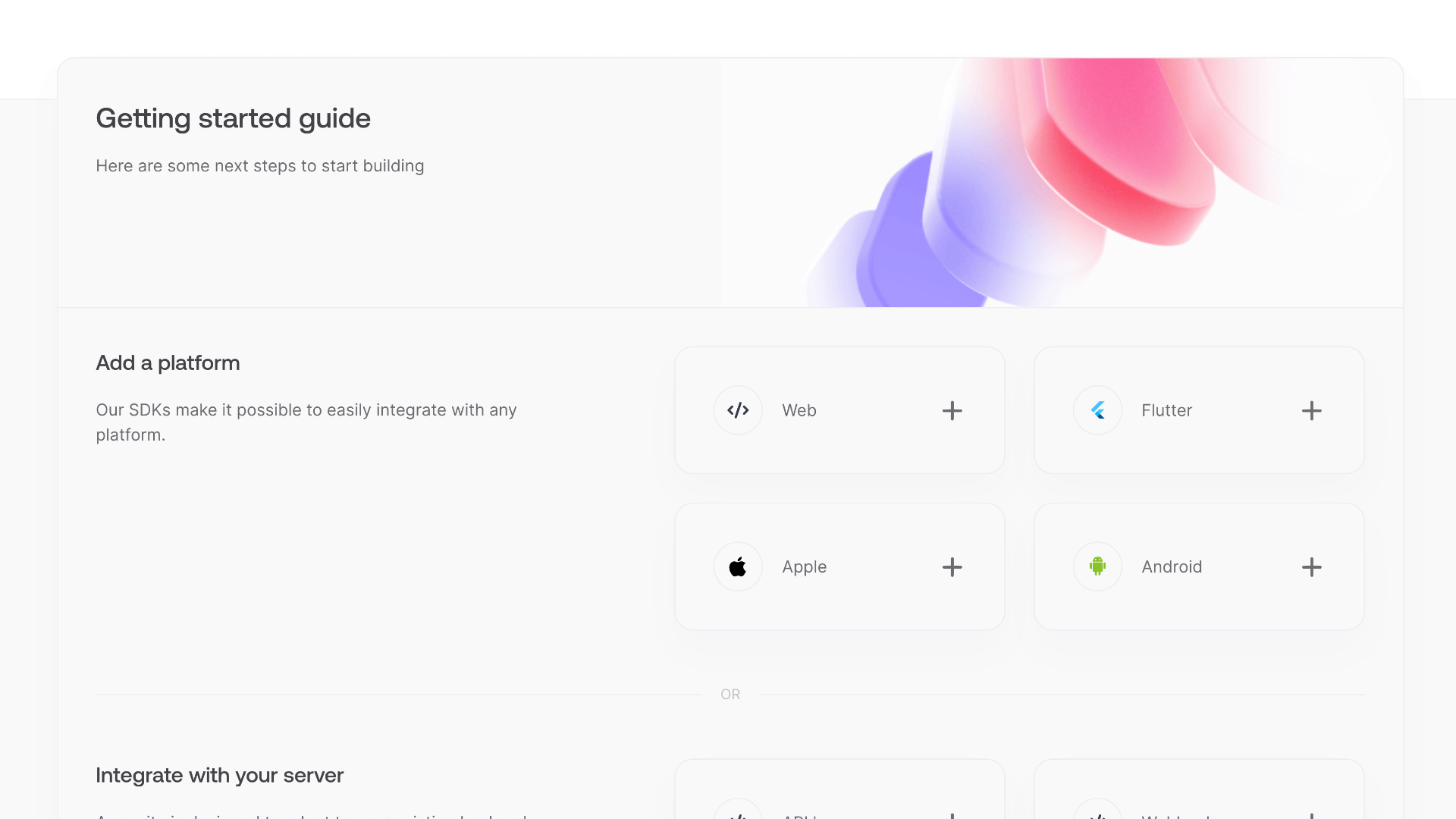
You can skip optional steps.
Create SvelteKit project
Create a SvelteKit project.
Shell
npx sv create
Install Appwrite
Install the JavaScript Appwrite SDK.
Shell
npm install appwrite@14.0.1
Import Appwrite
Find your project's ID in the Settings page.
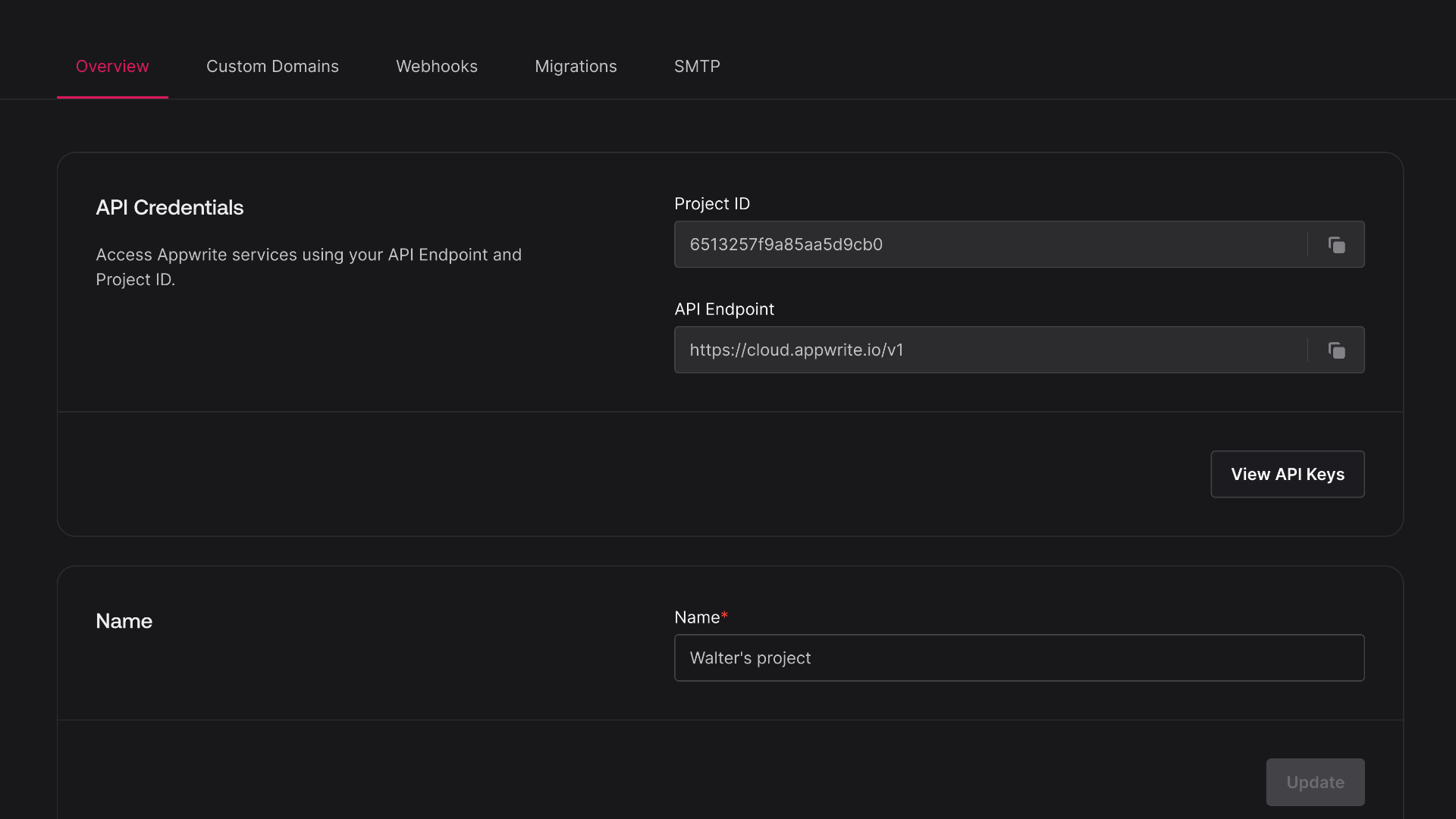
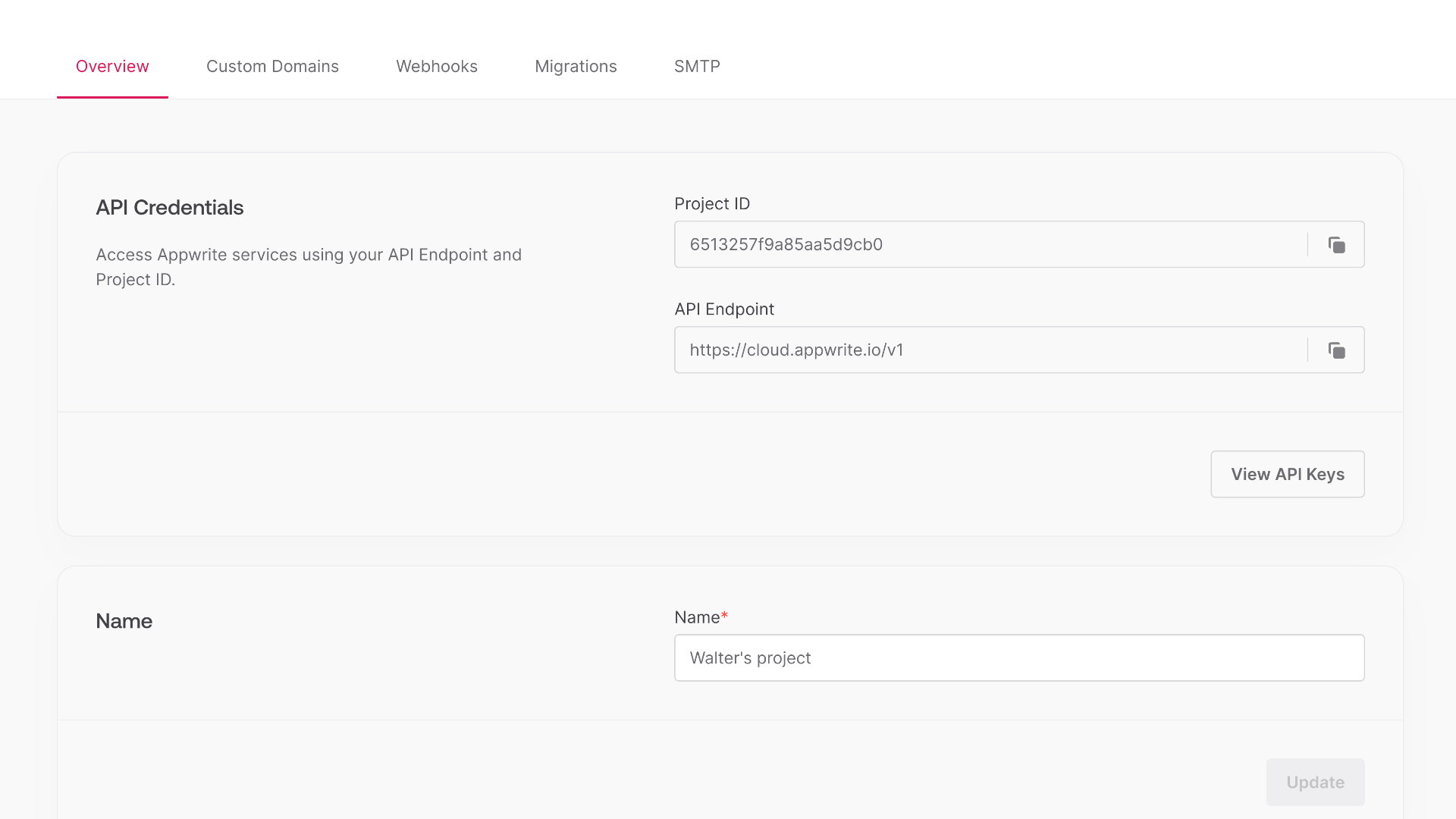
Create a new file src/lib/appwrite.js and add the following code to it, replace <PROJECT_ID> with your project ID.
Web
import { Client, Account } from 'appwrite';
export const client = new Client();
client
.setEndpoint('https://<REGION>.cloud.appwrite.io/v1')
.setProject('<PROJECT_ID>'); // Replace with your project ID
export const account = new Account(client);
export { ID } from 'appwrite';
Create a login page
Replace the contents of src/routes/+page.svelte with the following code.
HTML
<script>
import { account, ID } from '$lib/appwrite';
let loggedInUser = null;
async function login(email, password) {
await account.createEmailPasswordSession(email, password);
loggedInUser = await account.get();
}
async function register(email, password) {
await account.create(ID.unique(), email, password);
login(email, password);
}
function submit(e) {
e.preventDefault();
const formData = new FormData(e.target);
const type = e.submitter.dataset.type;
if (type === "login") {
login(formData.get('email'), formData.get('password'));
} else if (type === "register") {
register(formData.get('email'), formData.get('password'));
}
}
async function logout() {
await account.deleteSession('current');
loggedInUser = null;
}
</script>
<p>
{loggedInUser ? `Logged in as ${loggedInUser.name}` : 'Not logged in'}
</p>
<form on:submit={submit}>
<input type="email" placeholder="Email" name="email" required />
<input type="password" placeholder="Password" name="password" required />
<button type="submit" data-type="login">Login</button>
<button type="submit" data-type="register">Register</button>
</form>
<button on:click={logout}>Logout</button>
All set
Run your project with npm run dev and open localhost on port 5173 in your browser.