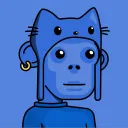
I am calling this function to handle login, i want to handle different scenarios for password less than 8 characters, invalid credentials, etc.
const handleLogin = async (e: FormEvent<HTMLFormElement>) => {
e.preventDefault();
try {
setUserLoading(true);
await account.createEmailSession(email, password);
setUserLoading(false);
router.push("/app");
} catch (error) {
console.log(error);
if (error instanceof AppwriteException) {
{/* @ts-ignore */}
alert(error.response.message)
}
}
};
So I am checking if the error is an instance of AppwriteException then I just want to alert the user the message. I can see this in the browser console e.g.
AppwriteException {
name: 'AppwriteException',
code: 401,
type: 'user_invalid_credentials',
response: {
message: 'Invalid credentials. Please check the email and password.',
code: 401,
type: 'user_invalid_credentials',
version: '0.10.28'
},
stack: 'AppwriteException: Invalid credentials. Please check the email and password.\n' +
' at Client.eval (webpack-internal:///(app-client)/./node_modules/appwrite/dist/esm/sdk.js:410:27)\n' +
' at Generator.next (<anonymous>)\n' +
' at fulfilled (webpack-internal:///(app-client)/./node_modules/appwrite/dist/esm/sdk.js:41:58)',
message: 'Invalid credentials. Please check the email and password.'
}
Just accessing error.response.message
but getting this error Property 'message' does not exist on type 'string'.
web sdk version: 11.0.0
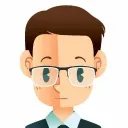
Even though it looks as an object response
its actually a string.
So try something like this
} catch (error) {
console.log(error);
if (error instanceof AppwriteException) {
const response = JSON.parse(error.response ?? '{}') ?? {};
alert(response.message);
}
}
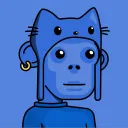
Yes it is declared as a string in client.d.ts
declare class AppwriteException extends Error {
code: number;
response: string;
type: string;
constructor(message: string, code?: number, type?: string, response?: string);
}
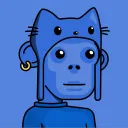
thinking of doing something like this
} catch (error) {
console.log(error);
if (error instanceof AppwriteException) {
const response = JSON.parse(error.response ?? '{}') ?? {};
const errorMessage = response.message || 'An unknown error occurred.';
alert(errorMessage);
}
}
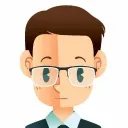
It worked for you that way?
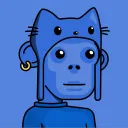
nope, it does not work
Unhandled Runtime Error
SyntaxError: "[object Object]" is not valid JSON
because i think response here is an object
if (error instanceof AppwriteException) {
{/* @ts-ignore */}
alert(error.response.message)
}
this works but i have to use @ts-ignore
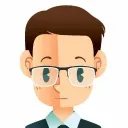
Ohh, gotcha make sense - the structure, because the problem is that the type is string.
If you want to avoid the @ts-ignore
you can cast it
const response = (error.response as any);
alert(response.message);
Recommended threads
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- delete document problems
i don't know what's going on but i get an attribute "tournamentid" not found in the collection when i try to delet the document... but this is just the document...
- Attributes Confusion
```import 'package:appwrite/models.dart'; class OrdersModel { String id, email, name, phone, status, user_id, address; int discount, total, created_at; L...
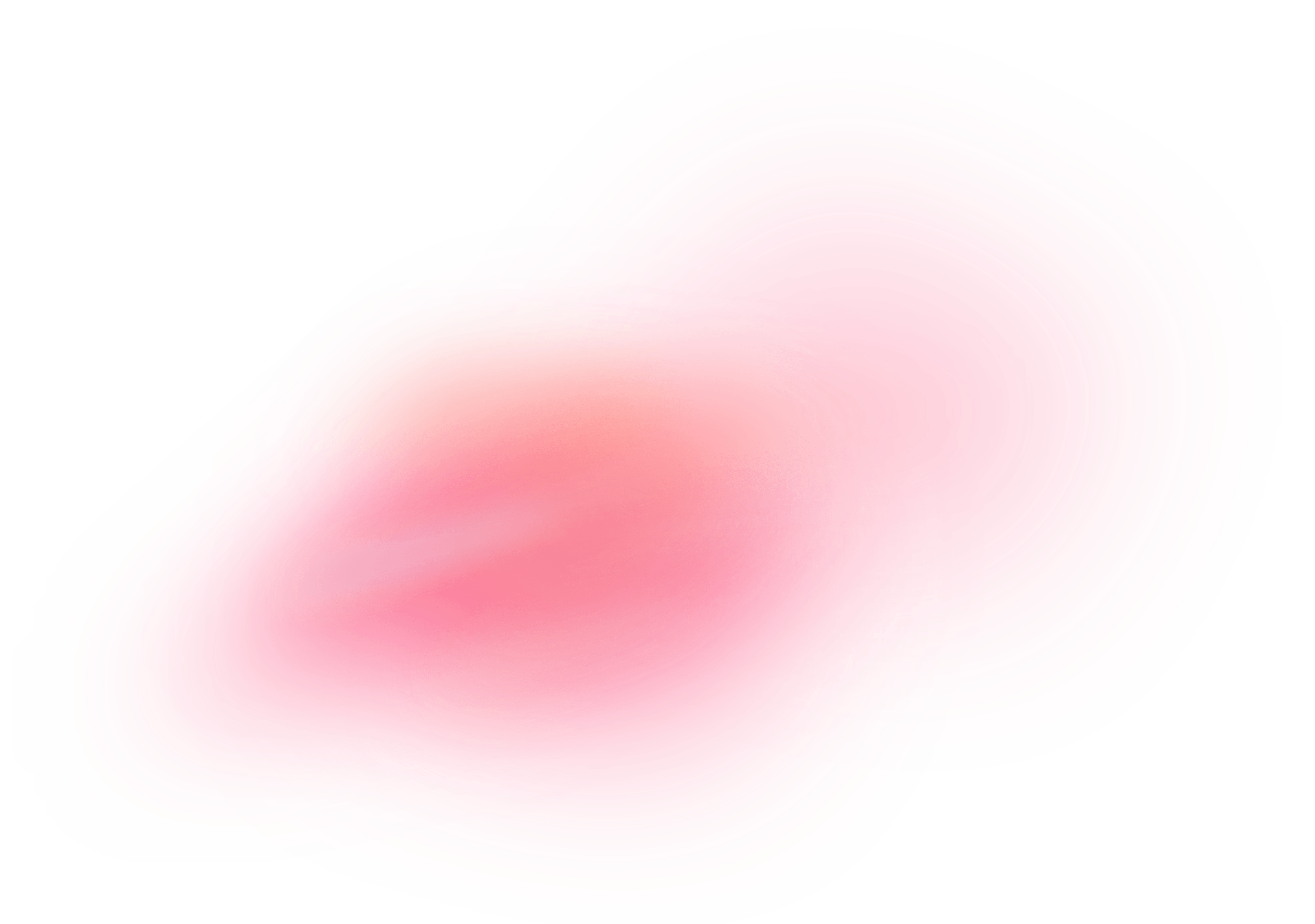