
package vasu.apps.schooldashboard.Services
import android.util.Log import io.appwrite.Client import io.appwrite.ID import io.appwrite.exceptions.AppwriteException import io.appwrite.models.User import io.appwrite.services.Account
class AccountService(client: Client) { private val account = Account(client)
suspend fun getLoggedIn(): User<Map<String, Any>>? {
return try {
account.get()
} catch (e: AppwriteException) {
null
}
}
suspend fun login(email: String, password: String): User<Map<String, Any>>? {
return try {
account.createEmailPasswordSession(email, password)
getLoggedIn()
} catch (e: AppwriteException) {
null
}
}
suspend fun register(
email: String,
password: String,
name: String,
phoneNumber: String, // e.g., "9876543210"
label: String // Custom label to be stored with phone number
): User<Map<String, Any>>? {
return try {
val sanitizedPhoneNumber = phoneNumber.filter { it.isDigit() }
val fullPhoneNumber = "+91$sanitizedPhoneNumber"
if (fullPhoneNumber.length > 15) {
Log.e("AccountService", "Phone number exceeds 15 character limit: $fullPhoneNumber")
return null
}
account.create(ID.unique(), email, password, name)
val user = login(email, password)
user?.let {
account.updatePhone(user.id, fullPhoneNumber)
}
user
} catch (e: AppwriteException) {
Log.e("AccountService", e.message.toString())
} as User<Map<String, Any>>?
}
suspend fun logout() {
account.deleteSession("current")
}
}
in this i get error like this : E Invalid phone param: Phone number must start with a '+' can have a maximum of fifteen digits.
and i input the number like this : +911234567890
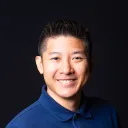
FYI, it's best to wrap code in backticks to format a bit nicer. You can use 1 backtick for inline code (https://www.markdownguide.org/basic-syntax/#code) and 3 backticks for multiline code (https://www.markdownguide.org/extended-syntax/#syntax-highlighting).
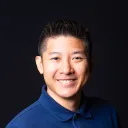
account.updatePhone(user.id, fullPhoneNumber)
What told you to pass user id and phone number as the params?
Recommended threads
- Email Verification Email
Hi everyone, I’m currently experiencing an issue with the email verification functionality. When I trigger the verification, the request returns a valid respon...
- Persistent 401 Unauthorized on all authe...
Hello, I'm facing a critical 401 Unauthorized error on my admin panel app and have exhausted all debugging options. The Problem: When my React app on localhos...
- Google Oauth help
this error pops up (TypeError: (0 , {imported module [project]/src/lib/auth.ts [app-rsc] (ecmascript)}.signIn) is not a function) but cant find a way to solve i...
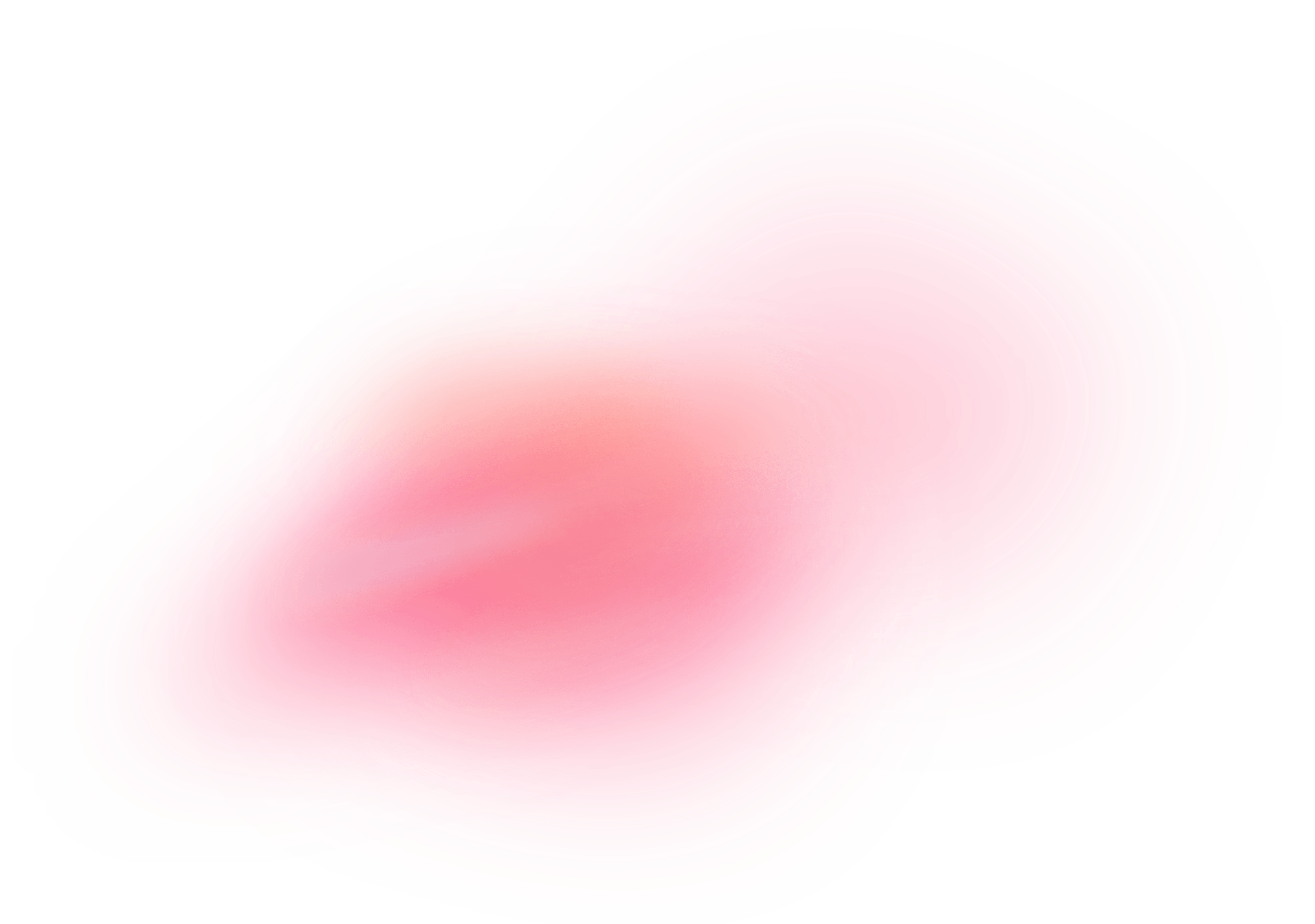