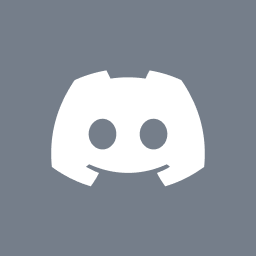
I created an app and am trying to upload PDF into appwrite storage bucket and fetch it back but currently my issue is in uploading the PDF to appwrite storage bucket here is the upload function const handleUpload = async () => { if (!validateFields() || !pdf) { setErrors((prevErrors) => ({ ...prevErrors, pdf: 'Please select a PDF file.' })); return; }
try { const fileId = pdfMetadata ? pdfMetadata.fileId : 'unique()';
// Fetch and convert the file to a Blob
const response = await fetch(pdf.uri);
const fileBlob = await response.blob();
// Upload the file
const uploadResponse = await storage.createFile('pdfs_bucket', fileId, fileBlob);
// Generate a public URL for the uploaded file
const publicURL = storage.getFilePreview('pdfs_bucket', uploadResponse.$id);
if (pdfMetadata) {
// Update existing document
await databases.updateDocument('Coou_App', 'Pdf_metadata', pdfMetadata.$id, {
faculty,
department,
level,
semester,
course,
pdf_url: publicURL.href,
});
Alert.alert('Success', 'PDF Updated successfully!');
} else {
// Create a new document
await databases.createDocument('Coou_App', 'Pdf_metadata', 'unique()', {
faculty,
department,
level,
semester,
course,
pdf_url: publicURL.href,
});
Alert.alert('Success', 'PDF Uploaded successfully!');
}
// Reset state
setPdfMetadata({});
setActionButtonText('Update PDF');
} catch (err) { setErrors((prevErrors) => ({ ...prevErrors, upload: err.message || 'Failed to upload PDF.', })); } };
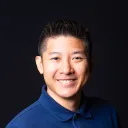
FYI, it's best to wrap code in backticks to format a bit nicer. You can use 1 backtick for inline code (https://www.markdownguide.org/basic-syntax/#code) and 3 backticks for multiline code (https://www.markdownguide.org/extended-syntax/#syntax-highlighting).
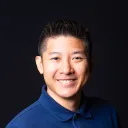
are you using the Appwrite react native SDK?
Recommended threads
- issue authenticating new user : [Appwrit...
i am currently facing an issue that didn't appear except few days back now, and so far i've tried and can't fix it i created a platform integration using my app...
- I am getting a 401 unauthorized response...
I have a Next.js application that stores user PDFs. I'm able to save them normally, but when trying to access the files using getFileView, I get a 401 Unauthori...
- Google OAuth2 screen loop issue in Flut...
i am trying to authenticate user on my app using google, everything works fine, but even after succesfull authentication instead of redirecting back to the app,...
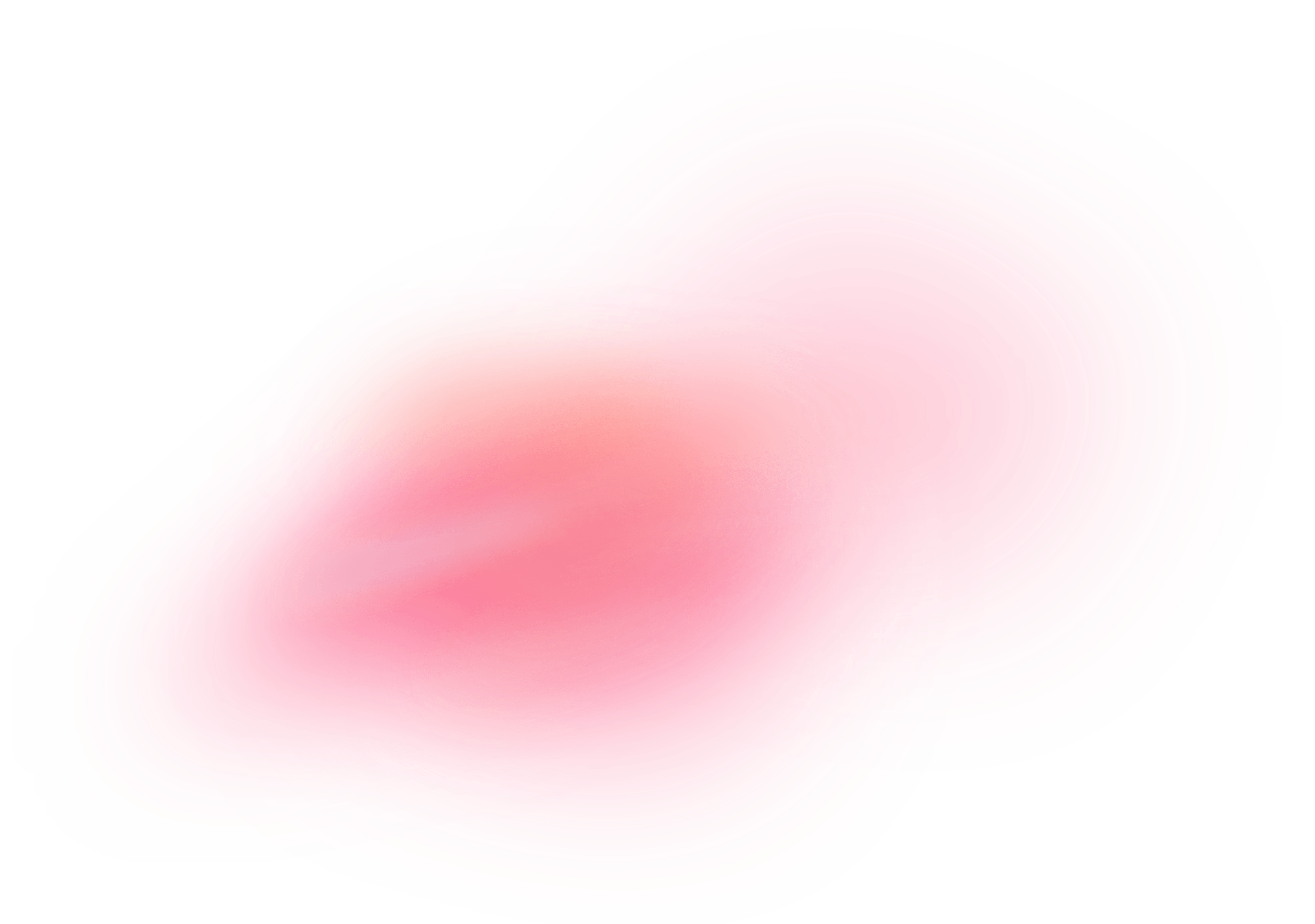