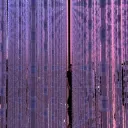
Hello everyone. I'm creating my first Node.JS function, but I don't have much experience with node and javascript.
I'm trying to create a function, that monitor events (update/create) around a specific collection. Every time a specific field of every document is updated or created in this collection, I need to update other fields.
However, I am experiencing difficulties in capturing events. I have configured the function on AppWrite Cloud, and when an event of these happens, my function is called, but then I haven't figured out how to capture the event inside node.js.
Also it seems there is no documentation on AppWrite docs about how to monitor events, so every help is much appreciated!
cc @Kenny
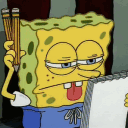
Hey! So to grab the event data you can do something like this
import { Client, Databases } from 'node-appwrite';
export default async ({ req, res, log, error }) => {
// The event should send the payload in the body
const body = req.bodyJson;
const id = body['id'];
// To get the information you want out of this you could do something like
const attributeData = body['attribute'];
// Do whatever calculations you need to set the new attributes derived value.
const newAttributeData = attributeData + 1;
// Setup your client
const client = new Client()
.setEndpoint(process.env.APPWRITE_FUNCTION_API_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(req.headers['x-appwrite-key'] ?? '');
const database = new Databases(client);
try {
// Update the derived attribute.
const response = await database.updateDocument(DATABASE_ID, COLLECTION_ID, id, {
newAttribute: newAttributeData
});
} catch(err) {
// Log any errors.
error(err.message);
}
// Return
return res.empty();
};
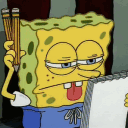
I didn't create a function and test this out, but this should be the logic you need to accomplish what you want
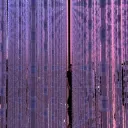
Thanks, Kenny! Let me test it π
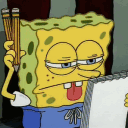
Here is the appropriate documentation https://appwrite.io/docs/products/functions/develop#request https://appwrite.io/docs/advanced/platform/events
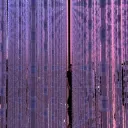
it works! Amazing
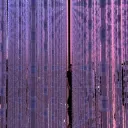
Thanks, Kenny π kudos to you!
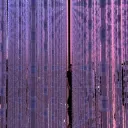
I was using a completely wrong approach
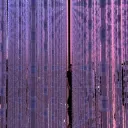
As far as you know, is it possible to trigger an event, if just specific attributes are updated, instead of the whole document @Kenny ?
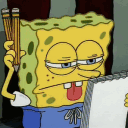
I donβt believe so :(
Recommended threads
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
- Connecting server functions to GitHub re...
The project I am working in has recently moved organizations on Appwrite. The same is true for the repo on GitHub, which as moved from a private user to a organ...
- Missing C++ libstdc library in Python fu...
I have a function running Python 3.12 which suddenly started dumping errors (as of today; it worked yesterday). I hadn't changed any code so I found this odd, b...
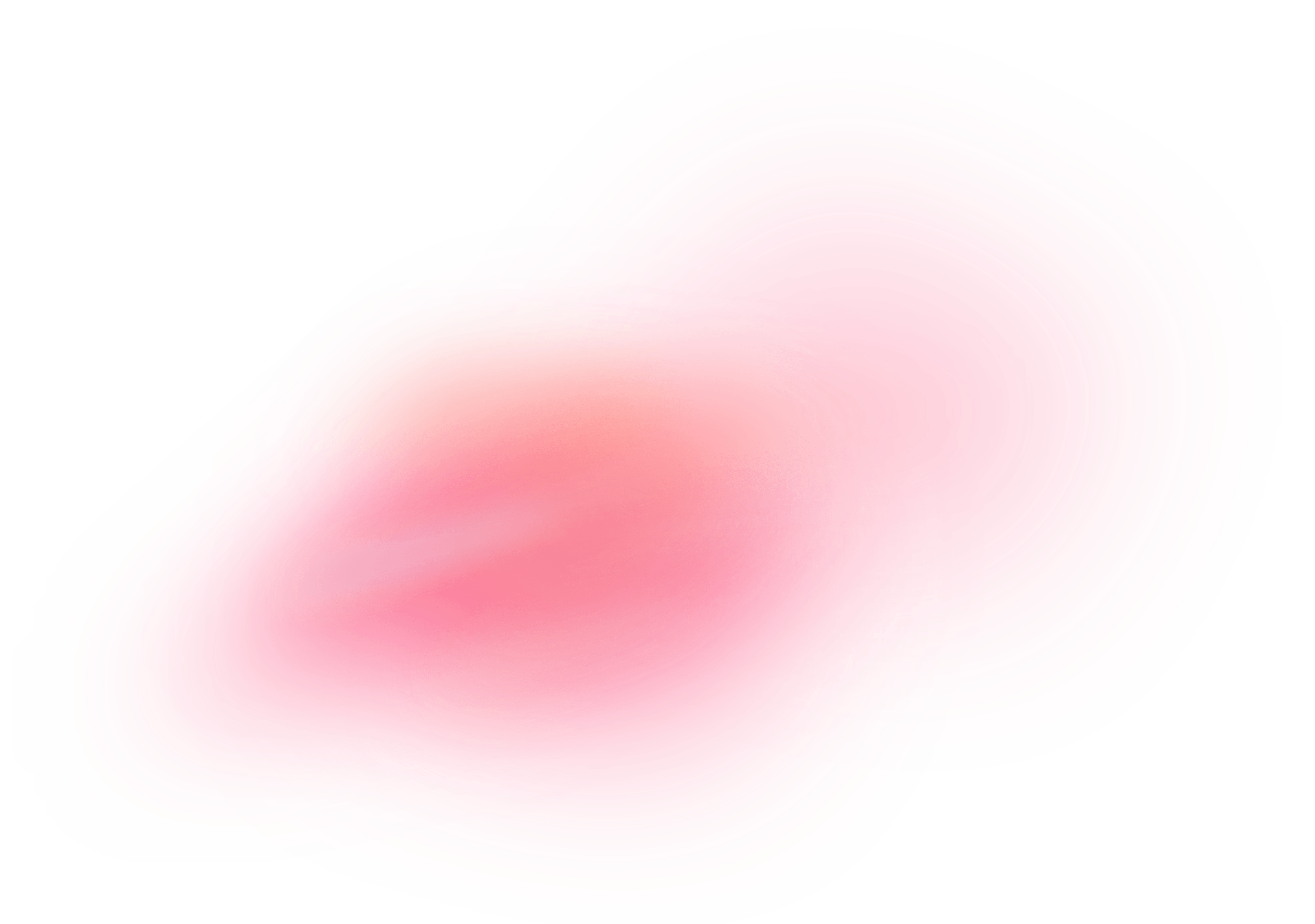