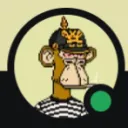
Hey guys, I have read the documentation and must be missing something. I am trying to zip a few images and upload the zip to appwrite. What am I doing wrong here?
I am in react-native. It appears react-native-appwrite SDK doesn't have the InputFile
class?
// Function to zip images and upload to Appwrite
const zipAndUploadImages = async (selectedImages) => {
console.log("Attempting to zip and upload selected images...");
try {
const zip = new JSZip();
for (let i = 0; i < selectedImages.length; i++) {
console.log(`Fetching image: ${selectedImages[i]}`);
const response = await fetch(selectedImages[i]);
const arrayBuffer = await response.arrayBuffer();
console.log(`Adding image_${i + 1}.jpg to zip`);
zip.file(`image_${i + 1}.jpg`, arrayBuffer);
}
console.log('Creating zip file...');
// Generate zip as Base64
const zipBase64 = await zip.generateAsync({ type: 'base64' });
console.log('Uploading zip file to Appwrite collection...');
const buffer = Uint8Array.from(atob(zipBase64), (char) => char.charCodeAt(0)); // Convert Base64 to Uint8Array
try{
const file = await storage.createFile(
config.appwriteFilesId, // Replace with your Appwrite bucket ID
ID.unique(),
buffer
);
console.log('File uploaded successfully!');
return file.$id;
}
catch (error){
console.error('Error storing zip in appwrite.', error)
return null;
}
} catch (error) {
console.error('Error zipping or uploading images:', error);
return null;
}
};
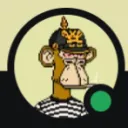
Focus on this part
const file = await storage.createFile(
config.appwriteFilesId, // Replace with your Appwrite bucket ID
ID.unique(),
buffer
Recommended threads
- "Invalid relationship value. Must be eit...
In my app i am trying to update the documents in my databse from a form in my app, and i am getting this error Error updating event users: AppwriteException: I...
- Profile Image from storage not showing (...
I am uploading a photo during register, and it is also being uploaded to appwrite storage . But in my profile screen it is showing black screen. I am attaching ...
- reoccurring resource limit usage
Two days ago I had of the error (attached) when there’s virtually no usage this month for any of my appwrite apps Yesterday we cleared up and I wasn’t getting...
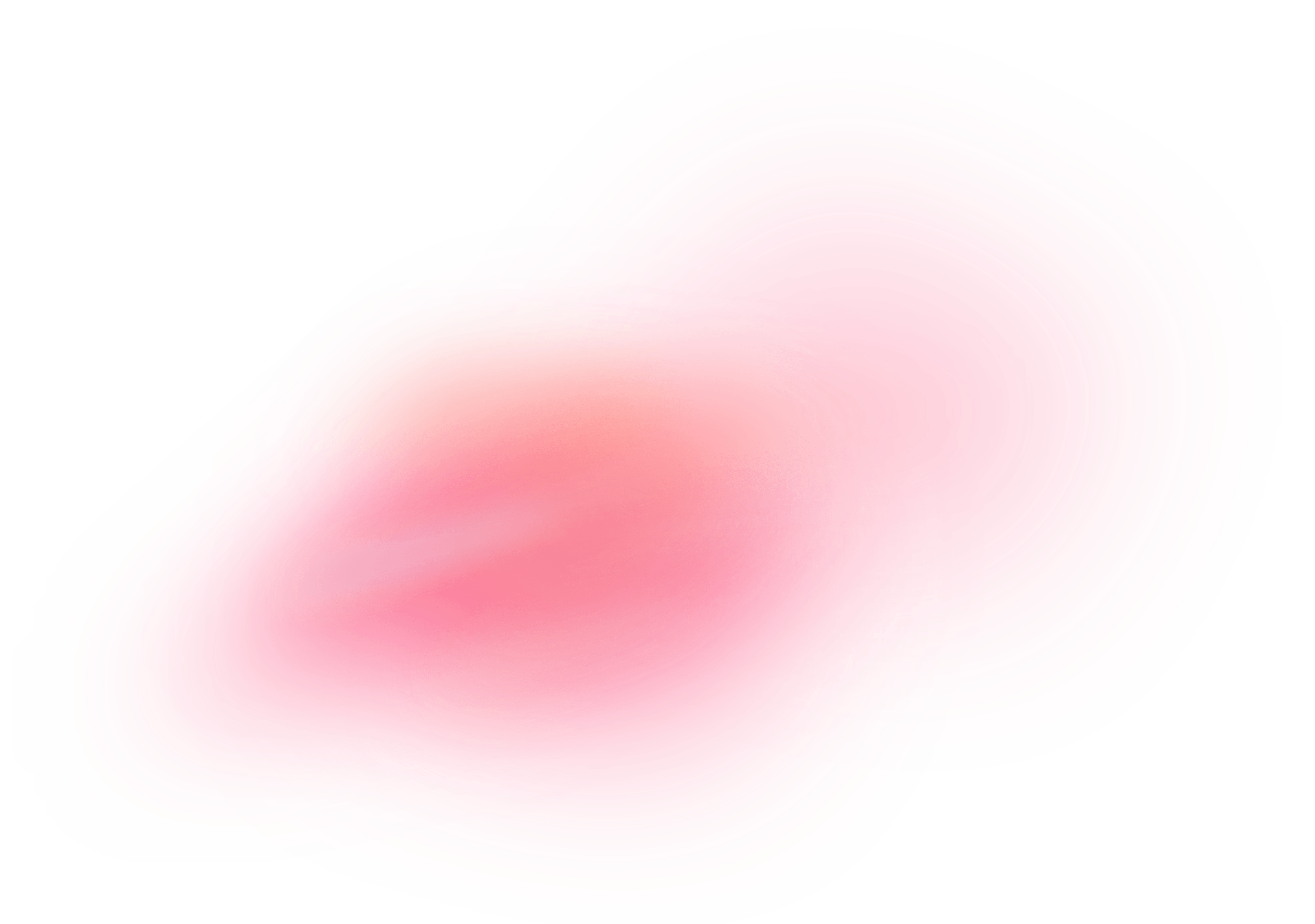