
Next.js:
- After logging in, we get a cookie with the appwrite session name, as expected. This is detected here, since we get a cookie matching the session name.
- We are able to get the { account } and I have logged that session was found. See code snippet below.
- The AppwriteException is thrown at
await account.deleteSession("current")
. Even though we have fetched an account, we have a seession, and that is the same session that is created during login. See login snippet below as well. - What the fudge is this behavior? Is this not the correct way to delete a session server side?
TypeScript
"use server";
import { createSessionClient } from "@/adapters/infrastructure/appwrite/server/appwrite-server-config";
import { cookies } from "next/headers";
import env from "@/config/env";
export const logoutAction = async () => {
const sessionCookie = cookies().get(env.appwriteSessionName);
if (!sessionCookie) {
return {
error: "Ingen session funnet",
};
}
try {
const { account } = await createSessionClient(sessionCookie);
await account.deleteSession("current");
cookies().delete(env.appwriteSessionName);
return {
success: true,
};
} catch (error) {
console.error("Logout Error: ", error);
return {
error: "Noe gikk galt, kunne ikke logge deg ut",
};
}
};
This is how we create the client session client, which confirms we found a session that we set:
TypeScript
export const createSessionClient = async (session: any) => {
const client = new Client()
.setEndpoint(env.publicAppwriteEndpoint)
.setProject(env.publicAppwriteProject);
if (session) {
console.log("Session Found!")
client.setSession(session);
} else {
console.log("Session NOT Found: Could not set it!")
}
return {
get account() {
return new Account(client);
},
get users() {
return new Users(client);
},
get databases() {
return new Databases(client);
},
};
};
TL;DR
Issue: AppwriteException is thrown when attempting to delete a session, even though the session was successfully found and created during login.
Solution: The developers need to ensure that the authenticated user has the necessary scope to delete a session. Verify the role permissions and scopes for the 'guests' role in Appwrite to include the required permissions to delete a session.
Login Action:
TypeScript
"use server";
import { z } from "zod";
import {
LoginUserSchema,
validateLoginUser,
} from "@/src/core/application/auth/schemas/login-user-schema";
import { ValidationError } from "@/src/core/adapters/infrastructure/errors/validation-error";
import { createAdminClient } from "@/adapters/infrastructure/appwrite/server/appwrite-server-config";
import { cookies } from "next/headers";
import env from "@/config/env";
type LoginActionDto = z.infer<typeof LoginUserSchema>;
export const loginAction = async (loginActionDto: LoginActionDto) => {
try {
validateLoginUser(loginActionDto);
const { email, password } = loginActionDto;
try {
const { account } = await createAdminClient();
const session = await account.createEmailPasswordSession(email, password);
cookies().set(env.appwriteSessionName, session.secret, {
httpOnly: true,
secure: true,
sameSite: "strict",
expires: new Date(session.expire),
path: "/",
});
return {
success: true,
};
} catch (error) {
console.log("Authentication Error: ", error);
return {
error: "Brukernavn eller passord er feil",
};
}
} catch (error) {
if (error instanceof ValidationError) {
throw error;
}
console.error("Unhandled Error occurred during registration flow:", error);
throw new ValidationError({
error: "Something unexpected went during registration",
});
}
};
Recommended threads
- Appwrite stopped working, I can't authen...
I'm having an issue with Appwrite. It was working fine just a while ago, but suddenly it stopped working for me and can't authenticate accounts. I even went bac...
- Set succes/failure url in console
Hi guys, I want to set up a succes and failure url for my OAuth2 provider Google. But I don't see any options for this? Is it not possible to do so? Beside th...
- Fail to receive the verification email a...
I added my email address to prevent it from showing "appwrite," but now I'm not receiving emails for verification or password resets. The function appears to be...
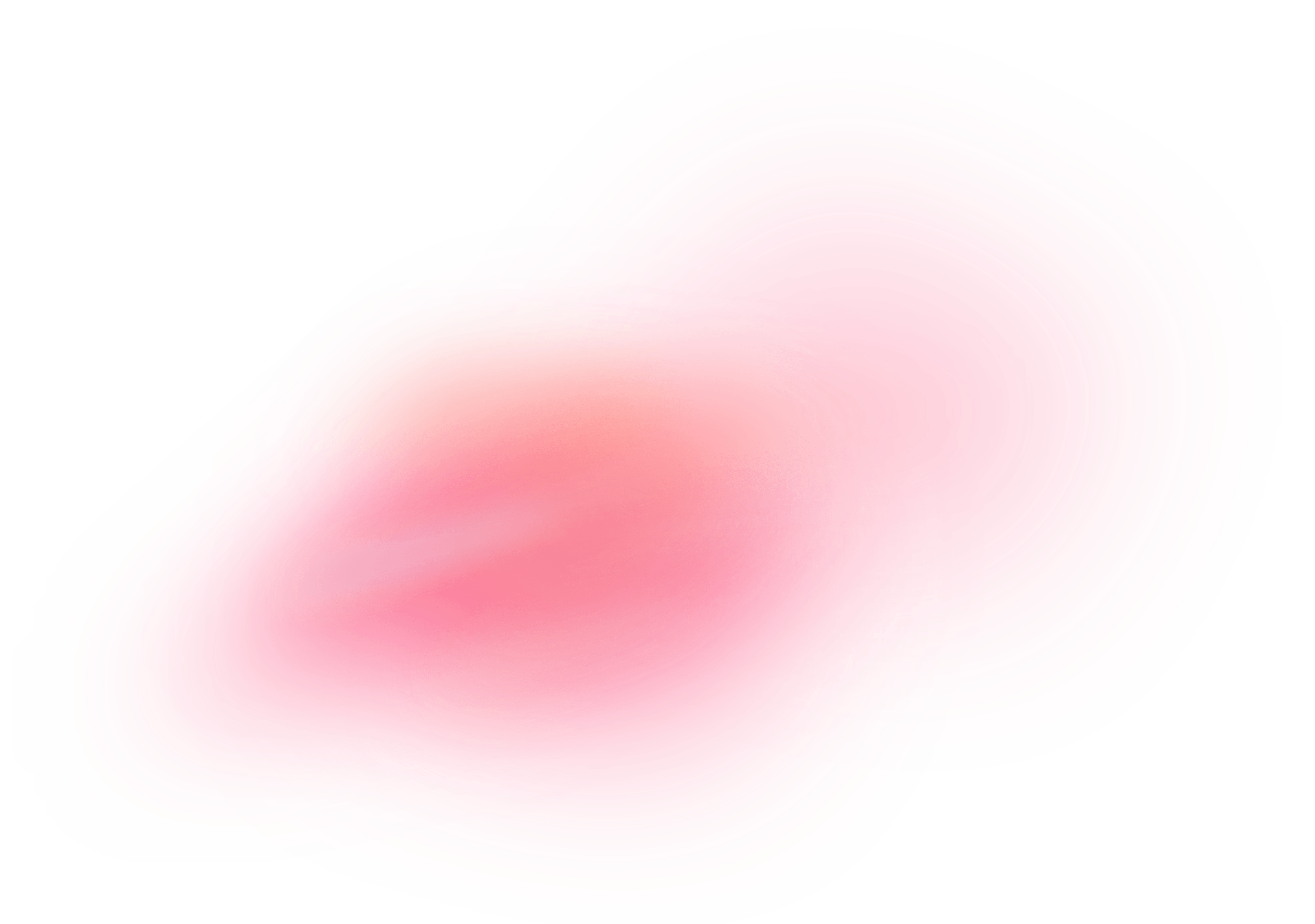