
I want to check if the user has a valid session before fetching data
import { Client, Users } from 'node-appwrite';
export async function GET() {
const client = new Client()
.setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT as string)
.setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT_ID as string)
.setKey(process.env.APPWRITE_API_KEY as string); // Securely use API key
const usersAPI = new Users(client);
try {
// Fetch the user list from Appwrite
const usersList = await usersAPI.list();
// Log the labels for each user
usersList.users.forEach((user) => {
console.log(`User: ${user.name}, Labels: `, user.labels);
});
const users = usersList.users.map((user) => ({
name: user.name || 'N/A',
email: user.email,
phone: user.phone || 'N/A',
emailVerification: user.emailVerification,
phoneVerification: user.phoneVerification,
isAdmin: user.labels.includes('admin') // Check if 'admin' label exists in labels array
}));
return NextResponse.json({ totalUsers: usersList.total, users });
} catch (error: any) {
console.error('Error fetching users:', error);
return NextResponse.json({ totalUsers: 0, users: [] }, { status: 500 });
}
}
I am trying to make some middleware that validates a users session and checkf for some custom permissions or the admin label before allowing them to proceed. Using app router in NextJs.
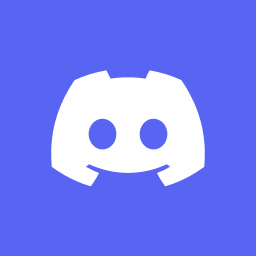
If you're using API key, then you have full access to everything
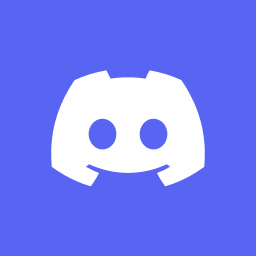
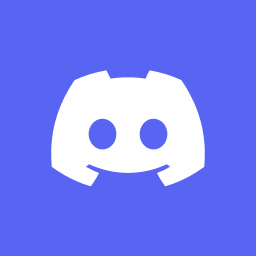
I think you should use .setSession in your case in order to work as the user instead of full admin server side
Recommended threads
- [CLOSED] 401 Access to this resource is ...
was adding a large number of documents 100k + via the api and creating documents started getting the error above and also can access the db on the cloud dashboa...
- Document Update Error: Invalid document ...
Trying to update the document but getting this error. Using appwrite cloud
- mime type for docx too long?
Hello, I am trying to upload a docx file to storage, but the mime type is too long. I get the following error: ``'Invalid document structure: Attribute "mimeTy...
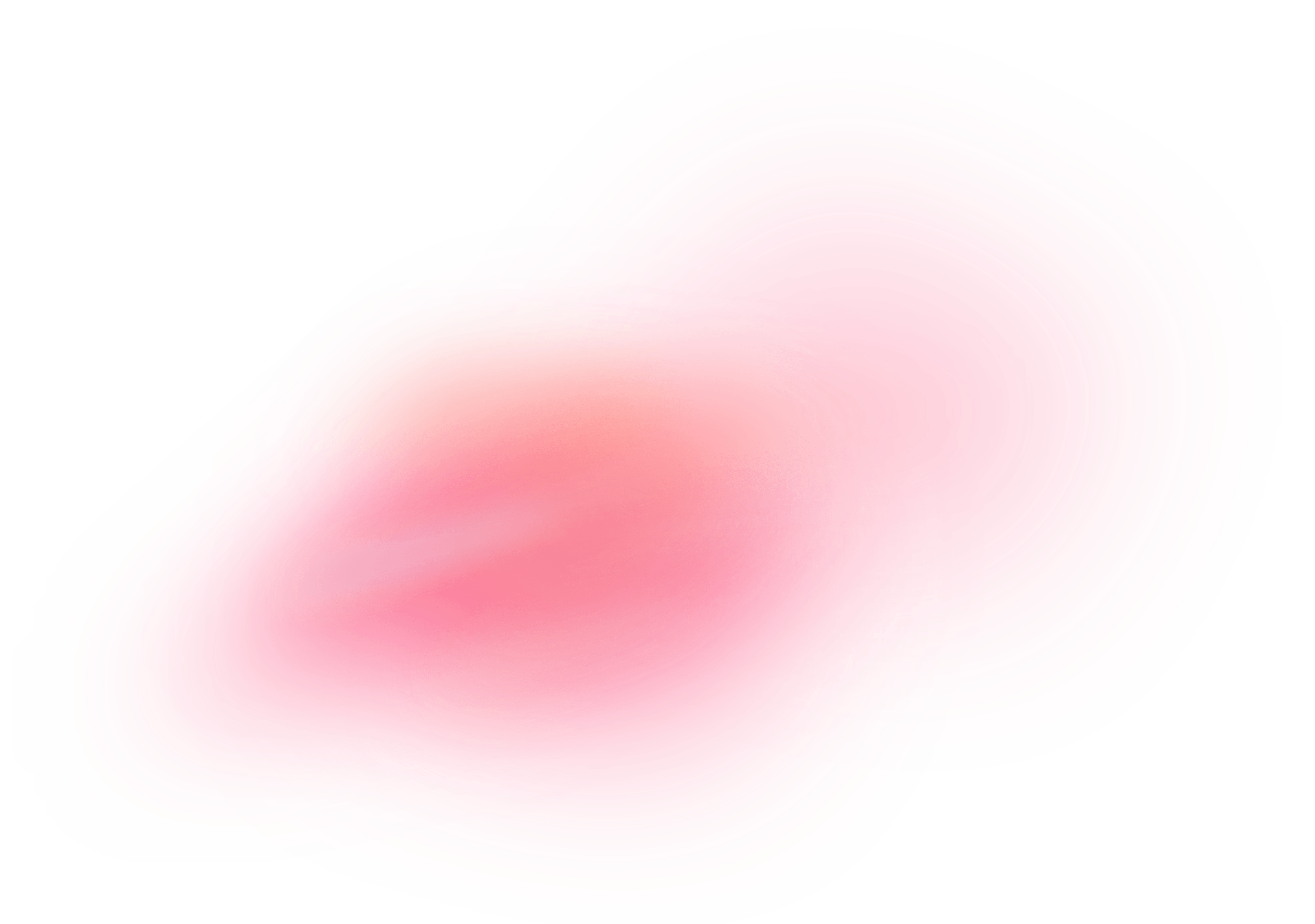