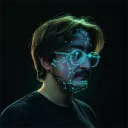
I'm encountering a persistent "Database not found" error in my Next.js application that uses Appwrite as the backend. Despite following the documentation and checking my configuration, I'm unable to resolve this issue. Below are the details: Environment:
Next.js (Version: [Your Next.js version]) Appwrite SDK (Version: [Your Appwrite SDK version]) Node.js (Version: [Your Node.js version])
Error Message: CopyAppwriteException: Database not found at _Client.call (webpack-internal:///(rsc)/./node_modules/node-appwrite/dist/client.mjs:283:13) ... code: 404, type: 'database_not_found', response: { message: 'Database not found', code: 404, type: 'database_not_found', version: '1.5.8' } Context: I'm trying to create a user document in my Appwrite database when a new user is created through a third-party authentication service (Dynamic). The error occurs when trying to execute the createDocument method of the Appwrite SDK.
Code Structure:
I have an appwrite.ts file that sets up the Appwrite client:
import { Client, Account, Databases, Users } from "node-appwrite";
export async function createAdminClient() { const client = new Client() .setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT!) .setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT!) .setKey(process.env.NEXT_APPWRITE_KEY!);
return { database: new Databases(client), }; }
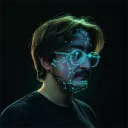
In my dynamic.user.actions.ts file, I have a createUser function:
const { APPWRITE_DATABASE_ID: DATABASE_ID, APPWRITE_USERS_COLLECTION_ID: USERS_COLLECTION_ID, APPWRITE_WALLETS_COLLECTION_ID: WALLETS_COLLECTION_ID, APPWRITE_VERIFIED_CREDENTIALS_COLLECTION_ID: VERIFIED_CREDENTIALS_COLLECTION_ID, } = process.env;
export const createUser = async (userData: CreateUserProps) => { try { const { database } = await createAdminClient(); console.log("New user data", userData);
const newUser = await database.createDocument(
DATABASE_ID,
USERS_COLLECTION_ID,
userData.id,
{
userId: userData.id,
email: userData.email,
// ... other user fields ...
}
);
// ... code to create related documents ...
return parseStringify(newUser);
} catch (error) { console.error("An error occurred while creating the user:", error); throw error; } };
Steps to Reproduce:
A new user signs up through the Dynamic authentication service. The webhook is triggered, calling my createUser function. The function attempts to create a new document in the Appwrite database. The "Database not found" error is thrown.
What I've Tried:
Triple-checked that the DATABASE_ID matches an existing database in my Appwrite project, as well with other env variables. Verified that the API key has the necessary permissions to access and modify the database. Verified permissions in each collection are set to All for all CRUD options. Tried hardcoding the database and collection IDs instead of using environment variables. Logged the database ID and other relevant information before the createDocument call and all variables are correctly passed.
Recommended threads
- Type Mismatch in AppwriteException
There is a discrepancy in the TypeScript type definitions for AppwriteException. The response property is defined as a string in the type definitions, but in pr...
- What Query's are valid for GetDocument?
Documentation shows that Queries are valid here, but doesn't explain which queries are valid. At first I presumed this to be a bug, but before creating a githu...
- Appwrite exception: user_unauthorized, t...
After refreshing the app it is working perfectly
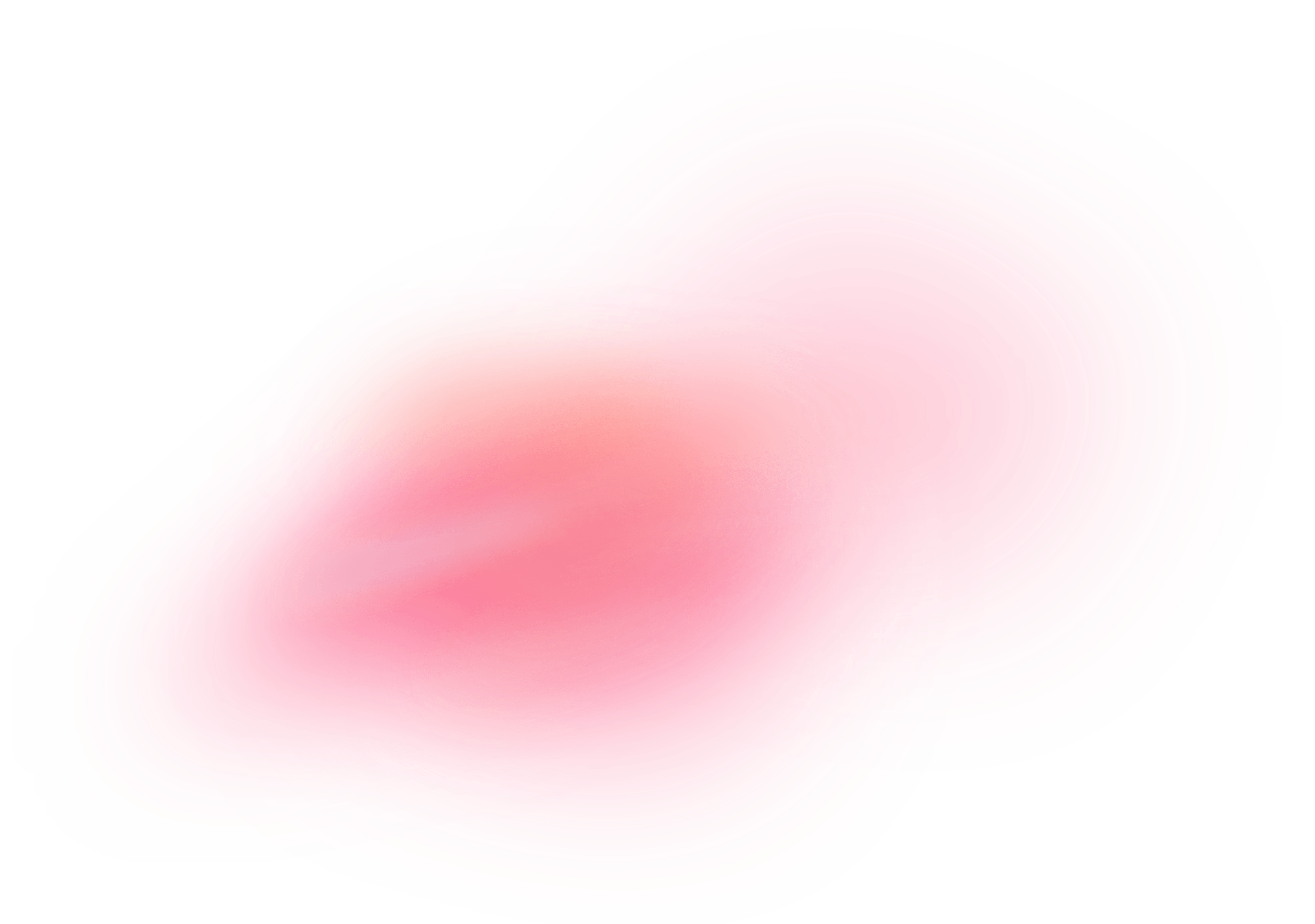