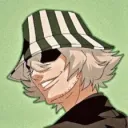
this is my auth.js:
import { Client, Account, ID } from "appwrite";
export class AuthService {
client = new Client();
account;
constructor() {
this.client
.setEndpoint(import.meta.env.VITE_APPWRITE_URL)
.setProject(import.meta.env.VITE_APPWRITE_PROJECT_ID);
this.account = new Account(this.client);
}
async createAccount({ email, password, name }) {
try {
const userAccount = await this.account.create(ID.unique(), email, password, name);
if (userAccount) {
return this.login({ email, password });
} else {
return userAccount;
}
} catch (error) {
throw error;
}
}
async login({ email, password }) {
try {
return await this.account.createEmailPasswordSession(email, password);
} catch (error) {
throw error;
}
}
async getCurrentUser() {
try {
return await this.account.get();
} catch (error) {
console.log("Appwrite service :: getCurrentUser :: error", error);
}
return null;
}
async logout() {
try {
await this.account.deleteSessions();
} catch (error) {
console.log("Appwrite service :: logout :: error", error);
}
}
}
const authService = new AuthService();
export default authService
and this is how i am saving my appwrite url in the .env file:
VITE_APP_APPWRITE_URL=https://cloud.appwrite.io/v1
Please help me to fix this

You're not using the name env variable

In the code you're using VITE_APPWRITE_URL in your .env file you're using VITE_APP_APPWRITE_URL
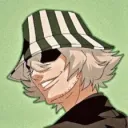
i fixed everything in my conf file, i am importing the .env variables from the conf file like in the second image and now i am getting this error:
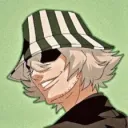
conf file:
const conf = {
appwriteUrl: String(import.meta.env.VITE_APP_APPWRITE_URL),
appwriteProjectId: String(import.meta.env.VITE_APP_APPWRITE_PROJECT_ID),
appwriteDatabaseId: String(import.meta.env.VITE_APP_APPWRITE_DATABASE_ID),
appwriteCollectionId: String(import.meta.env.VITE_APP_APPWRITE_COLLECTION_ID),
appwriteBucketId: String(import.meta.env.VITE_APP_APPWRITE_BUCKET_ID),
}
export default conf
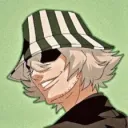
auth.js:
import conf from '../conf/conf.js';
import { Client, Account, ID } from "appwrite";
export class AuthService {
client = new Client();
account;
constructor() {
this.client
.setEndpoint(conf.appwriteUrl)
.setProject(conf.appwriteProjectId);
this.account = new Account(this.client);
}
async createAccount({email, password, name}) {
try {
const userAccount = await this.account.create(ID.unique(), email, password, name);
if (userAccount) {
// call another method
return this.login({email, password});
} else {
return userAccount;
}
} catch (error) {
throw error;
}
}
async login({email, password}) {
try {
return await this.account.createEmailSession(email, password);
} catch (error) {
throw error;
}
}
async getCurrentUser() {
try {
return await this.account.get();
} catch (error) {
console.log("Appwrite serive :: getCurrentUser :: error", error);
}
return null;
}
async logout() {
try {
await this.account.deleteSessions();
} catch (error) {
console.log("Appwrite serive :: logout :: error", error);
}
}
}
const authService = new AuthService();
export default authService
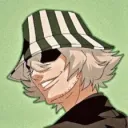
this is the error i am getting
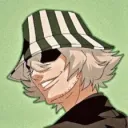
.env file:
VITE_APP_APPWRITE_URL=https://cloud.appwrite.io/v1
VITE_APPWRITE_PROJECT_ID="664-------c4"
VITE_APPWRITE_DATABASE_ID='66------48'
VITE_APPWRITE_COLLECTION_ID='66-----d'
VITE_APPWRITE_BUCKET_ID='66------d'
Recommended threads
- React native Google OAuth not working - ...
## Code: *Pretty much just copied from appwrite docs* ```javascript const signInWithOAuth = async (provider) => { setLoading(true); try { // Crea...
- 25 document limit
Unable to bypass the 25 document limit: https://github.com/Mooshieblob1/GenerateImagesPreview
- cant get custom domain to work with appw...
Hi, how do I get a custom domain to work? I have set database.vrtuhub.com on the appwrite console as a custom domain and did the cname record for it bit i still...
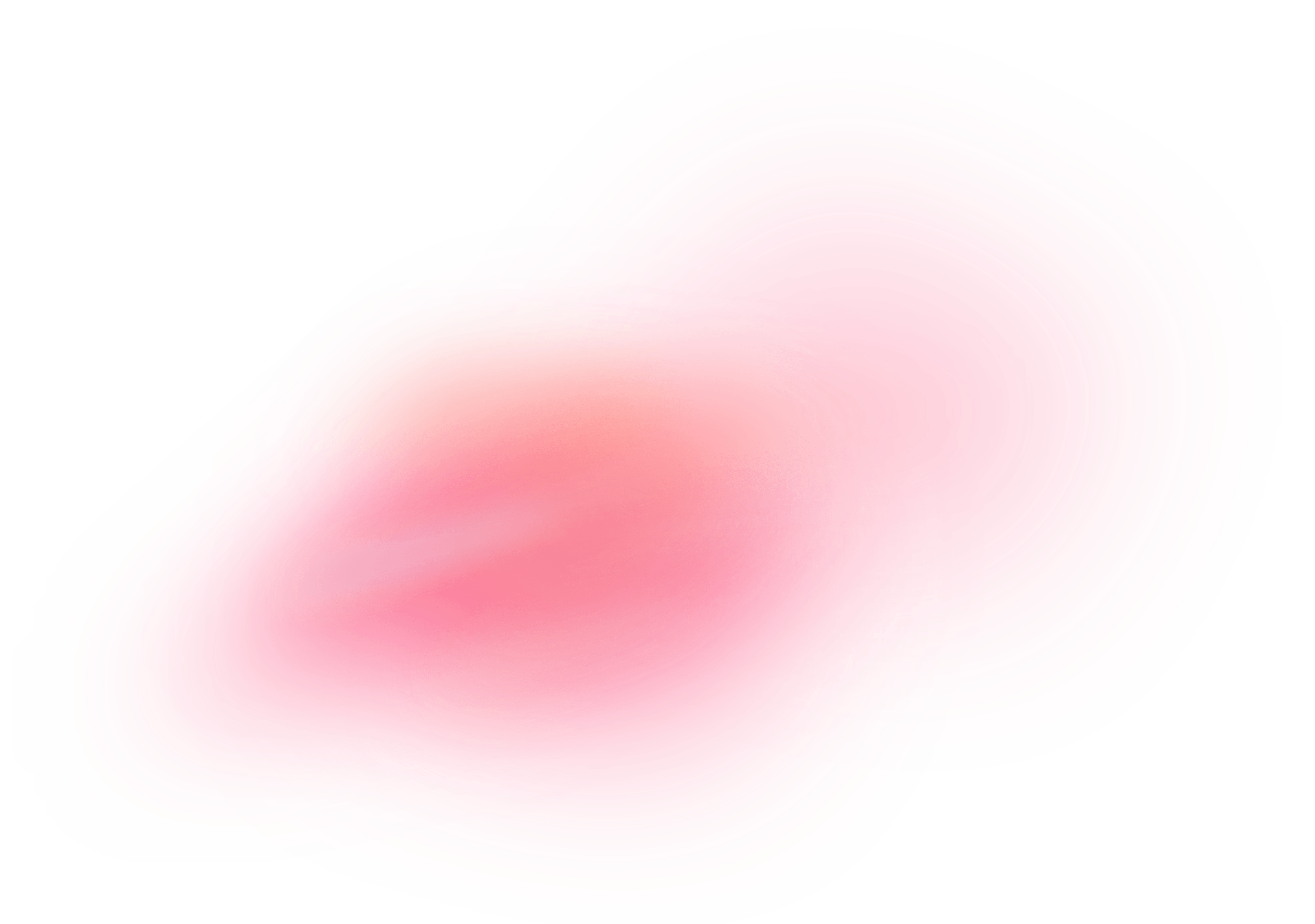