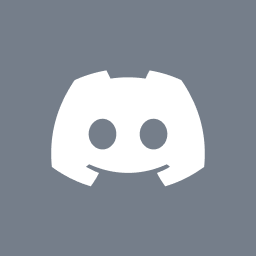
Consider this php function ```<?php
function sendEmailViaSendGrid($to, $subject, $text) { $apiKey = 'send_grid_api_key'; // Replace with your SendGrid API key
echo "Script Started\n";
$data = [
'personalizations' => [
[
'to' => [
['email' => $to]
]
]
],
'from' => ['email' => 'maurice.volaski@einsteinmed.edu'], // Replace with your email
'subject' => $subject,
'content' => [
[
'type' => 'text/plain',
'value' => $text
]
]
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.sendgrid.com/v3/mail/send');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
$result = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
}
if (curl_getinfo($ch, CURLINFO_HTTP_CODE) === 202) {
echo 'Email sent successfully.';
} else {
echo 'Error in sending email: ' . $result;
}
echo "Script Ended\n";
curl_close($ch);
}
sendEmailViaSendGrid('programming@fluxsoft.com', 'Test Subject', 'Test Email Body');
?> ``` It actually sends the email, but in appwrite, I get 1) "no response recorded" 2) under Logs and errors, "internal runtime error". What is causing that and how come I don’t see anything echoed?

no response recorded
is probably because you did not return anything as an execution output.- Not sure about this but there is a particular format for functions that needs to be followed, did you do that? See this section: https://appwrite.io/docs/functions#writingYourOwnFunction
Recommended threads
- 1.6 to 1.7 does not work
Appwrite (self-hosted) stopped working after updating from 1.6.2 that was stable. Getting the general_server_error. Mentioning I fallowed all steps for upgrad...
- Svelte App Whitelist
https://appwrite.io/docs/quick-starts/sveltekit Based on the example from the docs, how woul'd I go about adding a "whitelist" that checks if the user has a sp...
- How to have two Appwrite Instances in Sy...
Hello, I am new to Appwrite. I am currently developing a training app for our sport with a friend and we would like to use Appwrite for this. We would like to d...
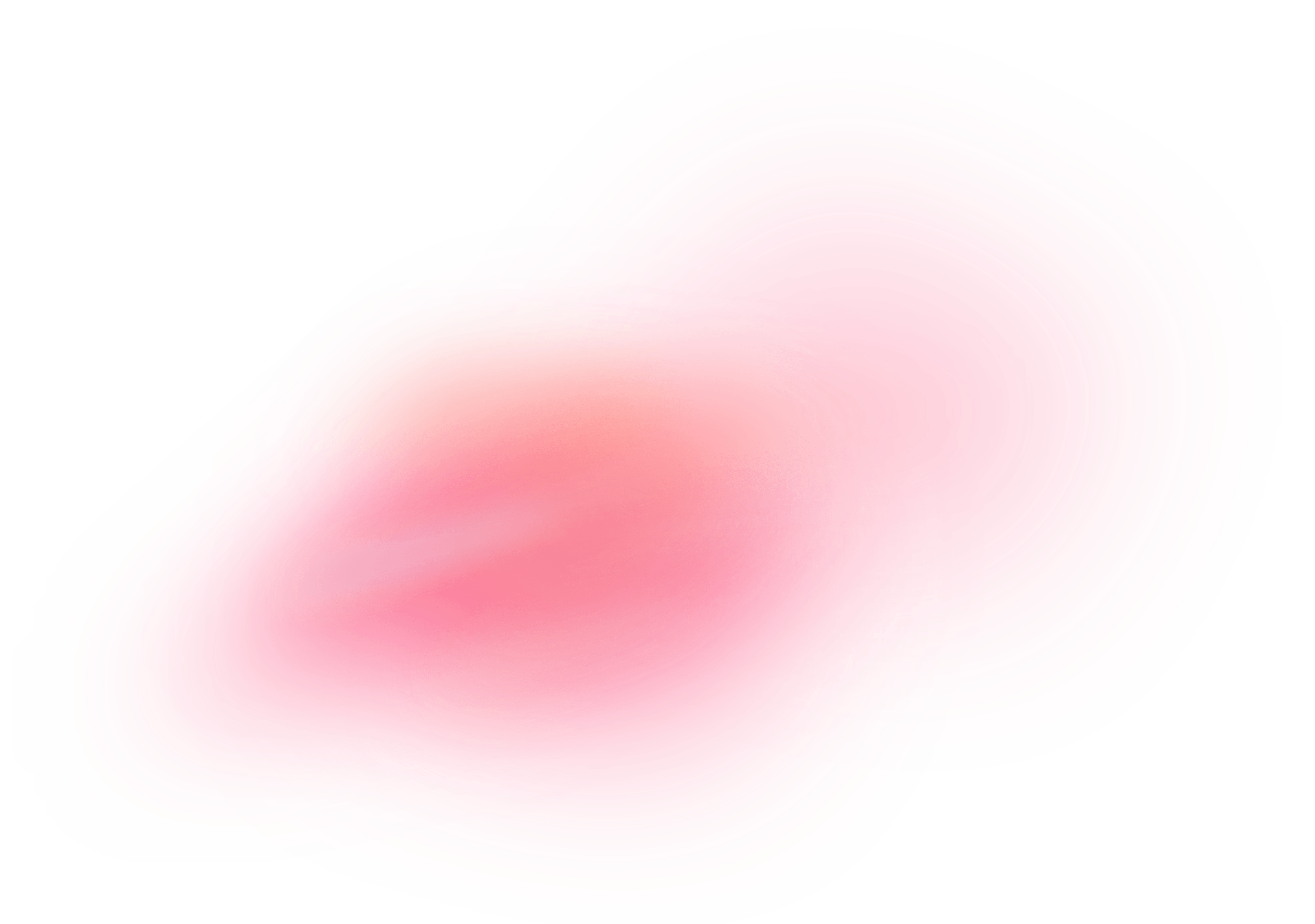