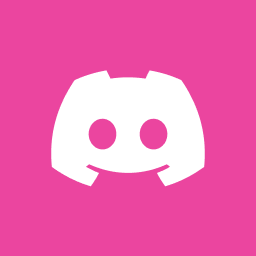
When creating an anonymous session server side I can set cookies this way:
default: async ({ fetch, cookies }) => {
try {
const response = await fetch(`${AppwriteEndpoint}/account/sessions/anonymous`, {
method: 'POST',
headers: {
'x-appwrite-project': AppwriteProject
}
});
const json = await response.json();
if (json.code >= 400) {
return fail(400, { message: json.message });
}
const ssrHostname = SsrHostname === 'localhost' ? SsrHostname : '.' + SsrHostname;
console.log(`ssrhostname = ${ssrHostname}`);
const appwriteHostname =
AppwriteHostname === 'localhost' ? AppwriteHostname : '.' + AppwriteHostname;
console.log(`appwritehostname = ${appwriteHostname}`);
const cookiesStr = (response.headers.get('set-cookie') ?? '')
.split(appwriteHostname)
.join(ssrHostname);
const cookiesArray = setCookie.splitCookiesString(cookiesStr);
const cookiesParsed = cookiesArray.map((cookie) => setCookie.parseString(cookie));
for (const cookie of cookiesParsed) {
cookies.set(cookie.name, cookie.value, {
domain: cookie.domain,
secure: cookie.secure,
sameSite: cookie.sameSite as any,
path: cookie.path,
maxAge: cookie.maxAge,
httpOnly: cookie.httpOnly,
expires: cookie.expires
});
console.log(`cookie: ${cookie.domain}`);
console.log(`cookie: ${cookie.path}`);
}
return json;
} catch (err: any) {
return fail(400, { message: err.message });
}
}
} satisfies Actions;```
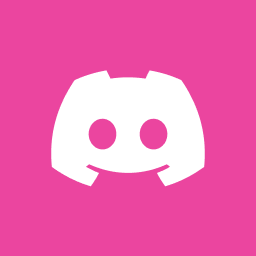
I want to do the same kind of logic when a user logs in. How can I achieve this.
body.email as string,
body.password as string
I see that I get a session object back.
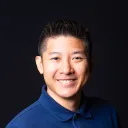
you need to manually make the API call just like how your sample code manually called the anonymous session endpoint so that you can grab the cookie the same way
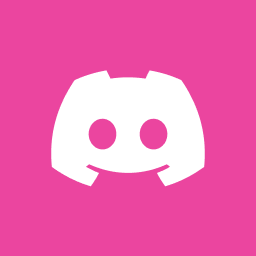
Ok, here is what i have so far. Hope it is in the right direction:
let payload: Payload = {};
if (typeof body.email !== 'undefined') {
payload['email'] = body.email;
}
if (typeof body.password !== 'undefined') {
payload['password'] = body.password;
}
const response = await fetch(`${AppwriteEndpoint}/account/sessions/email`, {
method: 'POST',
headers: {
'x-appwrite-project': AppwriteProject
},
body: JSON.stringify(payload)
});
console.log(response);
I'm just not sure on where to put the payload?
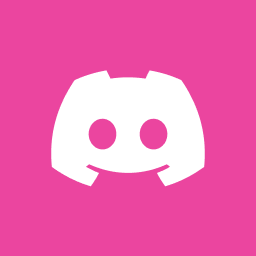
I'm getting back a bad request.
[Symbol(realm)]: null,
[Symbol(state)]: {
aborted: false,
rangeRequested: false,
timingAllowPassed: true,
requestIncludesCredentials: true,
type: 'default',
status: 400,
timingInfo: {
startTime: 67667657.25008106,
redirectStartTime: 0,
redirectEndTime: 0,
postRedirectStartTime: 67667657.25008106,
finalServiceWorkerStartTime: 0,
finalNetworkResponseStartTime: 0,
finalNetworkRequestStartTime: 0,
endTime: 0,
encodedBodySize: 114,
decodedBodySize: 0,
finalConnectionTimingInfo: null
},
cacheState: '',
statusText: 'Bad Request',
headersList: HeadersList {
cookies: null,
[Symbol(headers map)]: [Map],
[Symbol(headers map sorted)]: null
},
urlList: [ [URL] ],
body: { stream: undefined }
},
[Symbol(headers)]: HeadersList {
cookies: null,
[Symbol(headers map)]: Map(19) {
'access-control-allow-credentials' => [Object],
'access-control-allow-headers' => [Object],
'access-control-allow-methods' => [Object],
'access-control-allow-origin' => [Object],
'access-control-expose-headers' => [Object],
'cache-control' => [Object],
'content-encoding' => [Object],
'content-length' => [Object],
'content-type' => [Object],
'date' => [Object],
'expires' => [Object],
'pragma' => [Object],
'server' => [Object],
'x-content-type-options' => [Object],
'x-debug-fallback' => [Object],
'x-debug-speed' => [Object],
'x-ratelimit-limit' => [Object],
'x-ratelimit-remaining' => [Object],
'x-ratelimit-reset' => [Object]
},
[Symbol(headers map sorted)]: null
}
}
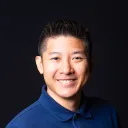
what's the response body?
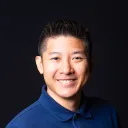
btw, it's helpful to include the language when adding multiline code so you have syntax highlighting
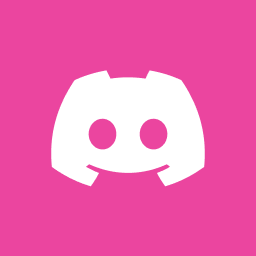
not sure how to do that
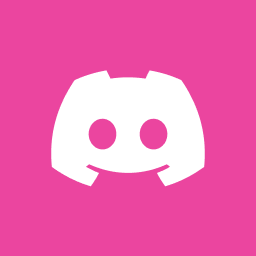
body: { stream: undefined }
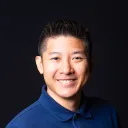
have you looked at any documentation for fetch?
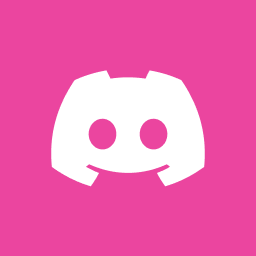
So when i do : console.log(JSON.stringify({payload}));
The body is : {"payload":{"email":"example@test.com","password":"password"}}
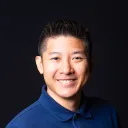
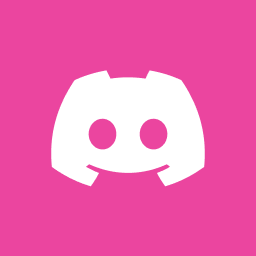
Yeah I looked at the REST, no example shows up to see how it works
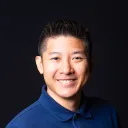
switch the version to 1.3
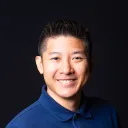
Make sure to also read the REST api docs: https://appwrite.io/docs/rest
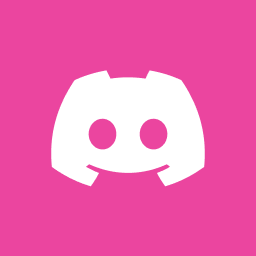
ahhhh That's what i needed. Thanks.
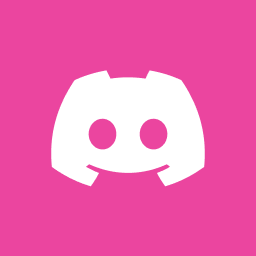
Thank You Seven for you patience with this. Those docs solved my issue.
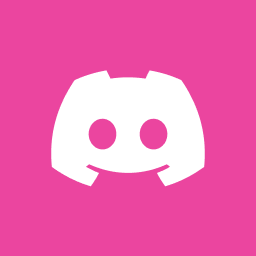
[SOLVED] Setting cookies
Recommended threads
- How To Send Email Verification From Serv...
How can I send email verification from server action or component after sign up in next.js ? Is there any way ?
- x-appwrite-user-jwt missing
Even for logged in users I can't see "x-appwrite-user-jwt" or "x-appwrite-user-id" in headers of an appwrite function. I'm trying to send "x-appwrite-user-id" m...
- ATTRIBUTE NOT SHOWING DELETE
Hi, I'm trying to delete an attribute in my collection but it won't delete, It's not even showing the delete when I click on the hamburger icon, Its showing onl...
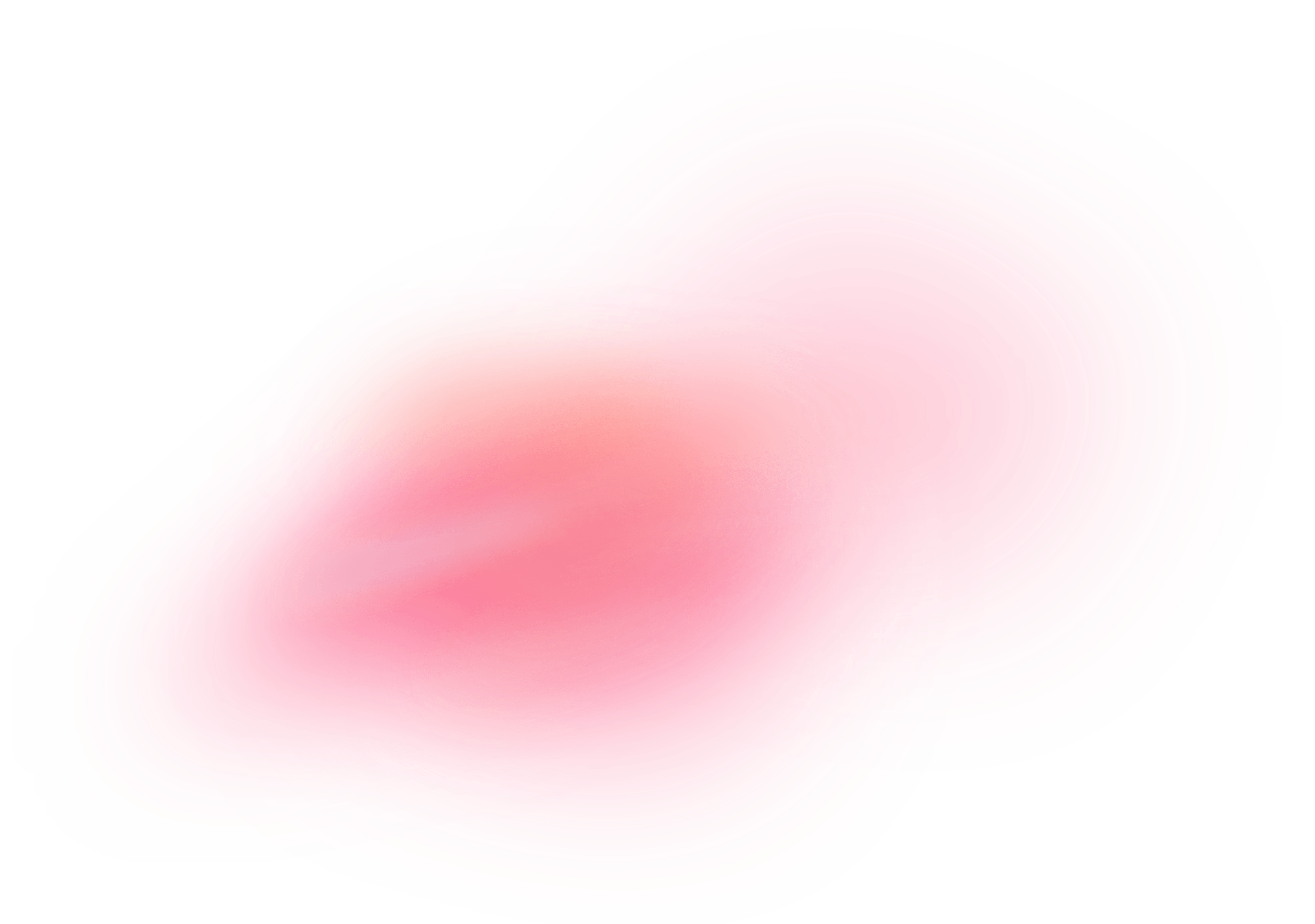