
Hopefully this isn't a complete dingus of a question.
Tl;dr I'm building a simple web-app with user-auth, upon log in a user is directed to their profile page. While logged in, if the user refreshes the page, they're logged out. Is this the correct response or do I not know what I'm doing?
The user logs in via email and password, an email session is created. I get their user information and send that to context so I can access their ID, name and avatar app-wide:
setLoading(true);
await account.createEmailSession(email, password);
const userInfo = await getCurrentUser();
authUser(userInfo);
navigate('/profile');
}
I'm using react-router to have protected paths, I have a helper function that will send a unauthorized user to the log-in if they try to access a restricted page such as profile.
Is the user logged out on refresh because, by default, appwrite uses localStorage for session management, and that's the correct action?
Is the solution to add a custom domain as an endpoint?
If so, can the app run on a subdomain and a different subdomain accesses the API? If I have example.com
, can I use demo-app.example.com
for the app and appwrite.example.com
for the API? Or does it need to be a subdomain - SLD relationship to work?
Thanks in advance!
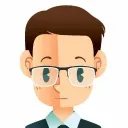
As Appwrite is your BaaS and your code will run in a body-less frontend you'll need to verify the account each time the pages loads with account.get()
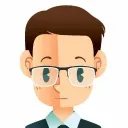
This is an example of the login flow https://discord.com/channels/564160730845151244/1111116935938187294/1111119219094994966
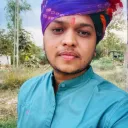
You need state persistence to achieve this. Refer this answer from stackoverflow

Oh I do! Sorry I blanked on that, that's what getCurrentUser
is, it returns the user data via account.get()
, which then gets sent to context.
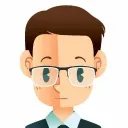
Good
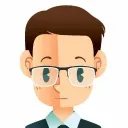
But, are you using the getCurrentUser
function in each page load?
Or only after success login?

Only after the success login, because with react-router there isn't typically a reload when changing between pages (at least that's how I understand it).
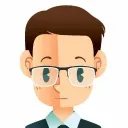
It makes sense, Yes.
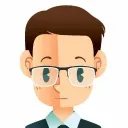
But you should add it in each reload - When you're initializing your app - so you'll cover also the case when the user is refreshing the page. Or when the user loads your website from a link or something.

Ooh that makes sense, thanks!

I tried that and it didn't work, I put a note to be logged when account.get()
was called, and I saw the note when expected but the session still gets terminated upon refresh. I think I need to find another way to handle session management.
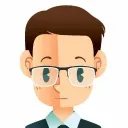
the session still gets terminated upon refresh I think this will continue to happened till you run the
account.get()
upon refresh.

I have the call where it'll run automatically when navigating to the different pages, and the console logged notes showed that was working. But on the refresh there isn't an anything for account.get()
to return, that's why I think it's with how the session is managed. Unless I'm not understanding something and have gone down the wrong path, certainly an option.
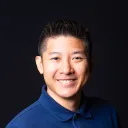
what's your tech stack? is that account.get() on refresh executing server side?

React (with react-router) and appwrite cloud. I checked and the account.get()
is not executing server side on a refresh. I don't think it can because the refresh ends the session, so the user is directed to the log-in screen automatically, maybe?
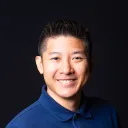
not nextjs?
client side, the SDK should be persisting the session

No, I'm still working on learning nextjs, I was late to the game.
That's what I'd understood from the docs, which is why I don't understand my issues. I don't have a domain name set, so I get the console message "Appwrite is using localStorage for session management. Increase your security by adding a custom domain as your API endpoint." upon log-in, but should that matter if I'm deploying locally while developing? I can see it acting up when it's deployed on Vercel, but locally..?
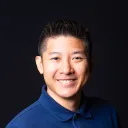
so when your app starts, are you calling account.get()
to check if they have a valid session?
can you share some of your code?

Ahhhhh thank you! That's where I went wrong! I moved it to a different component, it works properly now. Hot dang, it's just that easy if you put everything in the right place 😅 😂 Thank you so much for your help!
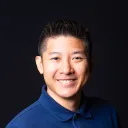
[SOLVED] User session terminated on refresh
Recommended threads
- Error getting session: AppwriteException...
I get this error `Error getting session: AppwriteException: User (role: guests) missing scope (account)` when running in prod. As soon as I try running my app o...
- PR Review and Issue Assign?
I am not familiar with how things work here. I know that Issue have to be assigned before solving problem, It is for not wasting contributors time but I like t...
- Need help with clerk auth
Im having issue with auth
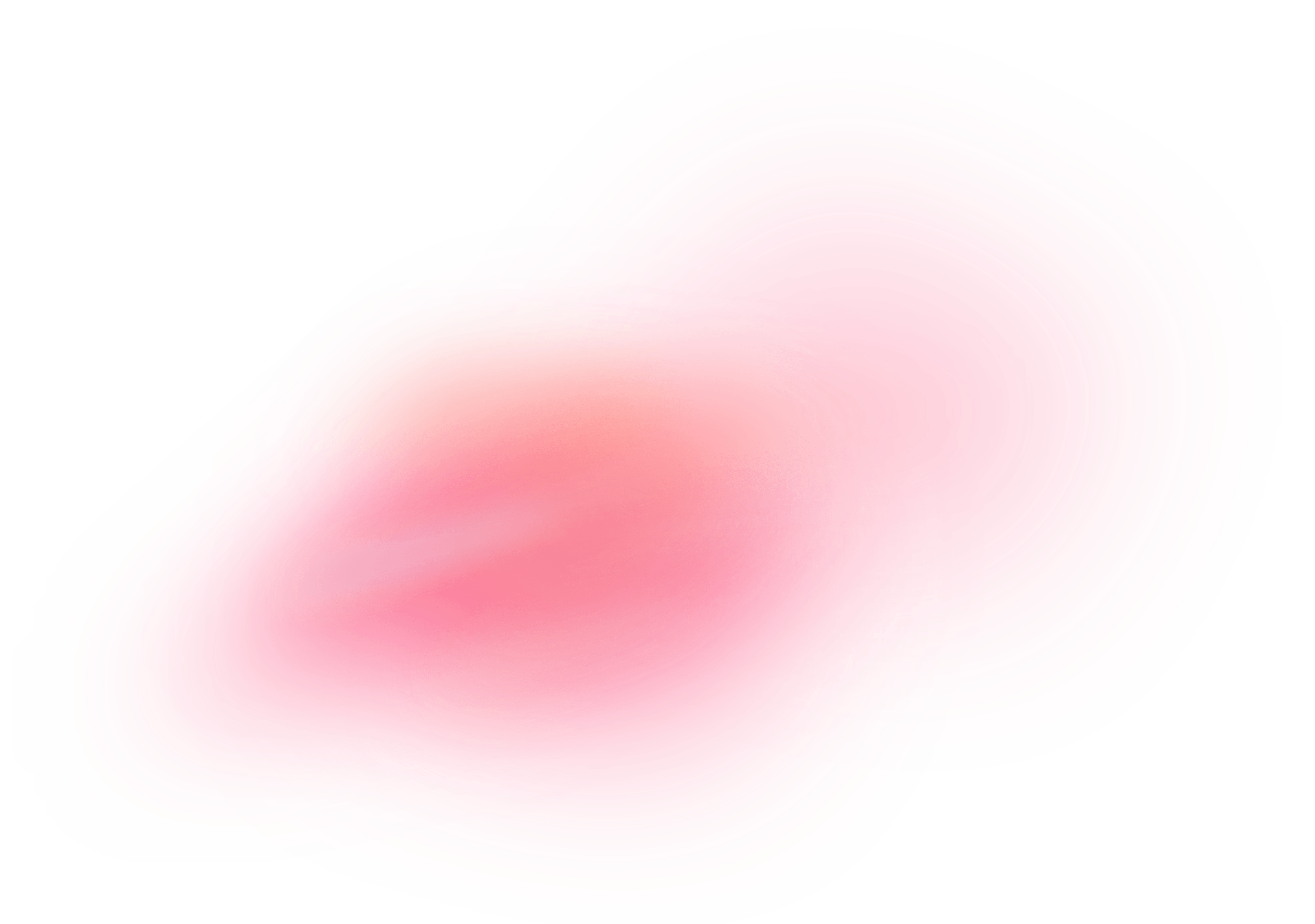