
import 'dart:core';
import 'dart:convert';
import 'package:dart_appwrite/dart_appwrite.dart';
Client client = Client();
Users users = Users(client);
Future<void> start(final req, final res) async {
//wrap in big try catch as per stevens instructions
try {
// Init SDK
print("instantiated the client and user");
final endpoint = req.variables['APPWRITE_FUNCTION_ENDPOINT'] ??
'SECRET_KEY variable not found. You can set it in Function settings.';
final projectID = req.variables['FUNCTION_PROJECT_ID'] ??
'PROJECT_ID variable not found. You can set it in Function settings.';
final apiKey = req.variables['APPWRITE_FUNCTION_API_KEY'] ??
'API_KEY variable not found. You can set it in Function settings.';
print("set up Hooks");
print(endpoint);
print(projectID);
print(apiKey);
print(req);
print(res);
client
.setEndpoint(endpoint) // Your API Endpoint
.setProject(projectID) // Your project ID
.setKey(apiKey) // Your secret API key
;
Map<String, dynamic> payload = jsonDecode(req);
print(payload);
print("decoded payload: $payload");
String? name = payload["name"];
String? password = payload["password"];
String? email = payload["email"];
print("name: $name, password: $password, email: $email");
if (name == null || password == null || email == null) {
res.json({"message": "Some fields are missing"}, status: 400);
return;
}
await users.create(
userId: ID.unique(),
email: email,
password: password,
name: name,
);
/*Future result = users.create(
userId: ID.unique(),
email: "test2@test.com",
password: "testtest",
name: "tester2",
);
*/
res.json({"message": "user created", "status": 200});
print("$name, $password, $email");
} on AppwriteException catch (e) {
print(e.message);
}
}

sorry for the late response, here is the code i have presently!
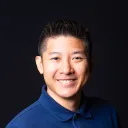
change the catch to:
} catch (e) {
print(e.toString());
res.json({"error": e.toString()});
}
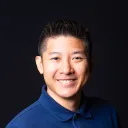
also don't print these:
print(req);
print(res);
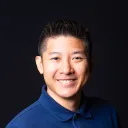
and you shouldn't be decoding req
. you should be decoding req.payload

oh perfect! I think thats why i wasnt recieving anything! im now getting data through! Thank you so much, its now getting most of the way through but its throwing this error now
general_route_not_found, Not Found (404)
but i think i know why thats happening I removed the v1 from the endpoint to see if maybe I wasn't supposed to add the v1/users to the url, so I think if I fix that it should run as expected, will keep you in the know! let me give it a shot!
and again I would like to thank you steven! (also the other two who came to help as well, Nimit and Safwan thank y'all for hopping in to assist as well! Yall helped make a few connections in my head and that helps a ton! So thank you both as well!) Y'alls assistance is much appreciated <a:Prayge2:846611105602338836>

May I ask on this, why was on appwrite exception
throwing the curl error? was I improperly using on appwrite exception
?

is Appwrite injecting Appwrite exception in place of regular exceptions? ie i call appwrite exception and its trying to recursively (i think this would be the right terminology) wrap the appwrite exception in an appwrite exception? or is something else happening with the Appwrite exception to cause it to throw the curl error? (its more than likely me just mis-using Appwrite exception but thought id ask incase theres a little more to it)

after fixing the above issues (including adding back v1/users to the endpoint) I am now receiving this error
AppwriteException: general_unauthorized_scope, User (role: guests) missing scope (users.write) (401)
my api key has user read and write permissions, do I need to also manually set permissions in the appwrite function itself as well? I thought my api key handled the permissions entirely
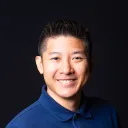
another part of your code was throwing an error, but because it wasn't thrown by the SDK, it was a different exception so your catch didn't catch the exception
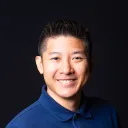
role: guests
means your API key is invalid or you didn't call setKey()

(by the way thank you for bearing with me through this process i know some of these questions are probably working with databases 101)
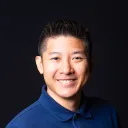
no problem! happy to help!
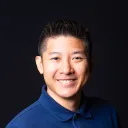
you might be missing the FUNCTION_PROJECT_ID
variable

I think i removed the FUNCTION_PROJECT_ID
since i read on the docs that the project id is passed to the function, is FUNCTION_PROJECT_ID
different from PROJECT_ID
?
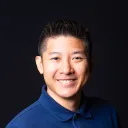
APPWRITE_FUNCTION_PROJECT_ID
is automatically supplied. See https://appwrite.io/docs/functions#functionVariables

ooohhhh so its supplied, but its not implemented for me I still have to take what its giving me and apply it otherwise its basically just an unused variable?
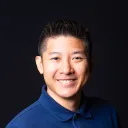
yes...and note it's APPWRITE_FUNCTION_PROJECT_ID
, not FUNCTION_PROJECT_ID
or PROJECT_ID

Gotcha! so should be a rather easy fix, so I need to supply the FUNCTION_PROJECT_ID
and the PROJECT_ID
Appwrite takes care of supplying APPWRITE_FUNCTION_PROJECT_ID
?
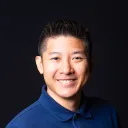
no, just use req.variables['APPWRITE_FUNCTION_PROJECT_ID']

It works! <a:HYPE:676250466073575461> Thank you so much Steven!

Hello all,
So I've been reading through the various talks on the server/support topics here plus reading through the docs a bit and I was wondering a few things! Before my questions I'd also like to correct some of the information I put above (for clarification sake just incase its a factor in things) my appwrite instance is self hosted, but not done so locally. Please anyone feel free to answer, even if its not every question as I can gleam what information I can
1.A) executing a Login/Signup from the client side app was super easy...- when I wasn't using Appwrite functions to do most of the heavy lifting for me. From what I've gathered from reading it is ill-advised to include an API key in the client... How would I go about sending the information to my Appwrite function for verification and receiving the response back (for logging in and signing up) without an API key? (originally, I was using a super limited API key that only had the ability to execute functions from the client side. Based off of what I've been seeing here, and reading on the docs that doesn't seem like the proper way to do things!)
I've read a few places on the docs a JWT token is required for the server function to do things such as signup/create a session/login on behalf of the client. If so can you point me to some good resources on how to properly generate a JWT token (and which side to do so on (generating as well as proper way to send) server or client side) or some examples of these being done from a flutter app by means of using an Appwrite function executed from the client visual examples are definitely preferred if at all possible, either that be video showing the process or a code demo is super useful
1.B) for the JWT token I read somewhere the server can generate it and pass it off to the client in an environment variable (and from what I could imagine would be the proper way to do so as they only last for 15 minutes, but I may be mistaken and if so please correct me)
2.) I noticed that the server uses the dart_appwrite dependecy, are there conflicts with using that in the client as well?
From what I'm able to tell from my reading I should be using appwrite ^<current_version>
for the client as a dependency while any appwrite functions I write I should be using dart_appwrite ^<current_version>
<:this:808718890589880371> is this assertion based on my reading correct? (wanting to cover a few bases with these questions so please bear with me)
3.) is there a better way to swap my endpoint? right now for my function I'm taking the instantiated client and just setting the endpoint again when I need to swap from one to another, is there a better way to do so? Will any problems arise from me just setting the endpoint later in the script like I do when setting up the function? (so far I haven't noticed any issues from the appwrite function by doing so, but want to double check just incase) I think I remember reading something about a setEndpointRealtime()
? Is that the proper way to temporarily swap the endpoint when within a client application?
4.) Do I need to be using Http if I'm using appwrite ^<current_version>
client side to actually send my requests? Will I need to start using it if I start using JWT tokens?
5.) I read something about a CookieJar being tangentially related to JWT token, Do I need to set one up flutter side to use JWT if so any recommended dependency for a CookieJar for flutter?
Sorry again for the wall of text! Any and all answers are greatly appreciated <a:Prayge2:846611105602338836>
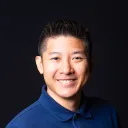
It's best to use separate <#1072905050399191082> posts for distinct topics so it might be best to use a new post for this

Oh gotcha my apologies I can do that!
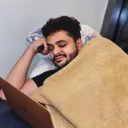
No worries! Feel free to make posts and I'll be happy to try and answer your questions 😁
Recommended threads
- Redirect URL sends HTTP instead of HTTPS...
I am not sure since when this issue is present, but my Google and Apple redirect URI are no longer pointing to the HTTPS redirect URI when I try to use OAuth. ...
- Failing to run document operations on sd...
Could someone point me in the right direction I'm going in cirlces. I have a problem with sdks and my self-hosted server in production (for ~3 years) I have bee...
- Functions fail to deploy after switching...
Hi <@1087889306208718959> , after switching my self-hosted Appwrite instance to use AWS S3 as the storage backend, my Cloud Functions stopped working. I’m runni...
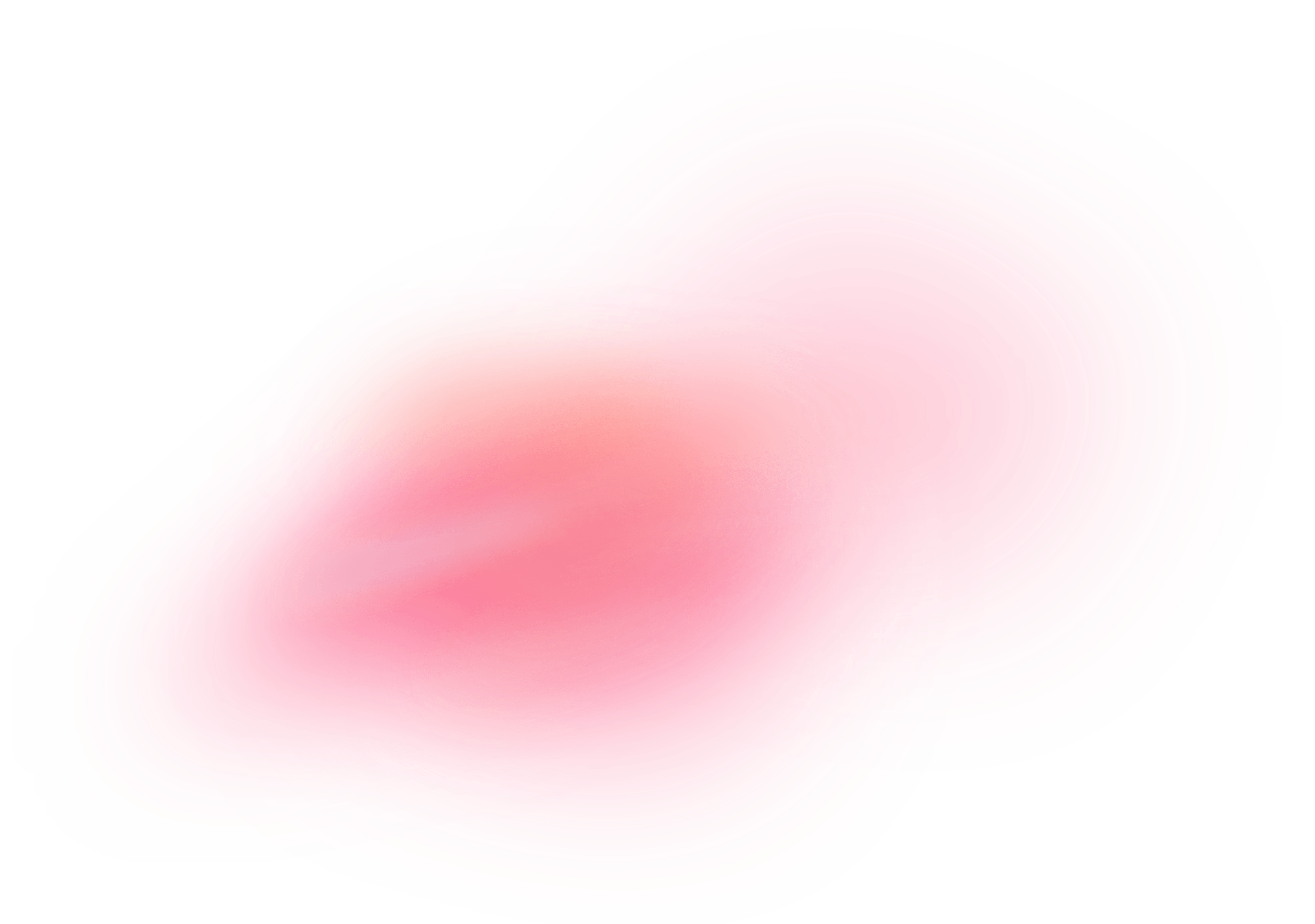