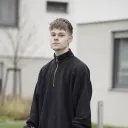
I use the following code in flutter for the session creation for the login with apple:
try {
final response = await account.createOAuth2Session(
provider: 'apple',
success: "https://XX.com/auth/oauth2/success",
failure: "https://XX.com/auth/oauth2/failure",
);
print(response);
return response;
} on AppwriteException {
rethrow;
}
}````
To open the login page I use:
```ElevatedButton(
child: const Text('Login with Apple'),
onPressed: () async {
await AuthHelper().loginWithApple().then((value) {
prefs.setSessionId(value.$id);
Navigator.of(context).pushReplacement(
MaterialPageRoute(
builder: (context) => const HomePage(),
),
);
}).onError((error, stackTrace) {
print(error);
});
},
),````
In the simulator I get the error seen in the screenshot. When I run the app on my iPhone with the cable connected the page opens fine, but when I click on login I get the error that the login is not possible ("registration not completed"). I host appwrite on digitalocean with a custom (sub-)domain.
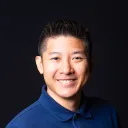
This typically happens because of some mismatch between what was entered into Appwrite for the OAuth2 and Appwrite. This might help: https://dev.to/appwrite/apple-sign-in-with-appwrite-2576
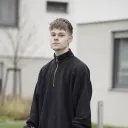
Yeah I used this tutorial but it didn’t work.
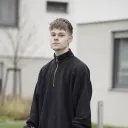
Maybe you can tell me what domain and redirect uri I should add? The redirect uri should be the one from the appwrite Formular? And the domain just the domain from the appwrite instance. But it doesn’t work for me this way. Nothing comes back. Do I have to modify the success and failure URLs?
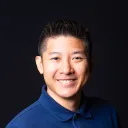
i've followed the tutorial before so it should work 🧐
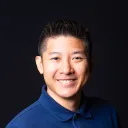
Maybe you can tell me what domain and redirect uri I should add?
The domain should be your appwrite domain. the redirect url should be something like:
https://[DOMAIN]/v1/account/sessions/oauth2/callback/apple/[PROJECT ID]
Do I have to modify the success and failure URLs? These are unrelated to the error you're getting.
Make sure your Service ID in Apple matches the Bundle ID you put in Appwrite.
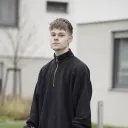
Yeah the problem was with the Service ID that has to match the Bundle ID in Appwrite. Thanks a lot!
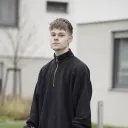
[SOLVED] Flutter Login with Apple
Recommended threads
- Apple OAuth Scopes
Hi Hi, I've configured sign in with apple and this is the response i'm getting from apple once i've signed in. I cant find anywhere I set scopes. I remember se...
- Sign In With Apple OAuth Help
Hi All! I've got a flutter & appwrite app which Im trying to use sign in with apple for. I already have sign in with google working and the function is the sam...
- [SOLVED] OAuth With Google & Flutter
Hi all, I'm trying to sign in with google and it all goes swimmingly until the call back. I get a new user created on the appwrite dashboard however the flutte...
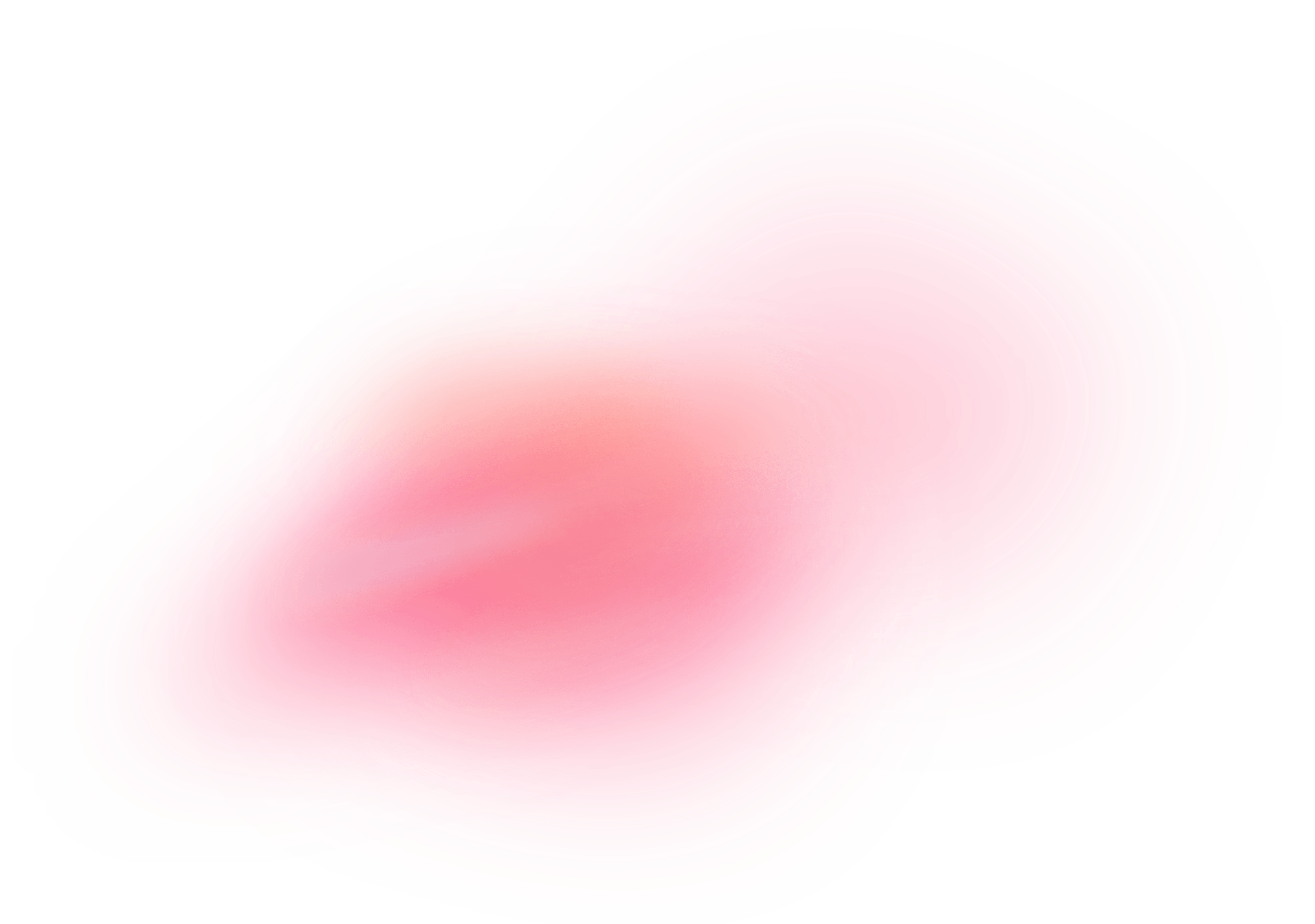