[SOLVED] Appwrite function that creates entry in database
- 0
- Tools
- Accounts
- Databases
- Users
- Functions
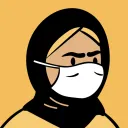
I"m having hard time doing a simple thing. I cannot get this to work. I found this example repo, https://github.com/Meldiron/almost-casino/ which was the only one showing how to create user related data on account creation, so I gave it a shot. I've edited the parts with sdk.Database(due the sdk api change) and added missing entries for db name.
const sdk = require("node-appwrite");
module.exports = async function (req, res) {
// Init SDK
const client = new sdk.Client();
const databases = new sdk.Databases(client);
if (
!req.env['APPWRITE_FUNCTION_ENDPOINT'] ||
!req.env['APPWRITE_FUNCTION_PROJECT_ID'] ||
!req.env['APPWRITE_FUNCTION_USER_ID'] ||
!req.env['APPWRITE_FUNCTION_API_KEY']
) {
throw new Error("Missing environment variables.");
}
const userId = req.env['APPWRITE_FUNCTION_USER_ID'];
console.log(userId, "thats my id");
const apiKey = req.env['APPWRITE_FUNCTION_API_KEY']
console.log(apiKey, "thats my api key")
const project = req.env["APPWRITE_FUNCTION_PROJECT_ID"]
console.log(project, "that's my project")
const endpoint = req.env['APPWRITE_FUNCTION_ENDPOINT']
console.log(endpoint, "that's my endpoint")
client
.setEndpoint(endpoint)
.setProject(project)
.setKey(apiKey);
let profile;
try {
profile = await databases.getDocument('profiles', 'profiles', userId);
} catch (err) {
console.log(err, "User profile not found, trying to make one")
profile = await databases.createDocument('profiles', 'profiles', userId, {balance: 500.0});
}
res.json({
profile
});
};
I don't even see the console logs, just the error about Cannot read property APPWRITE_FUNCTION_ENDPOINT
Any help would be appreciated, I have wasted 7 hours with this, and pretty much got stuck in an endless loop of doing the same thing over and over.
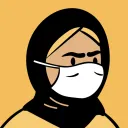
The function is deployed via CLI, in the right place, i see execution of it but I get error.
TypeError: Cannot read property 'APPWRITE_FUNCTION_ENDPOINT' of undefined
This is the front end execution of the function after anonymous login.
import { Appwrite, type RealtimeResponseEvent, type Models } from 'appwrite';
const appwrite = new Appwrite();
appwrite
.setEndpoint(import.meta.env.VITE_APPWRITE_ENDPOINT)
.setProject(import.meta.env.VITE_APPWRITE_PROJECT_ID);
export type Profile = {
balance: number;
} & Models.Document;
export class AppwriteService {
// .....
// Profile-related
public static async getProfile(userId: string): Promise<Profile> {
const response = await appwrite.functions.createExecution('createProfile', undefined, false);
if (response.statusCode !== 200) {
throw new Error(response.stderr);
}
return JSON.parse(response.response).profile;
}
}
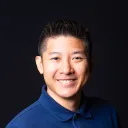
Another problem with this is the variables are in req.env
, but in newer versions of Appwrite, the variables are in req.variables
.
Make sure to read through the latest docs on writing functions: https://appwrite.io/docs/functions#writingYourOwnFunction
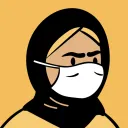
Ok that helped a bit, to get the userid, and projectid, but there are no variables for APPWRITE_FUNCTION_ENDPOINT and APPWRITE_FUNCTION_API_KEY. Are they even needed? Its a retorical question, as i still get 404 with out them. here is the output of req. ```js
{"variables":
{"APPWRITE_FUNCTION_ID":"createProfile",
"APPWRITE_FUNCTION_NAME":"createProfile",
"APPWRITE_FUNCTION_DEPLOYMENT":"66408d5a0175a68bbdaeb",
"APPWRITE_FUNCTION_PROJECT_ID":"almostCasino",
"APPWRITE_FUNCTION_TRIGGER":"http",
"APPWRITE_FUNCTION_RUNTIME_NAME":"Node.js",
"APPWRITE_FUNCTION_RUNTIME_VERSION":"16.0",
"APPWRITE_FUNCTION_DATA":"",
"APPWRITE_FUNCTION_USER_ID":"w6408d5ab75327e3948ae",
"APPWRITE_FUNCTION_JWT":"123asdf1rt1.eyJ1c2VySWQiOiI2NDA4ZDVhYjc1MzI3ZTM5NDhhZSIsInNlc3Npb25JZCI6IjY0MDhkNWFiNzYxNzIyNjZiOTQ0IiwiZXhwIjoxNjc4MzAxNDg3fQ.PZXMehwrDUMpjJnBHlskFYvuB9GKpshhoBHGgPcCKns"},
"headers":{},
"payload":""}
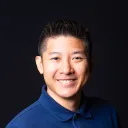
yes they are needed. you have to create those variables.
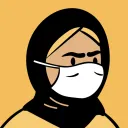
its the secret api i have created when creating the global appwrite instance? or its local to the project?
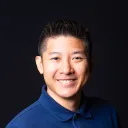
Maybe this will be helpful: https://appwrite.io/docs/getting-started-for-server
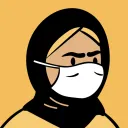
finally! Thanks @Steven
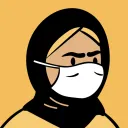
i had to make a costum api key for the project, and add scopes, for the usage, to be able to auth properly.
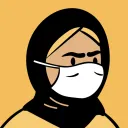
ok so we can use jwt to get the users stuff from his perspective, and its active 15min. Is the jwt auto reissued or we have to request it from time to time again ?
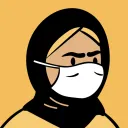
Im trying to think of a user that has been logged in for lets say 30minutes and wants to upload something, his jwt would be expired already in those 30minutes. So what would be a classic case in this scenario?
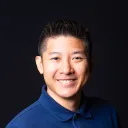
When a user executes a function, the JWT env var is auto-populated.
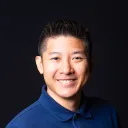
That said, I'm not sure what else you're doing in your function....The code you showed before suggests you don't need the JWT token because you're not doing anything on behalf of the user
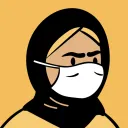
const sdk = require("node-appwrite");
module.exports = async function (req, res) {
const client = new sdk.Client();
const databases = new sdk.Databases(client);
const apiKey = req.variables["APPWRITE_FUNCTION_API_KEY"];
const endpoint = req.variables["APPWRITE_FUNCTION_ENDPOINT"];
const userId = req.variables["APPWRITE_FUNCTION_USER_ID"];
const project = req.variables["APPWRITE_FUNCTION_PROJECT_ID"];
if (!userId | !project | !apiKey | !endpoint) throw new Error("Missing environment variables.")
client
.setEndpoint(endpoint)
.setProject(project)
.setKey(apiKey);
let profile;
try {
profile = await databases.getDocument("profiles", "profiles", userId);
} catch (err) {
profile = await databases.createDocument("profiles", "profiles", userId, {
balance: 500.0,
});
}
res.json({
profile,
});
};
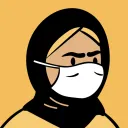
yes, it was a side question, i wanted to understand how it works. Thanks, I guess we can mark this thread as solved, thank you very much, With your help, I'm finally done with this long quest. 😉 <:appwriterocket:823996226894692403>
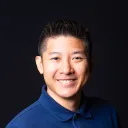
[SOLVED] Appwrite function that creates entry in database
Recommended threads
- Failed to verify JWT. Invalid token: Exp...
Hi I am trying to call a function from my mobile app, but I am receiving "Invalid token expired." My code looks more or less like this ```ts // from my app ...
- How do I pair Polar.sh + Hono + Appwrite...
This is what all it required by polar to see the webhook data. Managing with webhook data is not an issue, but how do I pair this all with appwrite functions st...
- The function became slower after being e...
I used the Python SDK and set `xasync=True` in `create_execution`, expecting it to execute quickly on another worker. However, the execution ended up taking sev...
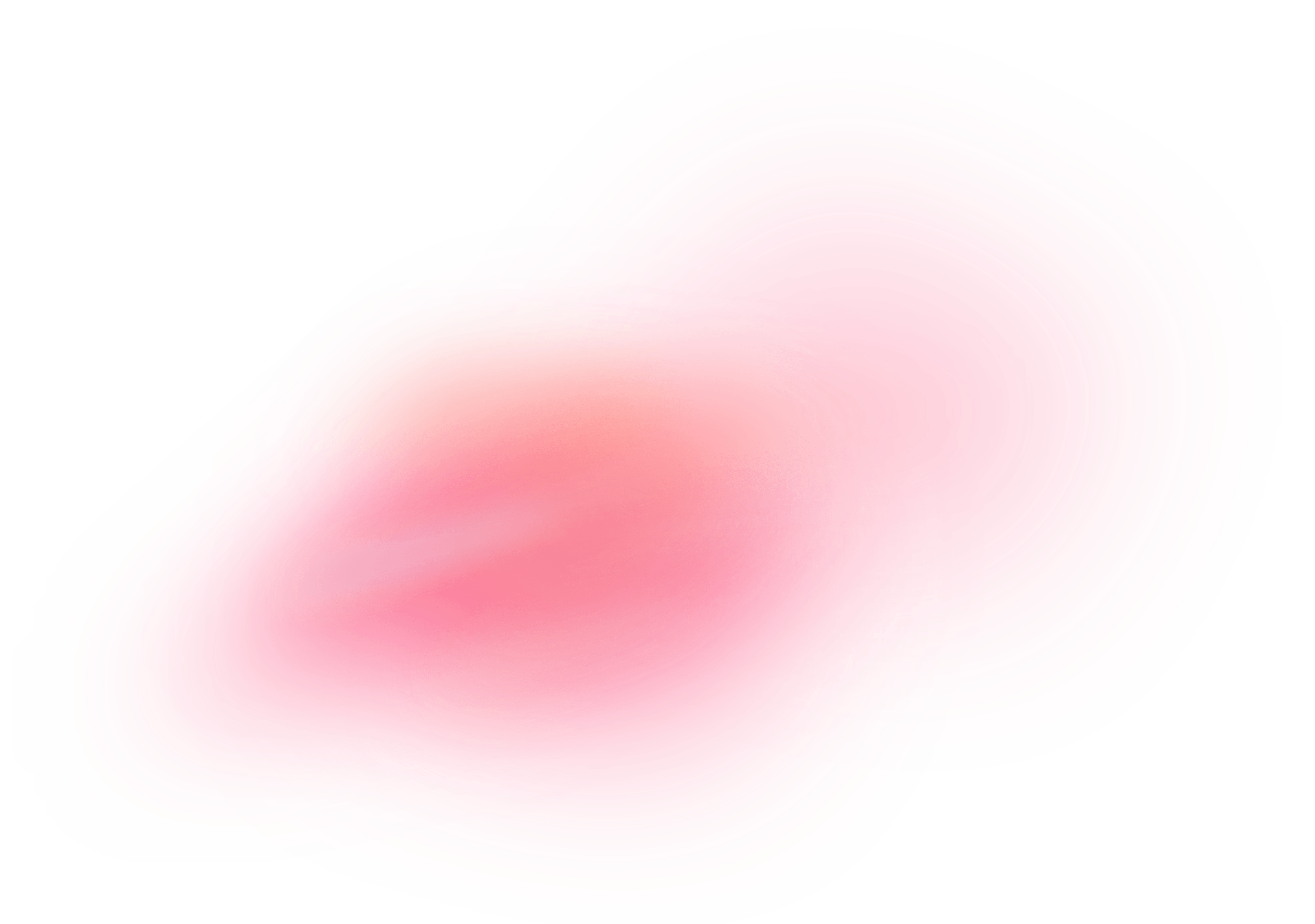