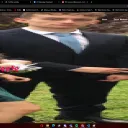
Code:
Pretty much just copied from appwrite docs
TypeScript
const signInWithOAuth = async (provider) => {
setLoading(true);
try {
// Create a deep link that works across Expo environments
// Ensure localhost is used for the hostname to validation error for success/failure URLs
const deepLink = new URL(makeRedirectUri({ preferLocalhost: false }));
if (!deepLink.hostname) {
deepLink.hostname = 'localhost';
}
console.log("deeplink: ", deepLink)
const scheme = `${deepLink.protocol}//`; // e.g. 'exp://' or 'playground://'
console.log("scheme: ", scheme)
// Start OAuth flow
const loginUrl = await account.createOAuth2Token(
provider,
`${deepLink}`,
`${deepLink}`,
);
console.log("loginUrl: ", loginUrl)
// Open loginUrl and listen for the scheme redirect
const result = await WebBrowser.openAuthSessionAsync(`${loginUrl}`, scheme);
console.log("openAuthSessionAsync: ", result)
// Extract credentials from OAuth redirect URL
const url = new URL(result.url);
const secret = url.searchParams.get('secret');
const userId = url.searchParams.get('userId');
console.log("url: ", url)
// Create session with OAuth credentials
const session = await account.createSession(userId, secret);
// Redirect as needed
console.log(session)
const profile = await account.get();
console.log(profile)
onSuccess(profile);
} catch (err) {
onError(err);
} finally {
setLoading(false);
}
};
Logs:
schemename
is the placeholder for the actual name set for scheme
in app.json
TypeScript
deeplink: "schemename://localhost"
scheme: schemename://
loginUrl: "https://cloud.appwrite.io/v1/account/tokens/oauth2/google?success=schemename%3A%2F%2Flocalhost&failure=schemename%3A%2F%2Flocalhost&project=projectId"
TL;DR
Developers are encountering a Google OAuth issue with a redirect_uri_mismatch. The code appears to be copied from the Appwrite docs. The issue seems to be related to the deep link and scheme used. The logs show the creation of the deep link and scheme. Checking if the scheme set in app.json matches the one passed in the code could resolve the redirect_uri_mismatch problem.Recommended threads
- More fields from Discord OAuth
Is it possible to save more infomation from Discord OAuth? For example Profile Pictures, Global Names, Flags, ... ```json "user": { "id": "26847331098...
- White Screen of Death
I am building an android application which is using React native(EXPO) and Appwrite. But While using the App in the Expo Go or Orbit it works perfectly wihout e...
- Is Expo SDK 53 compatible with Appwrite?
After upgrading to 53 and When using createEmailSession I am getting following error: Invariant Violation: TurboModuleRegistry.getEnforcing(...): 'PlatformCons...
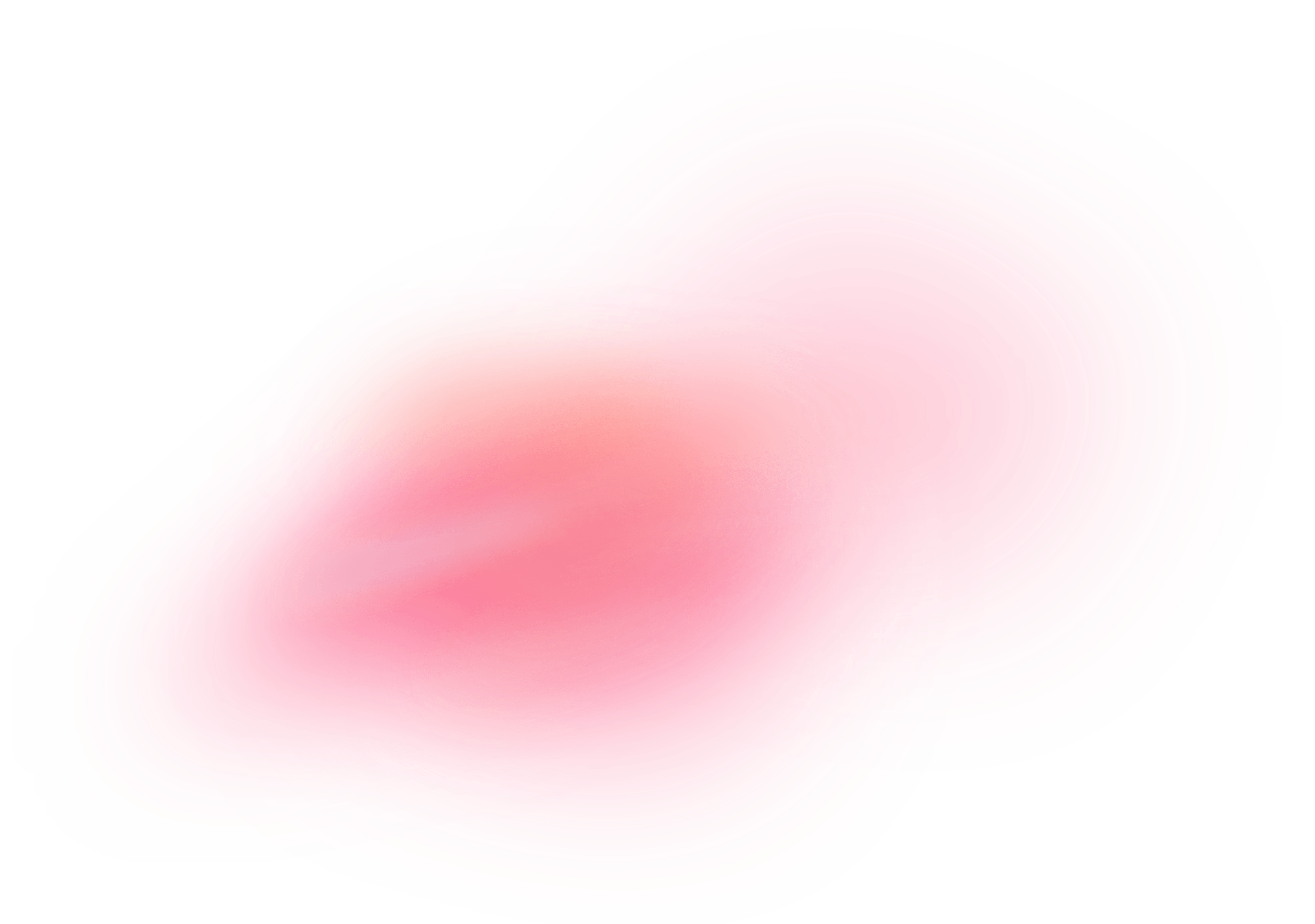