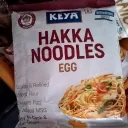
// Preparing the file `const setImageToCloud = async () => { setLoading(true); try { // Determine MIME type based on file extension if (!selectedImage) { throw new Error("No image selected"); }
const response = await fetch(selectedImage.uri); // fetch the local file
const blob = await response.blob(); // get file data as Blob
const fileObj = new File([blob], "photo.jpg", { type: blob.type });
// Now upload to Appwrite:
const res = await createFile({ file: fileObj! });
if (res.success) {
console.log("Image uploaded successfully");
setHideDrawer(true);
setImage(selectedImage?.uri!);
} else {
console.log("Image upload failed");
Alert.alert("Image upload failed");
}
} catch (error) {
console.log("Error uploading image", error);
Alert.alert("Error uploading image");
} finally {
setLoading(false);
}
};`
// For uploading the file to Appwrite `export const createFile = async ({ file }: { file: any }) => { try { const result = await storage.createFile( appwriteConfig.storageBucketOneId!, ID.unique(), file )
console.log("File created:", result);
return { success: true }
} catch (error) {
console.log("Error creating file:", error);
return { success: false };
}
}`
Recommended threads
- Check User on Server SDK
I have my backend which exposes some endpoints let's say /feed (return a hard coded json as response) I want this to be only accessible to users who are curre...
- Using Appwrite along with Twilio - Deplo...
Stuck on Querying user document...
- [AppwriteException: Network request fail...
When I tried to list the documents from one of the collections, it would show Error: Network request failed. But if I tried others, it would show all of the doc...
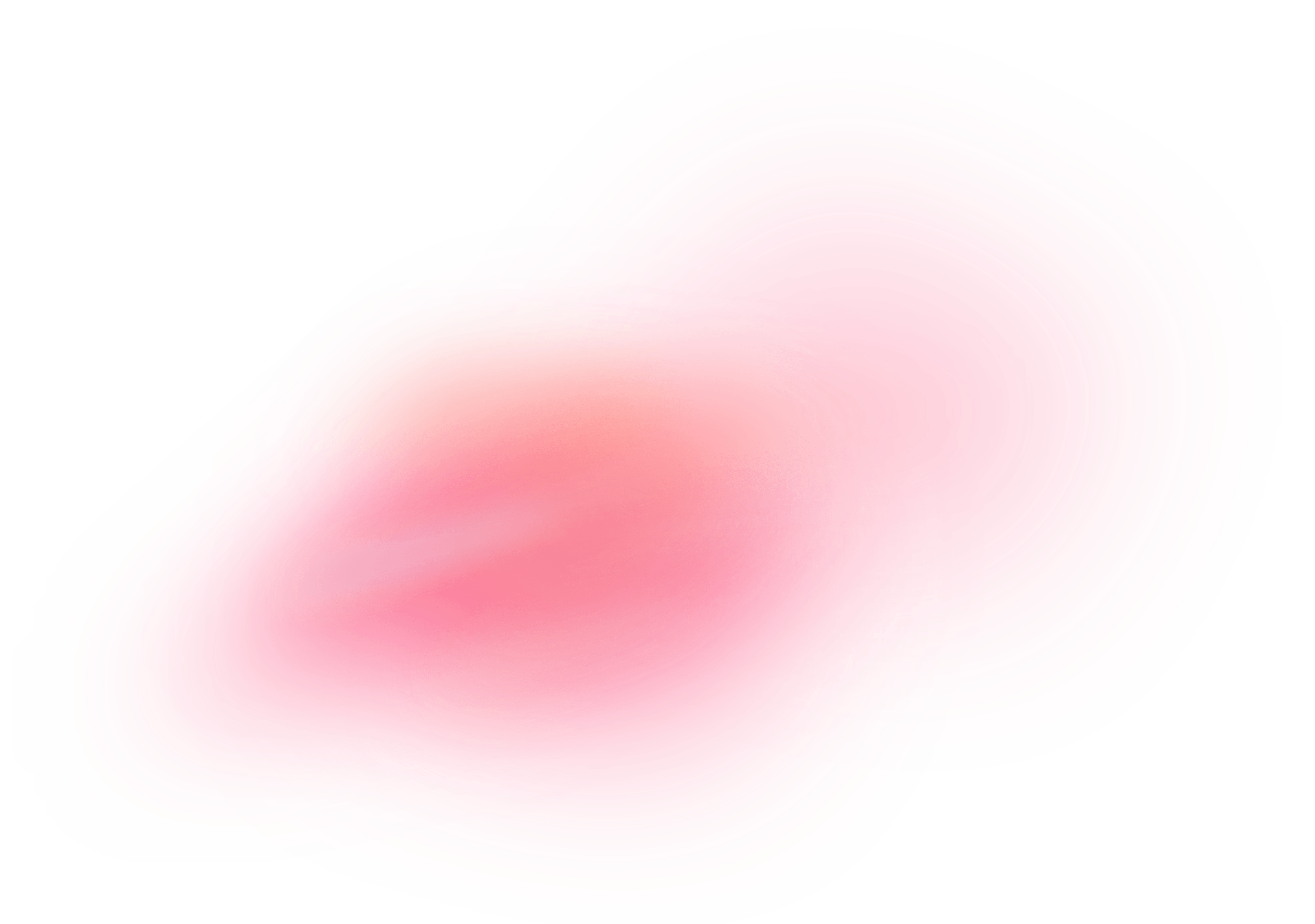