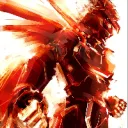
As I understand it appwrite doesn't support transactions. I'm attempting to make a document in three separate collections at the same time when a user registers. If one fails I want all to fail. This is my attempt at that. Is it wrong?
async ({
email,
password,
firstName,
lastName,
buildings,
units,
roles,
}) => {
const { account, databases } = createAdminClient()
const user = await account.create(ID.unique(), email, password)
const userDetails = await databases.createDocument(
import.meta.env.DATABASE_ID,
import.meta.env.USER_DETAILS_ID,
user.$id,
{
firstName: firstName,
lastName: lastName,
},
)
if (!userDetails) {
await account.deleteIdentity(user.$id)
}
const unitUsers = await Promise.all(
buildings.map((building, index) => {
databases.createDocument(
import.meta.env.DATABASE_ID,
import.meta.env.UNIT_USERS_ID,
ID.unique(),
{
unitId: building + units[index],
userId: user.$id,
role: roles[index],
},
)
}),
)
if (!unitUsers) {
await account.deleteIdentity(user.$id)
await databases.deleteDocument(
import.meta.env.DATABASE_ID,
import.meta.env.USER_DETAILS_ID,
userDetails.$id,
)
}
if (user && userDetails && unitUsers) return { registered: true }
},
}),
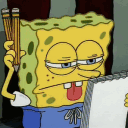
This looks fine but you might want to add some sort of error handling so when something goes wrong it's easy to find what happened, and you can give a useful message to the user.
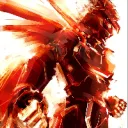
OK I'm going to be testing it very soon!
Recommended threads
- phantom relationships appear on parent c...
i have this bug were my past deleted collection apears as relationship to my parent collection. when i try to delete that relationship from parent it gives me e...
- Attributes Problem - Cloud
I am not able to see the attribute columns and their context on cloud. Can you help?
- Authorization header not working in Appw...
I have an Appwrite function that takes a custom bearer token as authentication. The function works fine locally when I test it with `appwrite run functions`, bu...
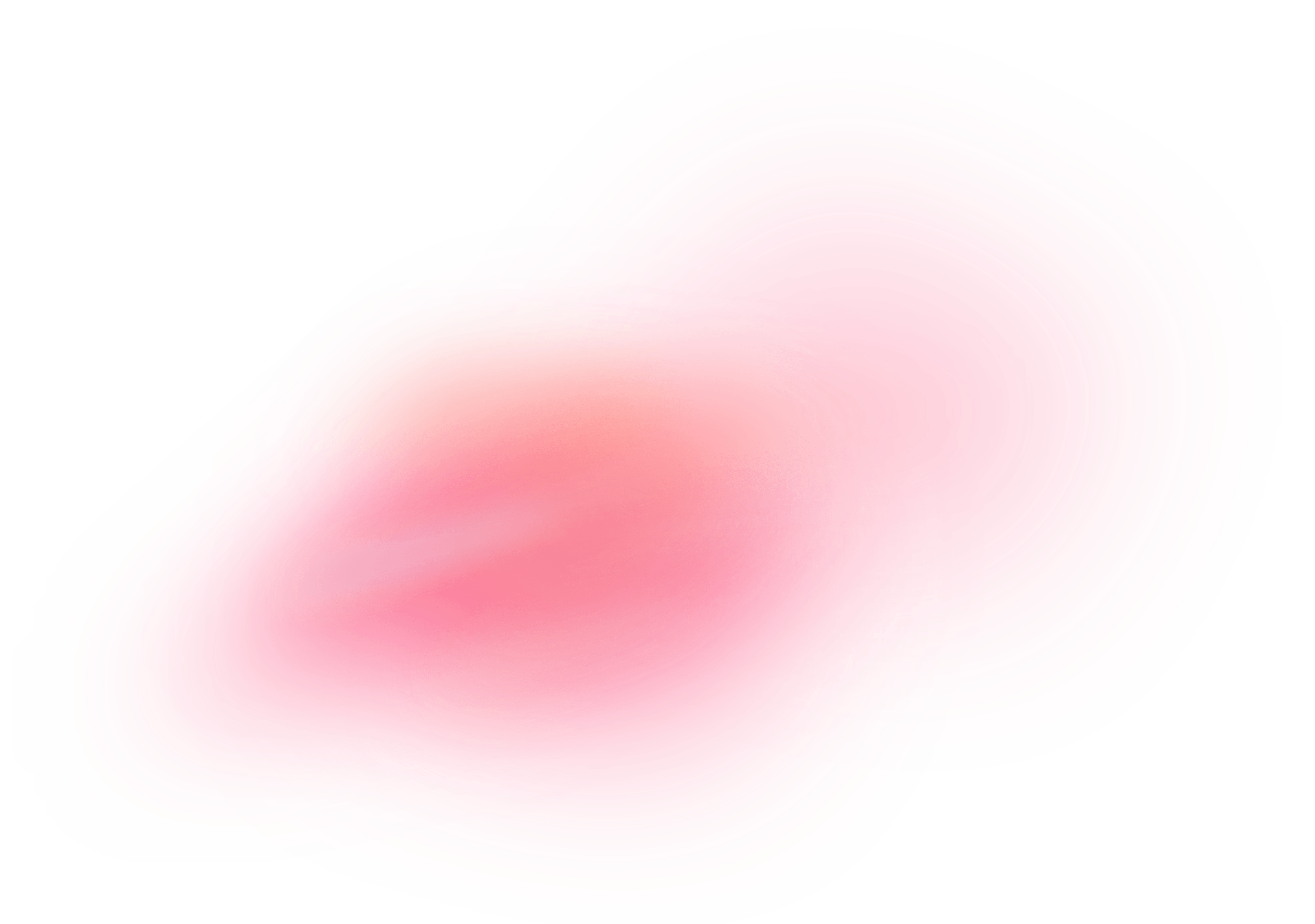