error: 'Document with the requested ID already exists.' even when using ID.unique()
- 0
- Databases
- Cloud

PS: tried to create docs without the relational stuff and the issue still persists..

I am trying to create multiple docs at the same time Like one by one going over the inputs of the users

something like :
for coreData in userUploads{
const coreDoc = await databases.createDocument(
DATABASE_ID,
CORE_COLLECTION_ID,
ID.unique(),
coreData
);
}

and idk why same loop is working for all other collections but when I try to upload in this specific core collection

then only the 1st doc gets generated while all the rest get issues of "document with requested ID already exists"

and the doc that actually exists in the db is with some completely random ID like : 67e95fe700060d076680 and if i clear the db and run again I again get completely unique id like 67e960dc000b6b5b40a2

import { Databases, ID, Query } from 'node-appwrite';
const DATABASE_ID = "sample_db";
const CORE_COLLECTION = "users_core";
const SOCIAL_COLLECTION = "users_social";
const PREFS_COLLECTION = "users_preferences";
// Sample data structure
const sampleUser = {
name: "Alex Smith",
// Social links
social: {
github: "github.com/alex",
},
// Preferences
preferences: {
language: "en"
}
};
async function createUserWithRelations() {
try {
const userUUID = ID.unique();
// Create social links document
const socialDoc = await databases.createDocument(
DATABASE_ID,
SOCIAL_COLLECTION,
ID.unique(),
{
userUUID: userUUID,
github: sampleUser.social.github,
}
);
// Create preferences document
const prefsDoc = await databases.createDocument(
DATABASE_ID,
PREFS_COLLECTION,
ID.unique(),
{
userUUID: userUUID,
language: sampleUser.preferences.language
}
);
// Create core user document with references
const coreDoc = await databases.createDocument( // <--- Error here
DATABASE_ID,
CORE_COLLECTION,
ID.unique(),
{
userUUID: userUUID,
name: sampleUser.name,
socialId: socialDoc.$id,
preferencesId: prefsDoc.$id
}
);
return {
core: coreDoc,
social: socialDoc,
preferences: prefsDoc
};
} catch (error) {
console.error("Error creating user:", error);
throw error;
}
}

this is a pseudo code of what I'm trying to do /how im working with my relational db

also tried the nested method , something like this :
async function createDocument() {
try {
// Single document creation with nested data
const doc = await databases.createDocument(
DATABASE_ID,
MAIN_COLLECTION,
ID.unique(),
{
name: sampleData.name,
email: sampleData.email,
// Directly embedding related data as nested objects
socialMedia: sampleData.social,
preferences: sampleData.preferences
}
);
return doc;
} catch (error) {
console.error("Error creating document:", error);
throw error;
}
}
and even this is not working

response: '{"message":"Document with the requested ID already exists. Try again with a different ID or use ID.unique() to generate a unique ID.","code":409,"type":"document_already_exists","version":"1.6.2"}'
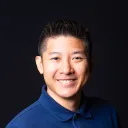
Do you have a unique index on any of those attributes?

initially I was not having a unique index as I thought the appwrite $id does a unique index automatically but now I just added a unique index as the UUID of the user and still the issue persists
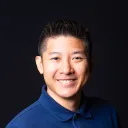
So you didn't have any unique indexes before?
Does this collection have any relationship attributes? do you have unique indexes on any of your collections?
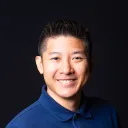
Btw, relationship attributes are experimental and should be used with caution as they can lead to performance problems

yes

I have a unique Index called user UUID in all of the 3 collections
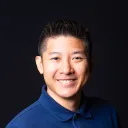
There were two questions

just a sec answering all

- yes , I didnt have any unique indexes before i did tried to create indexes when setting up the db but at that time the cloud was having the issue with the databases so I was unable to create the unique indexes at that time
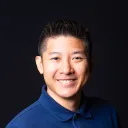
Perhaps a new document is trying to be created instead of linking the existing document which is why you see the duplicate error
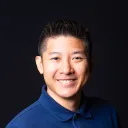
Can you share your exact code?

- about the unique indexes in collections I have a unique index of UUID in each of the collection
also really sorry for my dumbness , its my first time working with appwrite db 😅

thats honestly what I also thought but then I tried to use nexted way of creating the document and still facing the same issue

can I just send it over on dms?

its almost 80% identical , only the variable names are different
Recommended threads
- my database attribute stuck in processin...
when i created attributes in collection 3 of those attributes become "processing", and they are not updating, the worst thing is that i cant even delete them s...
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
- Forever Processing Issue
I encountered an issue when creating attributes in the collections . if you create an attribute of type string for example and choose a size of 200 or 250 or a...
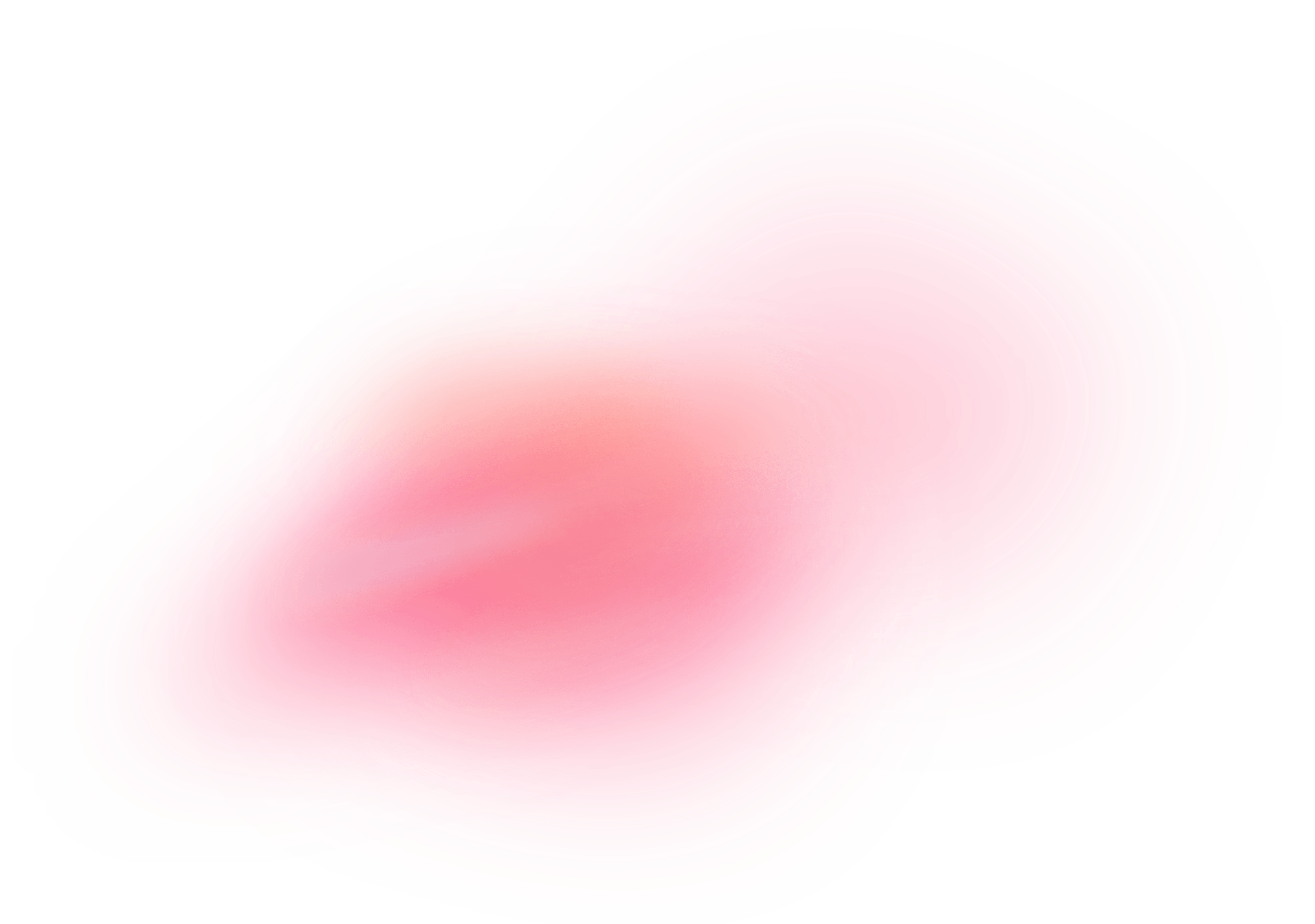