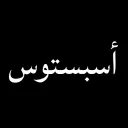
I am trying to implement oauth2 with Google, but it keeps giving this weird 404 error.
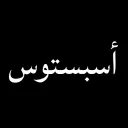
// index.tsx
// ...
<Button
className="bg-white flex flex-row gap-2"
variant="outline"
size="lg"
onTouchEnd={() => {
auth.signIn.google();
}}
>
<AntDesign name="google" size={24} color="black" />
<Label className="text-black">
Sign in with Google
</Label>
</Button>
// ...
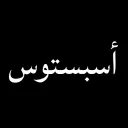
// AuthContext.tsx
import { useContext, createContext, useState, ReactNode } from "react";
import { makeRedirectUri } from "expo-auth-session";
import * as WebBrowser from "expo-web-browser";
import { account } from "@/lib/appwrite";
import { OAuthProvider, Models } from "react-native-appwrite";
import { Text, SafeAreaView } from "react-native";
import { AuthContextType } from "~/types/AuthContextType";
const AuthContext = createContext<AuthContextType>({
session: null,
user: null,
signIn: {
google: () => {},
},
signOut: () => {},
});
const AuthProvider = ({ children }: { children: ReactNode }) => {
const [loading, setLoading] = useState(false);
const [session, setSession] = useState<Models.Session | null>(null);
const [user, setUser] = useState<Models.Preferences | null>(null);
const signIn = {
google: async () => {
// Create a deep link that works across Expo environments
// Ensure localhost is used for the hostname to validation error for success/failure URLs
const deepLink = new URL(
makeRedirectUri({ preferLocalhost: true })
);
if (!deepLink.hostname) {
deepLink.hostname = "localhost";
}
const scheme = `${deepLink.protocol}//`; // e.g. 'exp://' or 'playground://'
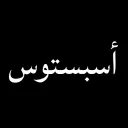
// Explicitly URL-encode the redirect URLs
const successRedirect = encodeURIComponent(deepLink.toString());
const failureRedirect = encodeURIComponent(deepLink.toString() + "/fail");
// Start OAuth flow
const loginUrl = await account.createOAuth2Session(
OAuthProvider.Google,
successRedirect, // Use the encoded URL
failureRedirect // Use the encoded URL
);
console.log("Scheme: ", scheme);
console.log("Deep Link: ", deepLink);
console.log("Success Redirect: ", successRedirect);
console.log("Failure Redirect: ", failureRedirect);
console.log("Login URL: ", loginUrl);
// Open loginUrl and listen for the scheme redirect
const result = await WebBrowser.openAuthSessionAsync(
`${loginUrl}`,
scheme
);
console.log("Result: ", result);
// Extract credentials from OAuth redirect URL
if('url' in result) {
const url = new URL(result.url);
const secret = url.searchParams.get("secret");
const userId = url.searchParams.get("userId");
if(secret && userId) {
let session = await account.createSession(userId, secret);
setSession(session);
let user = await account.get();
setUser(user);
}
console.log(result, url, secret, userId, session);
} else {
throw new Error("OAuth login failed.");
}
},
};
const signOut = async (): Promise<boolean> => {
if(session) {
await account.deleteSession(session.$id);
setSession(null);
} else {
setSession(null);
}
return true;
};
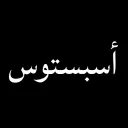
const contextData: AuthContextType = { session, user, signIn, signOut };
return (
<AuthContext.Provider value={contextData}>
{loading ? (
<SafeAreaView>
<Text>Loading...</Text>
</SafeAreaView>
) : (
children
)}
</AuthContext.Provider>
);
};
const useAuth = () => {
return useContext(AuthContext);
};
export { useAuth, AuthContext, AuthProvider };
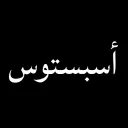
heres the console logs
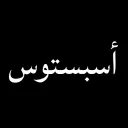
I've tried account#createOAuth2Token and that doesnt change anything
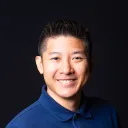
Weird. What URL are you ending up at?
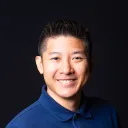
OAuth2 with React Native is not fully supported. The GitHub comment explains the current state: https://github.com/appwrite/sdk-for-react-native/issues/34#issuecomment-2654940715
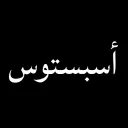
but since oauth2 isnt support ig that doesnt matter - do you know if magic urls are supported either? im guessing the deeplinking also applies to that
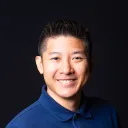
Correct, deep linking is the problem so anything related to that will be an issue
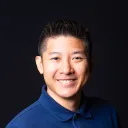
Unless you use universal links
Recommended threads
- Dumb question, api key for app? Protect ...
I have my project set up, and I'm developing with Flutter. My app does not require users to log in to retrieve some general project data, but authentication is ...
- Appwrite SDK react native 7.0.1
Hi I'm currently making an app, using react native and stumbled across a major problem regarding version 7.0.1 where they removed async functions. I reverted to...
- Account Deletion is not working
Hey There, i am trying to trigger a function with a swift ui button. The function ist to delete the currently active user with the userID, so that the account i...
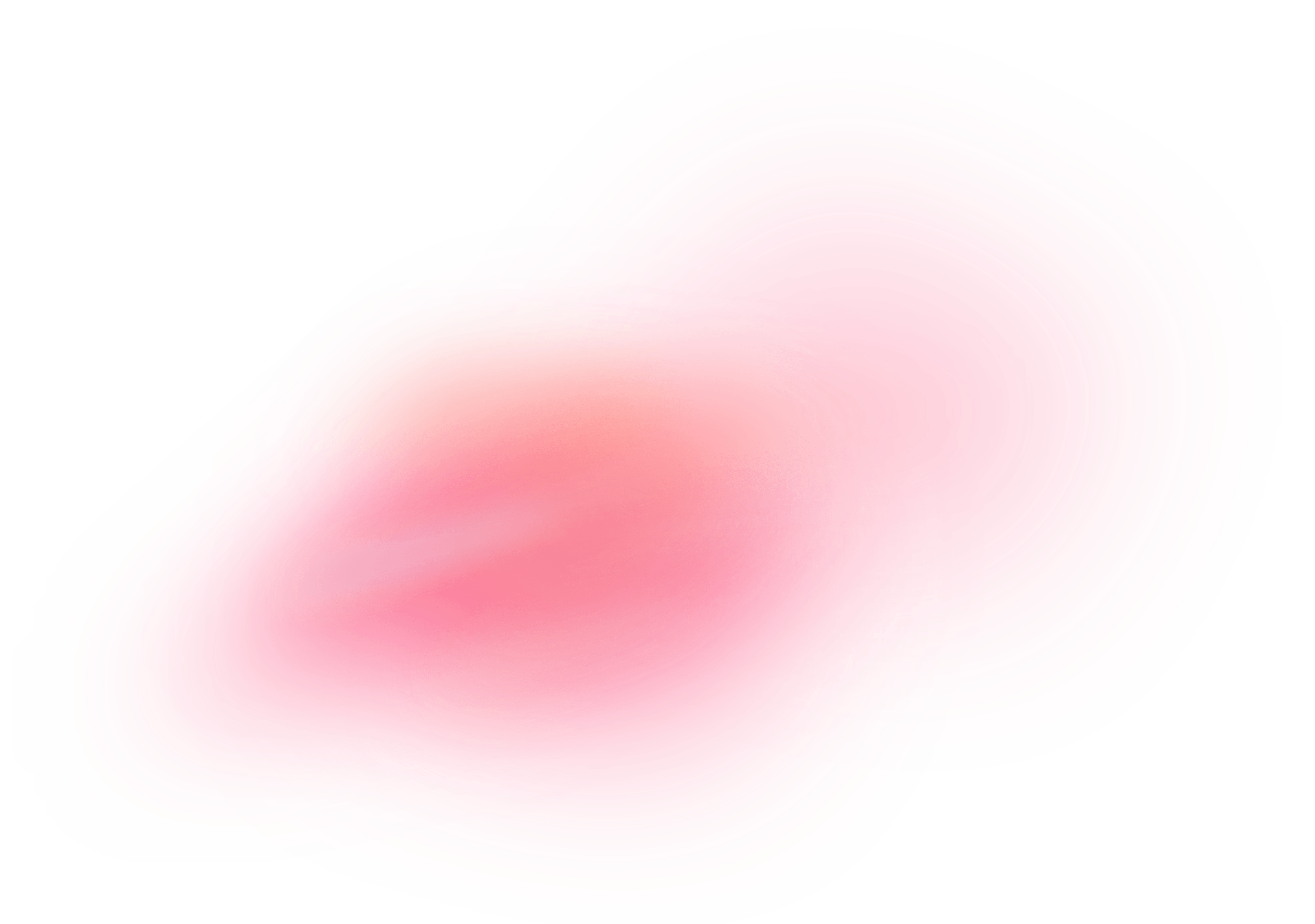