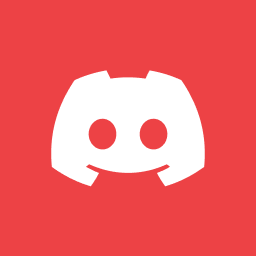
TypeScript
const { Client, Account } = require('appwrite');
const client = new Client();
client
.setEndpoint('xxxxxxxxxxxxxxxxxxxxx')
.setProject('xxxxxxxxxxxxxxxxx');
const authenticateAndGenerateJWT = async (email, password) => {
try {
const account = new Account(client);
// Step 1: Authenticate the user and create a session
const session = await account.createEmailPasswordSession(email, password);
console.log('Session created successfully:', session);
// Step 2: Set the session token for subsequent requests
client.setSession(session); // Use the session ID as the token
// Step 3: Generate JWT
const jwt = await account.createJWT();
console.log('JWT created successfully:', jwt.jwt);
return jwt.jwt; // Return the generated JWT
} catch (error) {
console.error('Error:', error.message);
throw new Error('Failed to authenticate or create JWT.');
}
};
error
TypeScript
Error: User (role: guests) missing scope (account)
Error: Failed to authenticate or create JWT.
TL;DR
To generate a JWT after authentication, developers should ensure the user has the necessary scope. In this case, the error 'User (role: guests) missing scope (account)' indicates a lack of permissions. Make sure the guest role has the 'account' scope enabled in the Appwrite console. Recommended threads
- I'm developing a CLI application using N...
I'm developing a CLI application using Node.js and the `node-appwrite` package, but I'm encountering an error ```ts import { Client, Account, ID } from 'node-a...
- Invalid Query Go SDK 0.2.0
Hello everyone, as you can see my log, the Go SDK somehow always return Error, but when i'm using client sdk for React it's fine
- custom domain not verifying
i am adding my custom domain(purchased from godaddy) to appwrite but event after i added DNS records in my domain it is not verifying it on appwrite. below are...
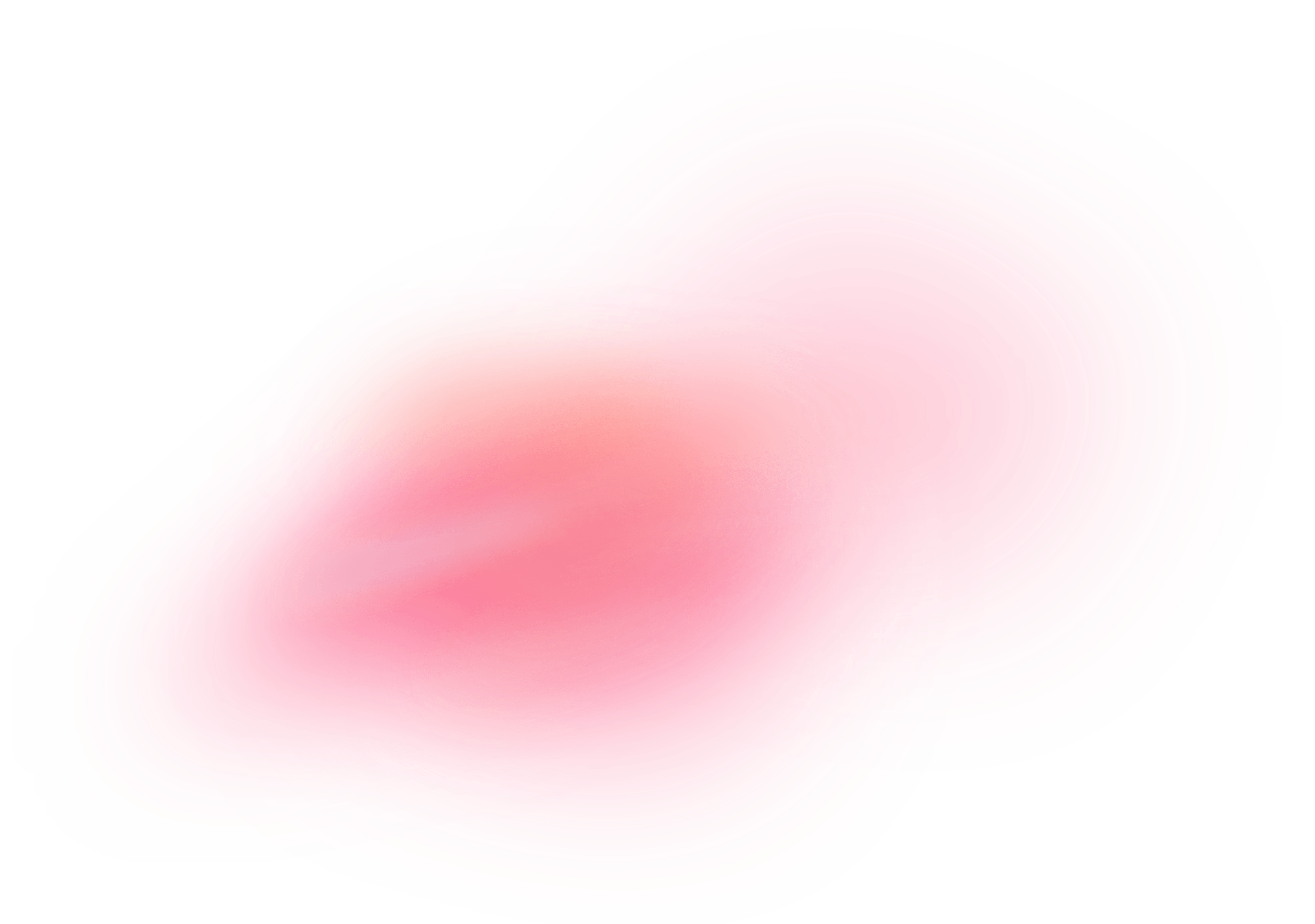