
Me and my team are having some issues with relations in .NET SDK. We have to first create an element and then update it with the relation of the object before it works, otherwise it gives permission error (yes i've set the right permissions). Any idea when a new SDK or a new version of the selfhosted appwrite docker image will release?
We're depeding on Appwrite for a project and it would be nice if relations worked as expected. I've seen a lot of progression on their Github but no new release? PS yes i know it's in beta but this seems like a basic requirement to us...

If needed i can privately share the project files
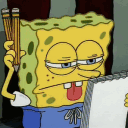
So when you try to create a new record with a relationship to another new record it fails saying you don't have the perms. But when you create each separately then update the parent record to relate to the child it works fine?

Yes exactly! Can you help me?
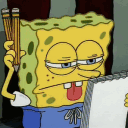
Can you successfully create the both items in the SDK?
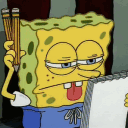
in seperate calls?
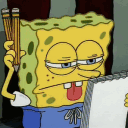
Can you send screenshot of the permissions you have setup for both collections please?

Yes in separate calls it works fine

all permissions are set like this
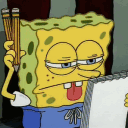
Do you have more than one relationship? Can you send the code just for the create document method, leaving out tokens

So just for explanation i've set up a abstract base class which is a wrapper around the appwrite SDK, just for easy creating of object inside the project. Which is the following method:

public async Task<T> Create(T item)
{
try
{
var result = await databases.CreateDocument(DatabaseId, _collectionId,
item.GetId() ?? throw new InvalidOperationException(), item.ToJson(true));
return JsonSerializableBase.FromJson<T>(result.Data.ToJson()) ?? throw new InvalidOperationException();
}
catch (Exception e)
{
throw new Exception($"Error in Create: {e.Message}");
}
}

Then for example in the SeedDb class, i use it as follows:

var createdActivity = await unitOfWork.ActivityService.Create(
new Activity(100.0, DateTime.Now, "Lunch", null)
);
var updatedActivity = await unitOfWork.ActivityService.Update(
createdActivity.Id,
new Activity(100.0, DateTime.Now, "Lunch", createdUsers.ToList())
);
var createdGroup = await unitOfWork.GroupService.Create(new Group("Dev Team"));
await unitOfWork.GroupService.Update(
createdGroup.Id,
new Group("Dev Team", createdUsers.ToList(), new List<Activity> { updatedActivity })
);

For example my model which i have here is serialized as following:

public class Activity : JsonSerializableBase
{
public Activity(double price, DateTime dateTime, string activityName, List<UserInfo>? userInfos)
{
Price = price;
DateTime = dateTime;
ActivityName = activityName;
UserInfos = userInfos;
Id = string.IsNullOrEmpty(Id) ? ID.Unique() : Id;
}
public Activity(double price, DateTime dateTime, string activityName)
{
Price = price;
DateTime = dateTime;
ActivityName = activityName;
Id = string.IsNullOrEmpty(Id) ? ID.Unique() : Id;
}
public Activity()
{
Id = ID.Unique();
}
[JsonProperty("Price")]
public double Price { get; set; }
[JsonProperty("DateTime")]
public DateTime DateTime { get; set; }
[JsonProperty("ActivityName")]
public string ActivityName { get; set; }
[JsonProperty("userInfo")]
public List<UserInfo>? UserInfos { get; set; }
}

Hope that clarifies how i use the sdk in code 🫡

And to fully answer your question i have multiple relations as you see in code
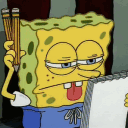
And you're sure every related item has the permissions set on them? This wouldn't really be an issue with the SDK as that is just a wrapper for the Appwrite REST API.

Yes correct but at times the issue is not the permission but it recreates an item that already exists when creating a relation. Altough the ids are the same
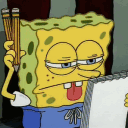
Hmm, I'm not entirely sure what the issue could be, and I know this does not help your situation right now, but relationships are in beta and pretty inefficient and have issues with them. I would realistically suggest migrating away from using them until they get the revamp they need.
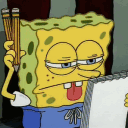
Right now, the suggestion for related data is to create a foreign key attribute and utilize that to fetch/update data when necessary. With the next release (1.7) database transactions are coming which should help you when you have, for example, a table of data and you need to fetch a child item for each record.
Recommended threads
- Properly contained appwrite main app can...
Hello! We tried to reinstall our main self-hosted appwrite with a new method but the main app 2 mins after launch throw this error: ```2025/06/22 16:16:14 s...
- Broken message
https://github.com/appwrite/appwrite/issues/10081 I just realized that I can just build appwrite myself, was this bug fixed in latest dev release?
- 404 errors after 7 Days
Local hosted Appwrite via docker. Last version and current version. After exactly 7 days Appwrite stops working. I get 404 route not found, cannot access anyth...
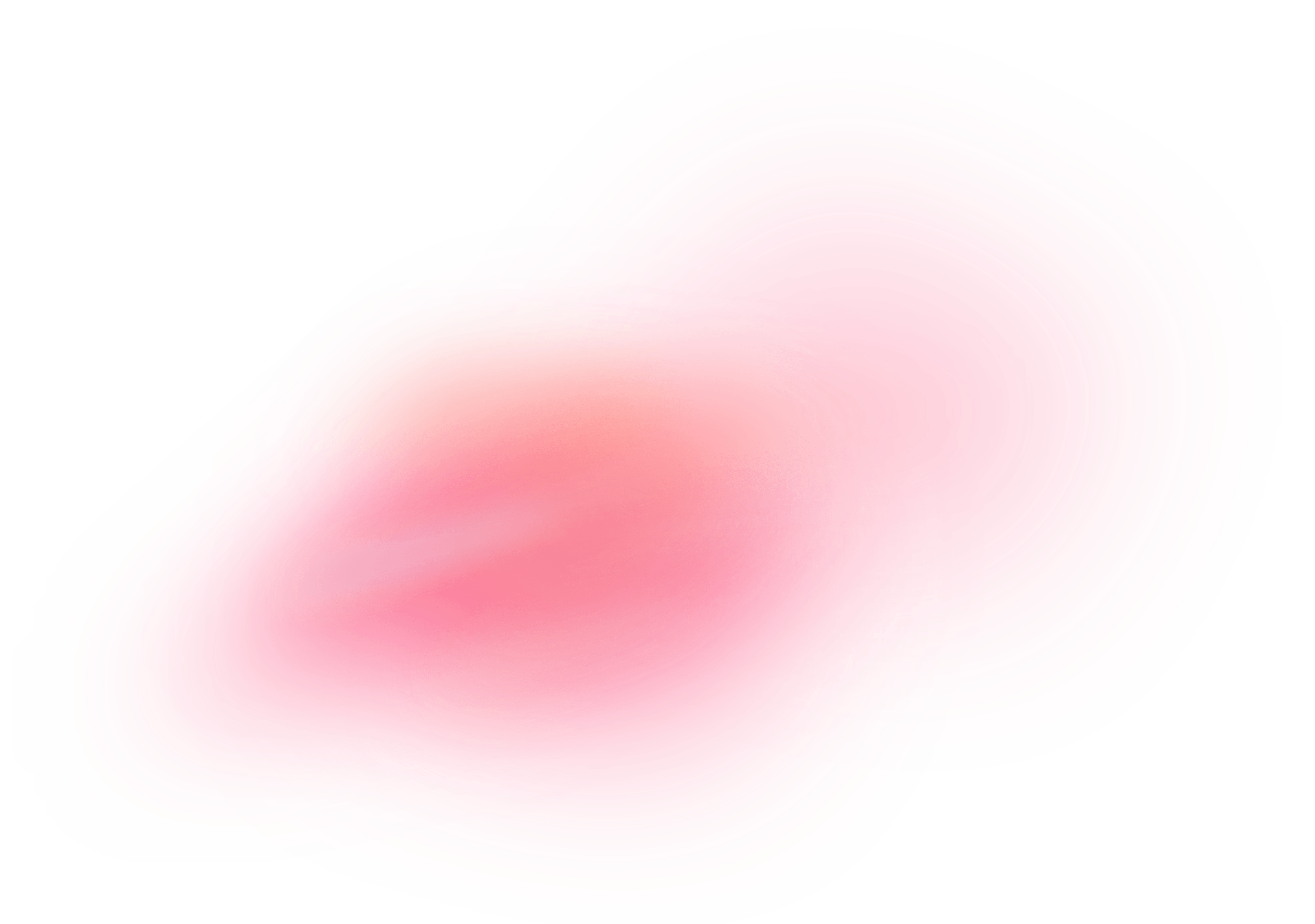