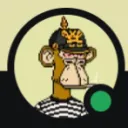
Hi there, I am getting a weird error where my file creation is timing out. Maybe I have the wrong syntax, but I tried to follow the documentation.
// Download the image directly as a stream
const imageResponse = await axios.get(output, { responseType: "arraybuffer", timeout: 10000}); // Use arraybuffer for compatibility
// Convert the array buffer to a Buffer
const imageBuffer = Buffer.from(imageResponse.data);
// Upload the image to Appwrite storage directly
const createFile = await storage.createFile(
config.bucketId, // Replace with your Appwrite storage bucket ID
ID.unique(), // Generate a unique file ID
imageBuffer, // Pass the stream directly
["user:" + account_id] // Set user-specific permissions (read/write access)
);
// Save metadata in Appwrite database
const metadata = {
account_id: account_id, // The user's account ID
file_id: createFile.$id, // File ID in Appwrite
userPrompt: additionalUserPrompt, // Store the prompt for reference
};
// Create MetaData entry
const createReplicateMetadataDocument = await databases.createDocument(
config.databaseId,
config.replicateMetadataId, // Replace with your Appwrite database collection ID
ID.unique(), // Generate a unique document ID
metadata
);
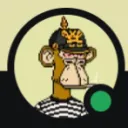
and here is the error from the function execution:
Error calling Replicate: TypeError: Cannot read properties of undefined (reading 'Symbol(Symbol.asyncIterator)')
at Storage.createFile (/usr/local/server/src/function/node_modules/node-appwrite/lib/services/storage.js:404:40)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async Module.default (file:///usr/local/server/src/function/src/main.js:76:24)
at async execute (/usr/local/server/src/server.js:208:16)
at async action (/usr/local/server/src/server.js:225:7)
at async /usr/local/server/src/server.js:14:5
The error is happening around 14s so it isn't the 30s timeout causing it. Anybody have ideas? Thanks.
Recommended threads
- Heads up: billing page errors
In my appwrite dashboard, I tried to open the billing page but it shows nothing, opened the browser dev tools and saw some errors, tried chrome (win 10, android...
- Document Level Permission
I want to know if a user who has created a document in a collection can assign document-level permission for another user for that same document?
- Implementing Google OAuth2.0 in React Na...
Hey , I want to implement Google OAuth 2.0 in my React Native Expo project but not getting how to do it. I have implemented in React project and in that project...
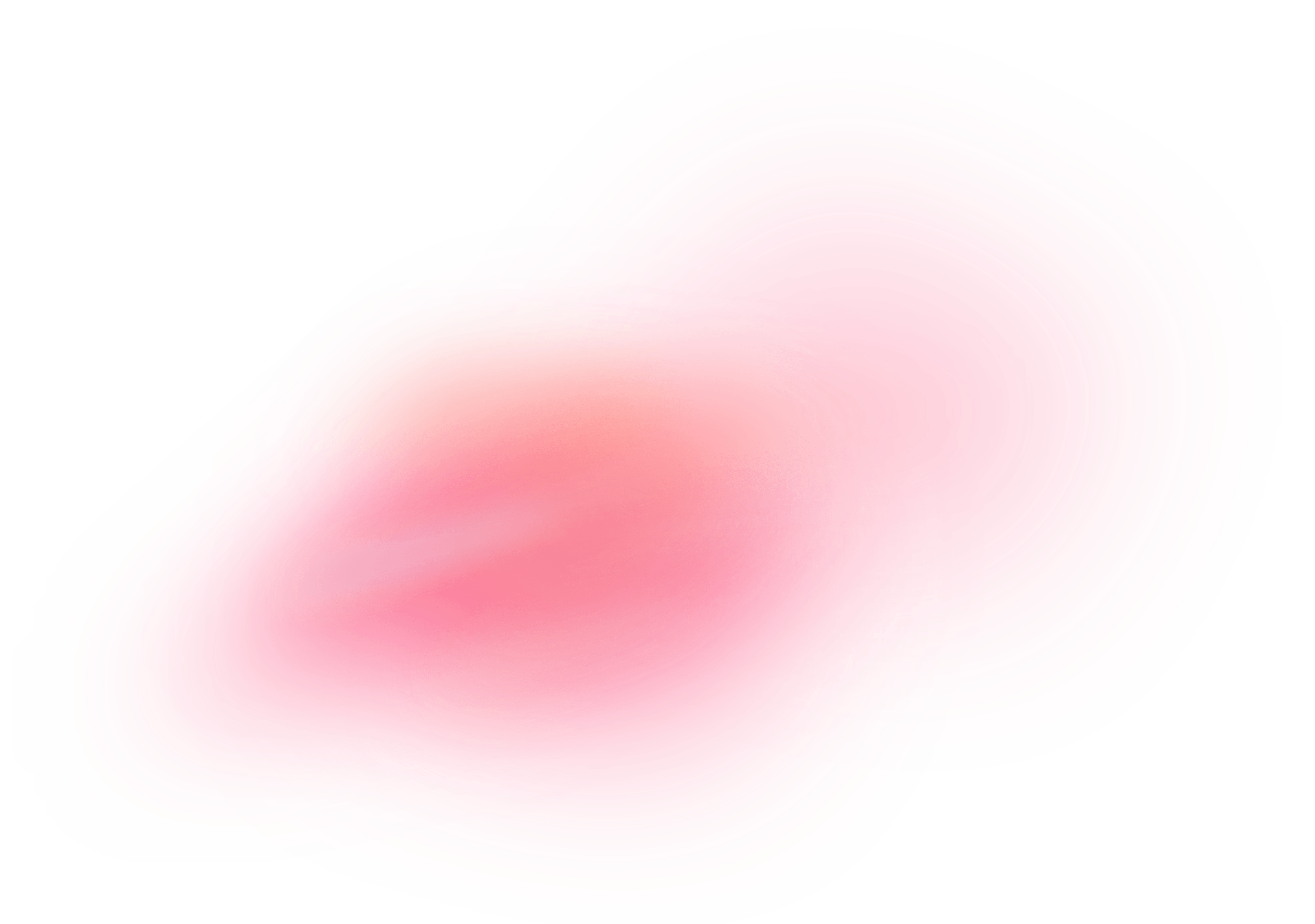