
Hi all, I am trying to create a function which connects to my db but I am getting a database not found error. I have put the exact db & collection IDs but still there is error. I have tried sending a simple text response & that worked, means path and everything is set correctly. I have also given correct permissions in db and collections. I have also selected All in the Scopes of my Function. But when I am trying to do a listDocuments call to my db, it is saying db not found.
I have used this tutorial for this https://www.youtube.com/watch?v=PmEIu-qrcac&list=PL-nc7zI7zjsbGzYBiG_V3tEFpmlzzbJgC&index=4
Here is my code. I tried replacing the IDs of the project, db and collections with the exact id, but still it is an issue. The star mark is where I put my IDs. import { Client, Databases } from "node-appwrite";
export default async ({ req, res, log, error }) => {
const client = new Client()
client
.setEndpoint('https://cloud.appwrite.io/v1')
.setProject('project id here ***')
const db = new Databases(client)
if (req.method == 'GET') {
const response = await db.listDocuments(
'db id here ***',
'collcetions id here ***'
)
return res.json(response.documents)
}
return res.send("Shlok, tesing if user delete worked")
}
Below is my error -
Error: Database not found at Client.call (/usr/local/server/src/function/node_modules/node-appwrite/lib/client.js:168:31) at process.processTicksAndRejections (node:internal/process/task_queues:95:5) at async Databases.listDocuments (/usr/local/server/src/function/node_modules/node-appwrite/lib/services/databases.js:905:16) at async Module.default (file:///usr/local/server/src/function/functions/main.js:12:26) at async execute (/usr/local/server/src/server.js:208:16) at async action (/usr/local/server/src/server.js:225:7) at async /usr/local/server/src/server.js:14:5
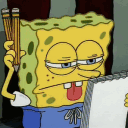
Is your project ID correct?

yes
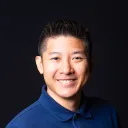
FYI, it's best to wrap code in backticks to format a bit nicer. You can use 1 backtick for inline code (https://www.markdownguide.org/basic-syntax/#code) and 3 backticks for multiline code (https://www.markdownguide.org/extended-syntax/#syntax-highlighting).

Thanks Steven, I will keep that in mind next time.
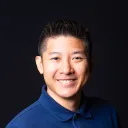
what are the permissions on your collection?

all ticked
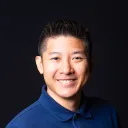
double check your project id, database id, and collection id are correct. If you're still having trouble, please share exactly what you're using in your code and the URL of this collection settings page on the Console

This is the structure of my project - attached in image. There is an .env file where I create all the variables for Appwrite IDs.
Example:
VITE_PROJECT_ID=671f17fb0030e3b17ebc
Now I have configured all three environment variables - Project id, database id & collection id in console -> Function Environment variables.
Here is the collection settings page on the Console https://cloud.appwrite.io/console/project-671f17fb0030e3b17ebc/databases/database-671f2f4f00014e62eae3/collection-671f3276001caa90944d/settings
Here are the contents of my main.js file
import { Client, Databases } from "node-appwrite";
const PROJECT_ID = process.env.VITE_PROJECT_ID
const DATABASE_ID = process.env.VITE_DATABASE_ID
const COLLECTION_ID_ALLUSERS = process.env.VITE_COLLECTION_ID_ALLUSERS
export default async ({ req, res, log, error }) => {
const client = new Client()
client
.setEndpoint('https://cloud.appwrite.io/v1')
.setProject(PROJECT_ID)
const db = new Databases(client)
if (req.method == 'GET') {
const response = await db.listDocuments(
DATABASE_ID,
COLLECTION_ID_ALLUSERS
)
return res.json(response.documents)
}
return res.send("Shlok, tesing if user delete worked")
}
Latest execution result
Error: Database not found
at Client.call (/usr/local/server/src/function/node_modules/node-appwrite/lib/client.js:168:31)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async Databases.listDocuments (/usr/local/server/src/function/node_modules/node-appwrite/lib/services/databases.js:905:16)
at async Module.default (file:///usr/local/server/src/function/functions/main.js:17:26)
at async execute (/usr/local/server/src/server.js:208:16)
at async action (/usr/local/server/src/server.js:225:7)
at async /usr/local/server/src/server.js:14:5
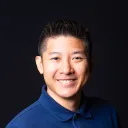
const PROJECT_ID = process.env.VITE_PROJECT_ID
const DATABASE_ID = process.env.VITE_DATABASE_ID
const COLLECTION_ID_ALLUSERS = process.env.VITE_COLLECTION_ID_ALLUSERS
Would you please log these variables to verify the correct values are being used?
Recommended threads
- Is my approach for deleting registered u...
A few weeks ago, I was advised not to use the registered users' id in my web app. Instead, I store the publicly viewable information such as username and email ...
- ❗[Help] Function stuck in "waiting" stat...
Hi Appwrite team 👋 I'm trying to contribute to Appwrite and followed the official setup instructions from the CONTRIBUTING.md guide to run the platform locall...
- Stuck in "deleting"
my parent element have relationship that doesnt exist and its stuck in "deleting", i cant delete it gives me error: Collection with the requested ID could not b...
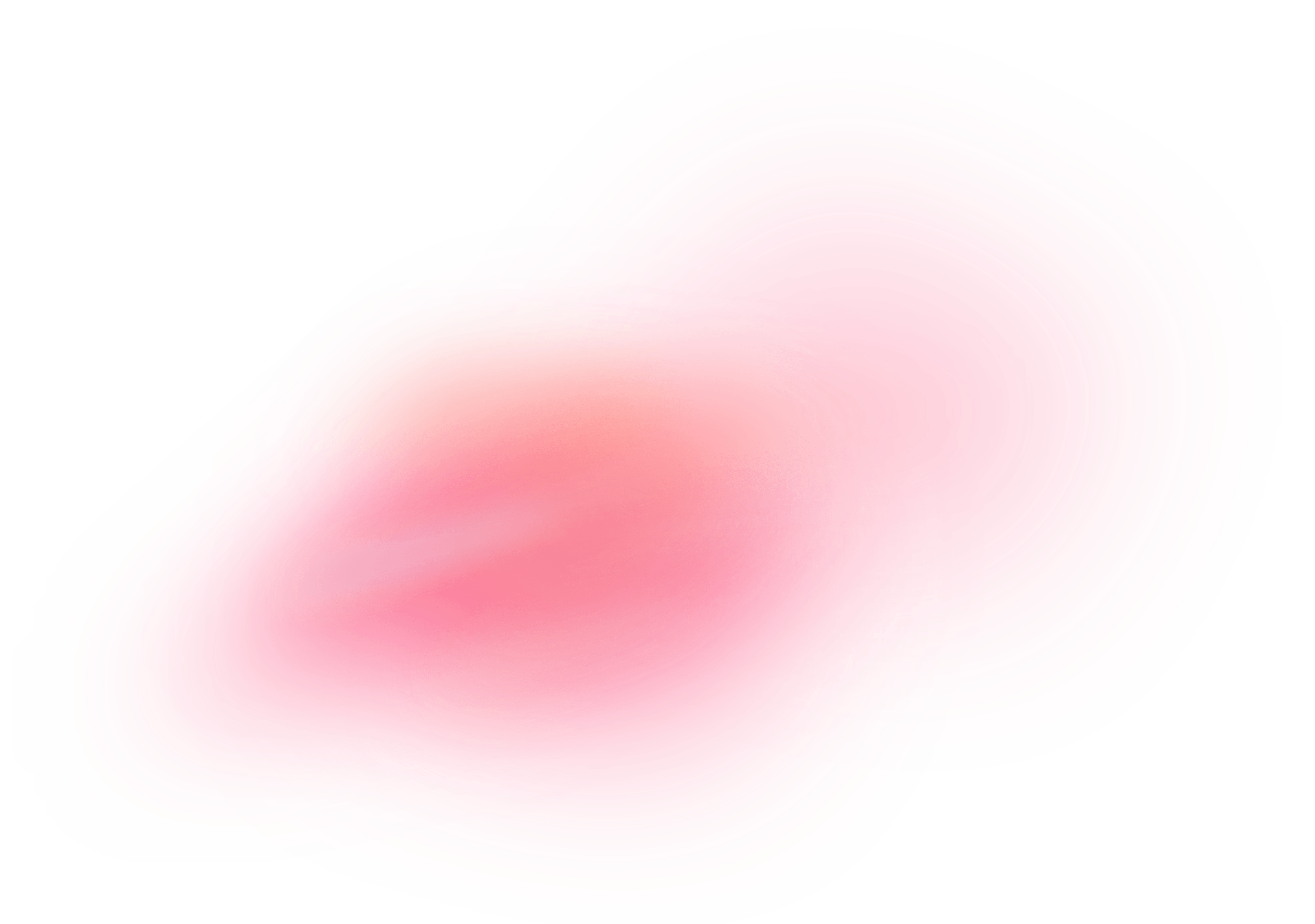