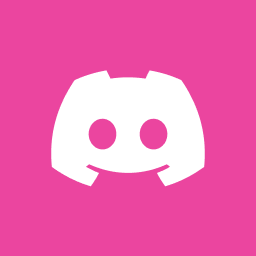
Hey Appwrite team!
I develop a Python function. it first get data from user collection, decrease their credits and try to update it. Apparently it should work fine but unexpectedly it is failing in updating the document.
It throws this error:
[ERROR] The document data and permissions are missing. You must provide either document data or permissions to be updated.
##Code
from appwrite.client import Client
from appwrite.services.databases import Databases
def main(context):
#get required values from headers
user_id = context.req.headers['x-appwrite-user-id']
api_key=context.req.headers['x-appwrite-key']
#get required values from environment variables
endpoint=os.environ['APPWRITE_FUNCTION_API_ENDPOINT']
project_id=os.environ['APPWRITE_FUNCTION_PROJECT_ID']
db_id=os.environ['DB_ID']
user_collection_id=os.environ['USER_COLLECTION_ID']
#setup appwrite client
client = Client()
client.set_endpoint(endpoint)
client.set_project(project_id)
client.set_key(api_key)
database = Databases(client)
#get user details
try:
user = database.get_document(db_id, user_collection_id, user_id)
context.log(f'User details: {user}')
except Exception as e:
context.log(f'Error getting user details: {e}')
#reduce user credits by 1
updated_credits = user['credits'] - 1
context.log(f'Updated credits: {updated_credits}')
try:
#save updated credits to user document
save = database.update_document(
database_id=db_id,
collection_id=user_collection_id,
document_id= user_id,
data={'credits': updated_credits}
)
context.log(f'saved: {save}')
return context.res.json({'ok': True,'credits': updated_credits},200)
except Exception as e:
context.log(f'Error saving updated credits: {e}')
return context.res.json({'ok': False,'error': str(e)},400)
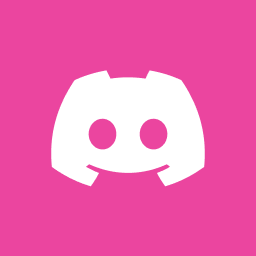
💻 My system
Appwrite 1.6.0 Linux server with Ubuntu 22.04 python runtime 3.11 using dynamic API from functions that has all database access.
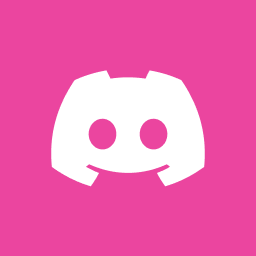
@D5 This is from appwrite python sdk. Not my custom function.
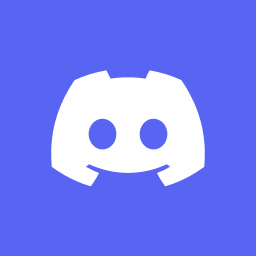
Oh, didn't knew that it had that formatting
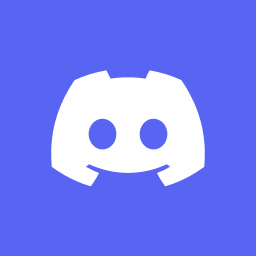
If so you need to specify the document ID, collection ID, apart from it content:
databases.update_document(
database_id = '<DATABASE_ID>',
collection_id = '<COLLECTION_ID>',
document_id = '<DOCUMENT_ID>',
data = {}, # optional
permissions = ["read("any")"] # optional
)
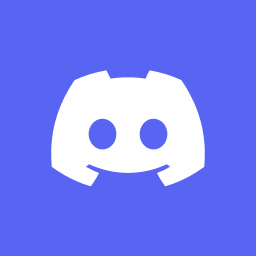
You have some required field in the data, so probably you need to specify that too
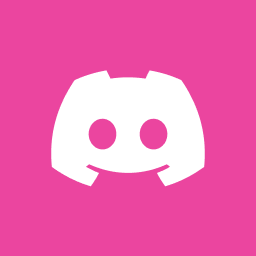
If you look closely, i already have specified all of the required fields as they need to be.
I tried this same setup with my flutter app and from client side we can easily update the document. It only fails when using in function with python sdk.
I think its a bug inside the sdk.
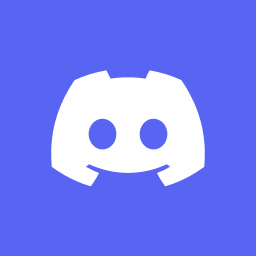
Do you have set an API key with the needed scopes in order to allow updating documents?
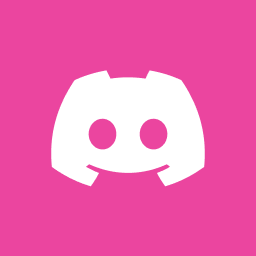
Yes my api has all database access types.
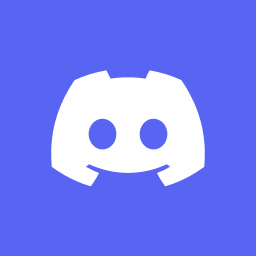
Not sure then. What appwrite version, runtime version are you using?
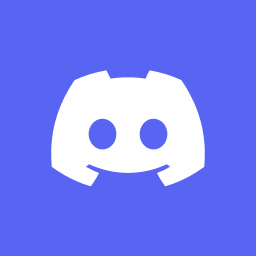
Are you using appwrite self-hosted?
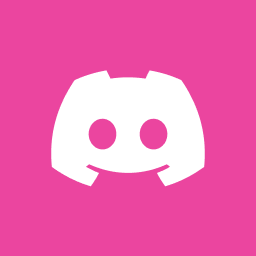
Appwrite: 1.6.0 Runtime: Python-3.11 Yes its a self hosted instance.
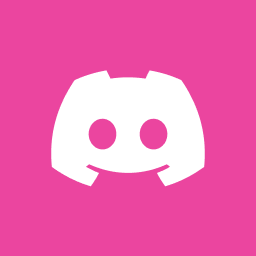
Anyone from core team members, do you guys aware of this problem?
Is there any workaround?
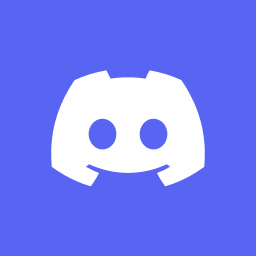
From what I understand, the error is being thrown because there is no data being specified to it. Are you 100% sure that the updated_credits is not empty? Do you can try to log that variable?
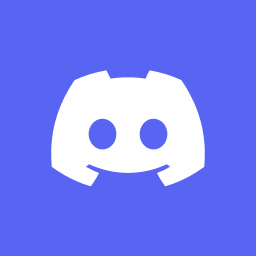
Also, what's your python SDK version? Do you can try the latest?
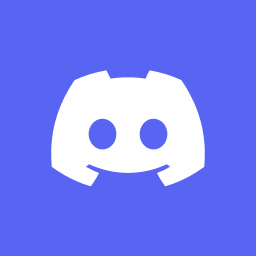
And also, do you can show the logs for the appwrite main container?
You can get them by running this:
docker compose logs appwrite
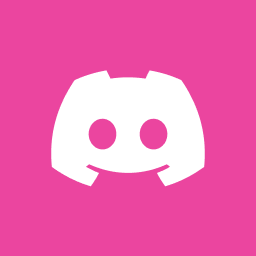
I have provided data by creating a dictionary as well. but he results are always same.
Here are my logs:
User details: {'credits': 4, 'name': 'John Doe', '$id': '6709f2d000358e2c41f7', '$createdAt': '2024-10-12T04:41:40.883+00:00', '$updatedAt': '2024-10-12T07:37:06.027+00:00', '$permissions': ['read("user:6709f2d000358e2c41f7")', 'update("user:6709f2d000358e2c41f7")'], '$databaseId': '6709f300000a8e07a263', '$collectionId': '6709fdd50029a53b91f3'}
Updated credits: 3
Error saving updated credits: The document data and permissions are missing. You must provide either document data or permissions to be updated.
I also have attached my appwrite logs
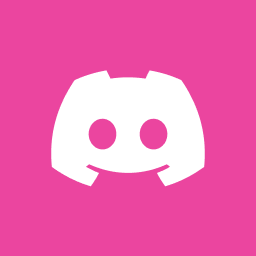
and I am using latest python sdk of appwrite.
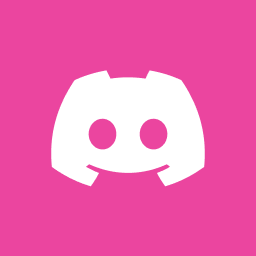
I tried the same setup usng node and surprisingly it worked as expected: Here is my code snippts:
import { Client, Databases } from 'node-appwrite';
// This Appwrite function will be executed every time your function is triggered
export default async ({ req, res, log, error }) => {
//get required values from headers
const userId =
'6709f2d000358e2c41f7'; //context.req.headers['x-appwrite-user-id'];
const apiKey = req.headers['x-appwrite-key'];
//get required values from environment variables
const endpoint = process.env.APPWRITE_FUNCTION_API_ENDPOINT ?? '';
const projectId = process.env.APPWRITE_FUNCTION_PROJECT_ID ?? '';
const dbId = process.env.DB_ID ?? '';
const userCollectionId = process.env.USER_COLLECTION_ID ?? '';
const client = new Client()
.setEndpoint(endpoint)
.setProject(projectId)
.setKey(apiKey);
const db = new Databases(client);
var user = await db.getDocument(
dbId,
userCollectionId,
userId
);
var credits = user['credits'];
var updatedCredits = credits - 1;
log('credits: ' + credits + 'updatedCredits: ' + updatedCredits);
log(user);
var update = await db.updateDocument(
dbId,
userCollectionId,
userId,
{
'credits': updatedCredits
}
);
log(update);
return res.json({"data":user});
};```
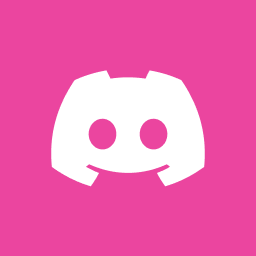
@D5 can you confirm the issue on your side as well?
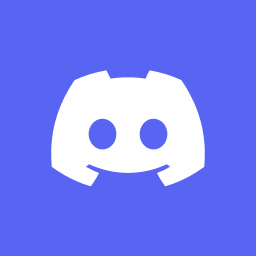
I can't confirm unfortunately, but you can create a bug report on github with the reprodcution steps
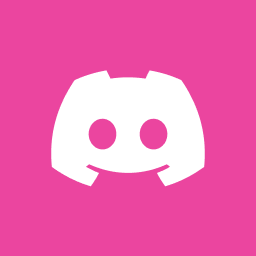
Yes i did it. Btw can't you refer any of the core Appwrite team members to look into this thread.
It would be highly appreciatable.
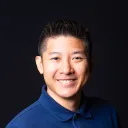
would you please clarify exactly what version is listed in your requirements file?
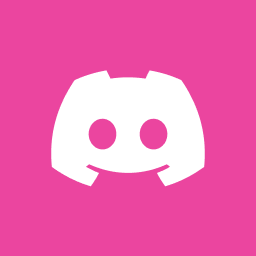
I am using appwrite-7.0.0
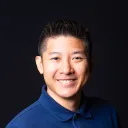
can you try with 6.1.0?
Recommended threads
- my database attribute stuck in processin...
when i created attributes in collection 3 of those attributes become "processing", and they are not updating, the worst thing is that i cant even delete them s...
- Error 1.7.4 console team no found
In console when i go to auth, select user, select a membership the url not work. Only work searching the team. It is by the region. project-default- and i get ...
- Is Quick Start for function creation wor...
I am trying to create a Node.js function using the Quick Start feature. It fails and tells me that it could not locate the package.json file. Isn't Quick Start ...
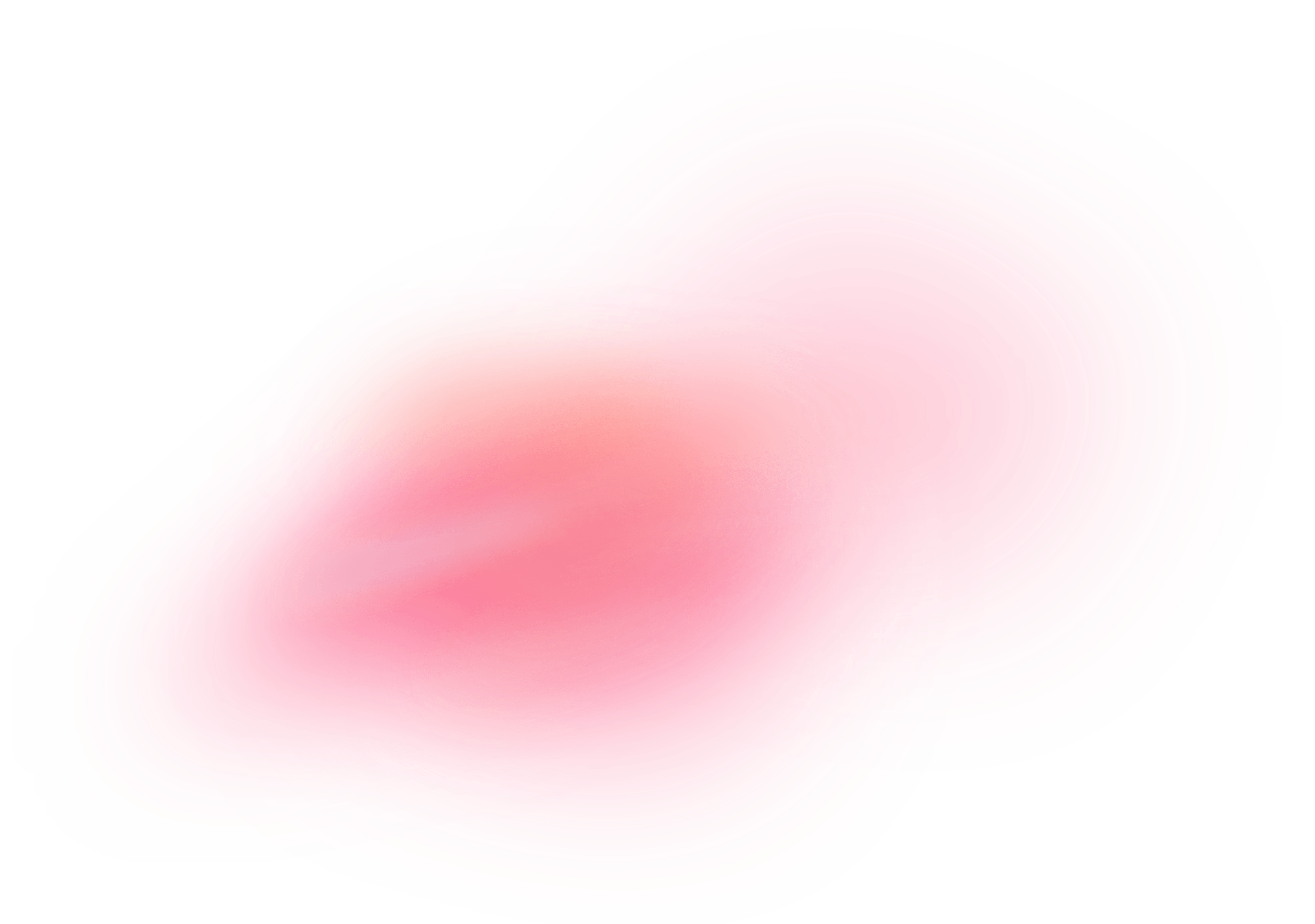