
I have implemented an email verification feature using Appwrite, but the verification process fails when the user clicks the email link. Here's what I'm working with:
Dart Code (Send Verification Email)
Future<void> sendEmailAndPasswordVerificationLink(String userId, String secret) async {
final token = await account.createVerification(url: 'http://67004d383faf2a735120.appwrite.global');
print(token.toMap());
}
Appwrite Cloud Function
import { Client, Users, Account } from 'node-appwrite';
export default async ({ req, res, log, error }) => {
const client = new Client()
.setEndpoint(process.env.APPWRITE_FUNCTION_API_ENDPOINT)
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(req.headers['x-appwrite-key'] ?? '');
const account = new Account(client);
const user = new Users(client);
try {
const { userId, secret } = req.query;
if (!userId || !secret) {
throw new Error('Missing userId or secret in query parameters');
}
const response = await account.updateVerification(userId, secret);
log(req.query);
return res.json({
success: true,
message: 'User verification successful',
data: response,
});
} catch (err) {
log(req.query);
return res.json({
success: false,
message: 'User verification failed',
error: err.message,
});
}
};
Problem:
I am successfully receiving the email, but when I click the verification link, the following error occurs:
{
"success": false,
"message": "User verification failed",
"error": "app.66f7536e00244af68e02@service.cloud.appwrite.io (role: applications) missing scope (public)"
}
It seems like there is a missing scope or permission issue, but I'm unsure how to resolve this. Could someone help me understand what this error means and how I can fix it?
Recommended threads
- Need help with createExecution function
Hi, Need some help understanding createExecution. When requesting function execution via createExecution, the function handler arguments are incorrect and rese...
- Error: User (role: guests) missing scope...
I want to send a verification code to the user and the given phone number and check it and create a session right after the user entered the secret. For me that...
- Apple OAuth Scopes
Hi Hi, I've configured sign in with apple and this is the response i'm getting from apple once i've signed in. I cant find anywhere I set scopes. I remember se...
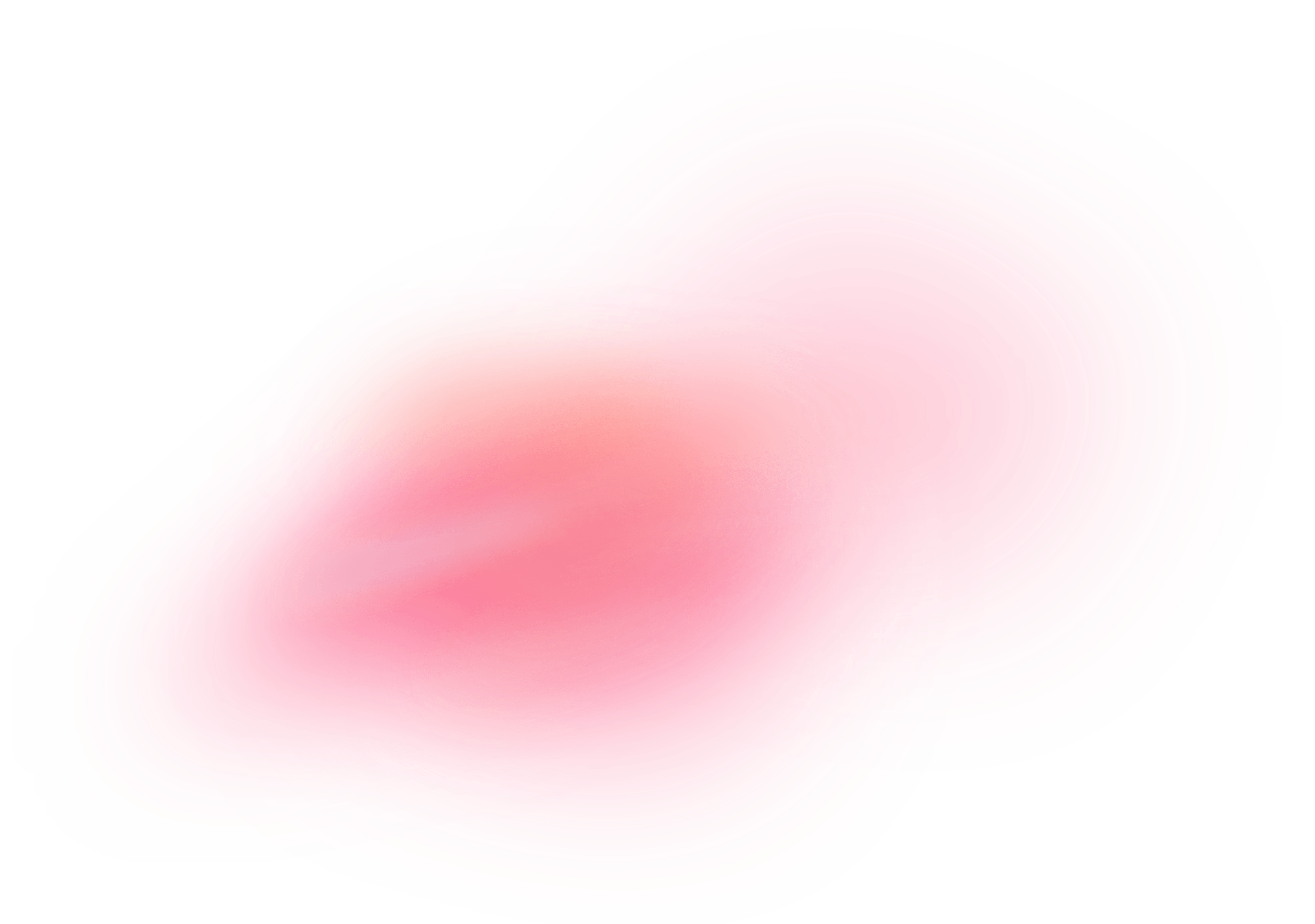