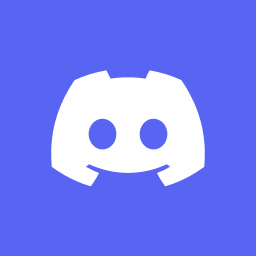
import { default as axios } from 'axios';
import fetch from 'node-fetch';
import { WebhookReceiver } from 'livekit-server-sdk';
// This is your Appwrite function
// It's executed each time we get a request
export default async ({ req, res, log, error }) => {
const client = new Client();
const functions = new Functions(client);
const database = new Databases(client);
client.setEndpoint('https://cloud.appwrite.io/v1')
.setProject(process.env.APPWRITE_FUNCTION_PROJECT_ID)
.setKey(process.env.APPWRITE_API_KEY);
const ENDPOINT = process.env.MY_APPWRITE_ENDPOINT;
const PROJECT_ID = process.env.APPWRITE_PROJECT_ID;
const API_KEY = process.env.APPWRITE_API_KEY;
const DATABASE_ID = process.env.DATABASE_ID;
const LIVEKIT_STREAMING_COLLECTION = process.env.LIVEKIT_STREAMING_COLLECTION;
const LIVE_KIT_API = process.env.LIVE_KIT_API;
const LIVE_KIT_SEC_KEY = process.env.LIVE_KIT_SEC_KEY;
const LIVE_KIT_WEBSOCKET = process.env.LIVE_KIT_WEBSOCKET;
const receiver = new WebhookReceiver(LIVE_KIT_API, LIVE_KIT_SEC_KEY);
if (req.method === 'POST') {
const jsondData = JSON.parse(req.bodyRaw);
const VideoLibraryId = jsondData.VideoLibraryId;
const VideoGuid = jsondData.VideoGuid;
const Status = jsondData.Status;
// Event is a WebhookEvent object
const event = await receiver.receive(req.body, req.get('Authorization'));
log(`Events ${event}`);
}
return res.send({method: 'method is POST'});
};
according to the documentation found on this page https://docs.livekit.io/home/server/webhooks,
i need to access the req.get()
but i'm getting error
please how do i do this on Appwrite Cloud function?

Just retrieve "Authorization" from the request headers

req.get() does not even exists
Recommended threads
- Need help with createExecution function
Hi, Need some help understanding createExecution. When requesting function execution via createExecution, the function handler arguments are incorrect and rese...
- Need Help with Google OAuth2 in Expo usi...
I'm learning React Native with Expo and trying to set up Google OAuth2 with Appwrite. I couldn't find any good docs or tutorials for this and my own attempt did...
- Got message for auto payment of 15usd fo...
how did this happen? 1. i claimed my 50usd credits via jsm hackathon - https://hackathon.jsmastery.pro/ 2. it asked me which org. to apply the credits on, i se...
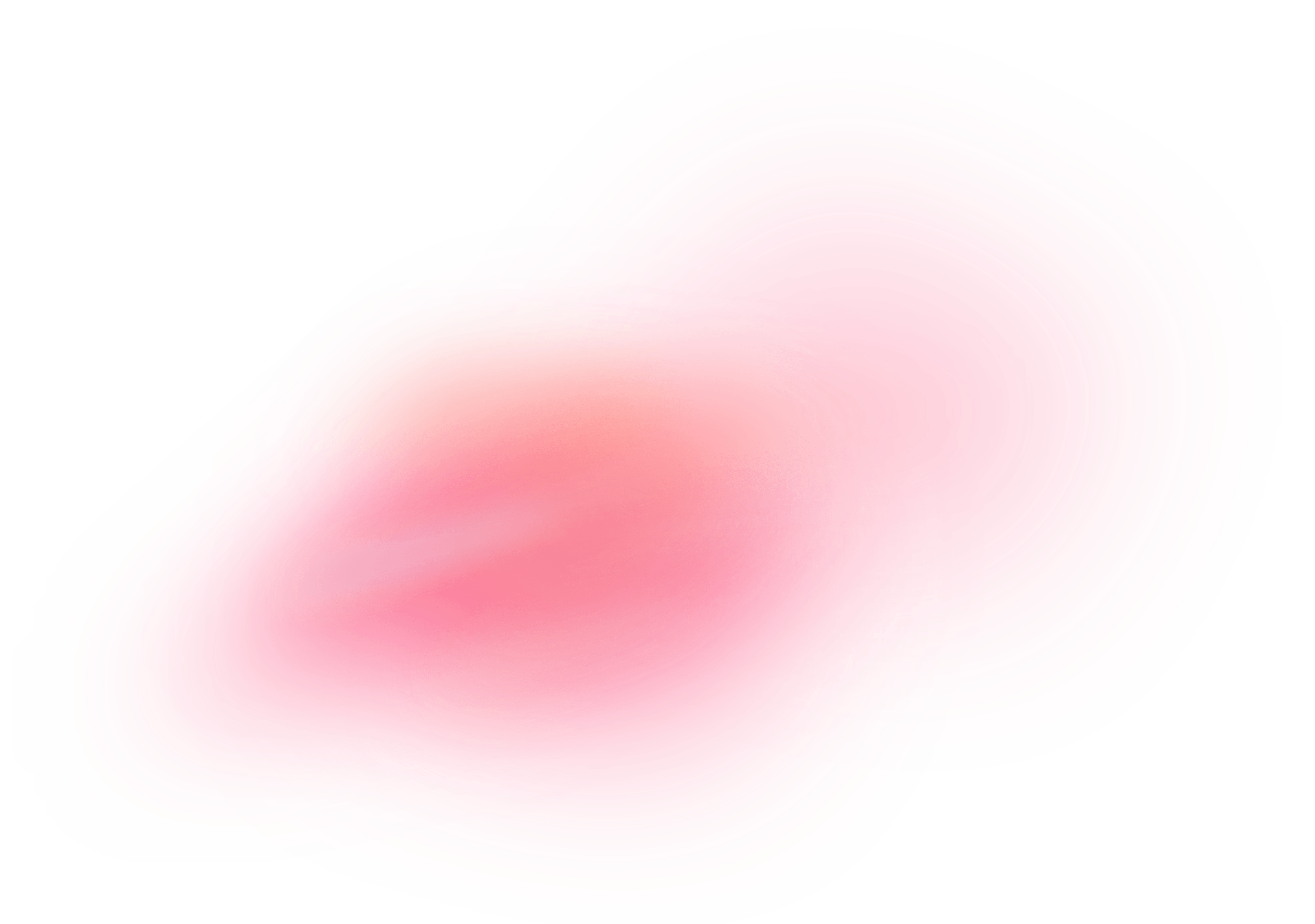