
here's the code for my API:
TypeScript
// /api/getbots.js
const sdk = require('node-appwrite');
async function getBotLinks(JWT) {
console.log(JWT)
console.log('1')
try {
const client = new sdk.Client()
.setEndpoint('https://cloud.appwrite.io/v1') // Your API Endpoint
.setProject('pysec-tools') // project
.setJWT(JWT)
const account = new sdk.Account(client);
const databases = new sdk.Databases(client);
const result = await databases.listDocuments(
'db', // databaseId
'66f3feb30027fc479578', // collectionId
[] // queries (optional)
);
console.log(result)
return [result, null];
} catch (error) {
return [false, error]; // Return false and the error
}
}
export default async function handler(req, res) {
const { JWT } = req.body;
// Initialize variables
let result = null;
let msg = '';
try {
// Correctly destructuring the array returned from checkSession
[result, msg] = await getBotLinks(JWT.jwt);
} catch (err) {
console.log('ERROR!')
console.log(err.stack)
return res.json({ result: null, message: err.stack });
}
console.log(result)
console.log(msg)
// Return the response with logged_in and message
return res.json({ result: result, message: msg });
}
the logs: ill share in next msg
TL;DR
Developers are having JWT permission issues when trying to list documents in an API. The error message states that the user is not authorized. The issue lies in the server-side code, not the client-side. They should ensure that the proper permissions are set up for the user in the Appwrite console. 
TypeScript
(THE JWT, I CENSORED)
1
false
AppwriteException: The current user is not authorized to perform the requested action.
at _Client.call (/home/ryanbaig/Projects/Web/PYSEC-TOOLS/node_modules/node-appwrite/src/client.ts:352:12)
at processTicksAndRejections (node:internal/process/task_queues:95:5)
at async Databases.listDocuments (/home/ryanbaig/Projects/Web/PYSEC-TOOLS/node_modules/node-appwrite/src/services/databases.ts:1697:13)
at async getBotLinks (/home/ryanbaig/Projects/Web/PYSEC-TOOLS/api/getnukebots.js:22:18)
at async handler (/home/ryanbaig/Projects/Web/PYSEC-TOOLS/api/getnukebots.js:44:21) {
code: 401,
type: 'user_unauthorized',
response: {
message: 'The current user is not authorized to perform the requested action.',
code: 401,
type: 'user_unauthorized',
version: '1.6.0'
}
}

this is server-side, the below code is used in client-side (client and account alr defined):
TypeScript
async function getLinks() {
const JWT = await account.createJWT();
// Attempt to get the current session
const response = await fetch('/api/getbots', {
method: 'POST', // Specify the method as POST
headers: {
'Content-Type': 'application/json', // Set the content type to JSON
'Accept': 'application/json' // Specify that you expect a JSON response
},
body: JSON.stringify({ JWT: JWT}) // Include the JWT
})
const result = await response.json(); // Await the JSON parsing
console.log(result)
}
getLinks();
Recommended threads
- The current user is not authorized to pe...
I want to create a document associated with user after log in with OAuth. The user were logged in, but Appwrite said user is unauthorized. User is logged in wi...
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- My account is blocked so please check an...
My account is blocked so please unblock my account because all the apps are closed due to which it is causing a lot of problems
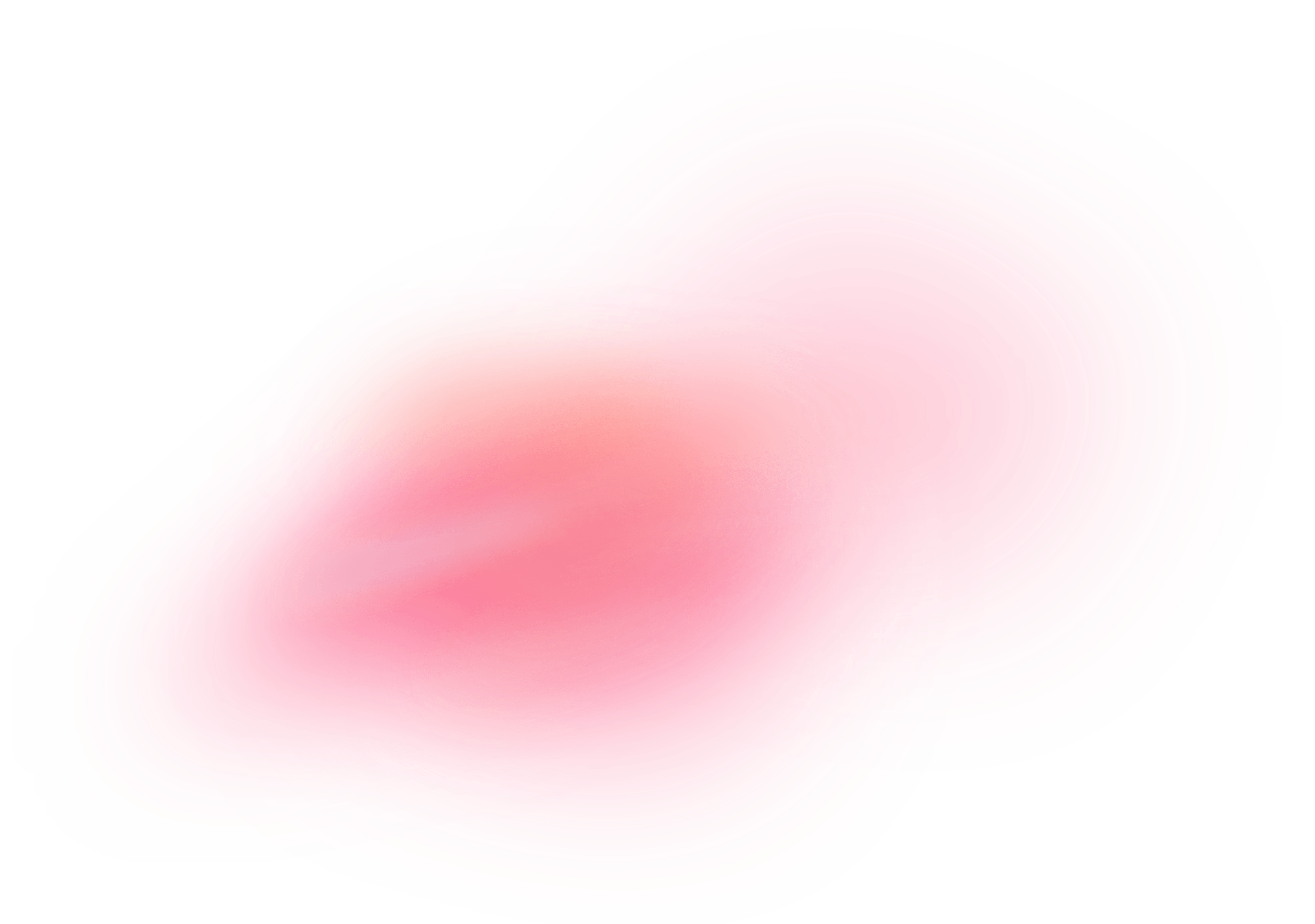