
So I'm attempting to re-do my signup process because I ran into issues adding to the database at the same time.
But im having issues. I read the docs and it absolutely cannot see what im doing wrong. It creates a account just fine on appwrite but wont redirect me to my dashboard.
sign up form code
import {
getLoggedInUser,
createUserClient,
createSessionClient,
} from "@/lib/server/appwrite";
import { redirect } from "next/navigation";
import { ID } from "appwrite";
import { cookies } from "next/headers";
import Link from "next/link";
export default async function RegisterPage() {
const user = await getLoggedInUser();
if (user) redirect("/dashboard");
async function handleRegister(formData) {
"use server";
const organization = formData.get("organization");
const email = formData.get("email");
const password = formData.get("password");
const repeatPassword = formData.get("repeatPassword");
const missionStatement = formData.get("missionStatement");
const organizationWebsite = formData.get("organizationWebsite");
if (password !== repeatPassword) {
// handle this error better
console.log("Passwords do not match");
}
const { account } = await createSessionClient();
try {
await account.create(ID.unique(), email, password, organization);
const session = await account.createEmailPasswordSession(email, password);
cookies().set("my-custom-session", session.secret, {
path: "/",
httpOnly: true,
sameSite: "Strict",
secure: true,
});
redirect("/dashboard");
// const data = { organization, missionStatement, organizationWebsite };
// await saveToDatabase(data);
} catch (error) {
console.log("Registration failed: " + error.message);
}
}
return (
form code stuff
)

my appwrite code
// src/lib/server/appwrite.js
"use server";
import { Client, Account } from "node-appwrite";
import { cookies } from "next/headers";
export async function createSessionClient() {
const client = new Client()
.setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT)
.setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT);
const session = cookies().get("my-custom-session");
if (!session || !session.value) {
console.log("No session");
}
client.setSession(session.value);
return {
get account() {
return new Account(client);
},
};
}
export async function createUserClient() {
const client = new Client()
.setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT)
.setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT);
return {
client,
account: new Account(client),
};
}
export async function getLoggedInUser() {
try {
const { account } = await createSessionClient();
return await account.get();
} catch (error) {
console.log(error);
return null;
}
}
export async function logout() {
try {
const { account } = await createSessionClient();
await account.deleteSession("current");
cookies().delete("my-custom-session");
} catch (error) {
console.error("Error during logout:", error.message);
throw error;
}
}
export async function createAdminClient() {
const client = new Client()
.setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT)
.setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT)
.setKey(process.env.NEXT_APPWRITE_KEY);
return {
get account() {
return new Account(client);
},
};
}
Recommended threads
- Query Appwrite
Hello, I have a question regarding Queries in Appwrite. If I have a string "YYYY-MM", how can I query the $createdAt column to match this filter?
- Different appwrite IDs are getting expos...
File_URL_FORMAT= https://cloud.appwrite.io/v1/storage/buckets/[BUCKET_ID]/files/[FILE_ID]/preview?project=[PROJECT_ID] I'm trying to access files in my web app...
- Invalid document structure: missing requ...
I just pick up my code that's working a week ago, and now I got this error: ``` code: 400, type: 'document_invalid_structure', response: { message: 'Inv...
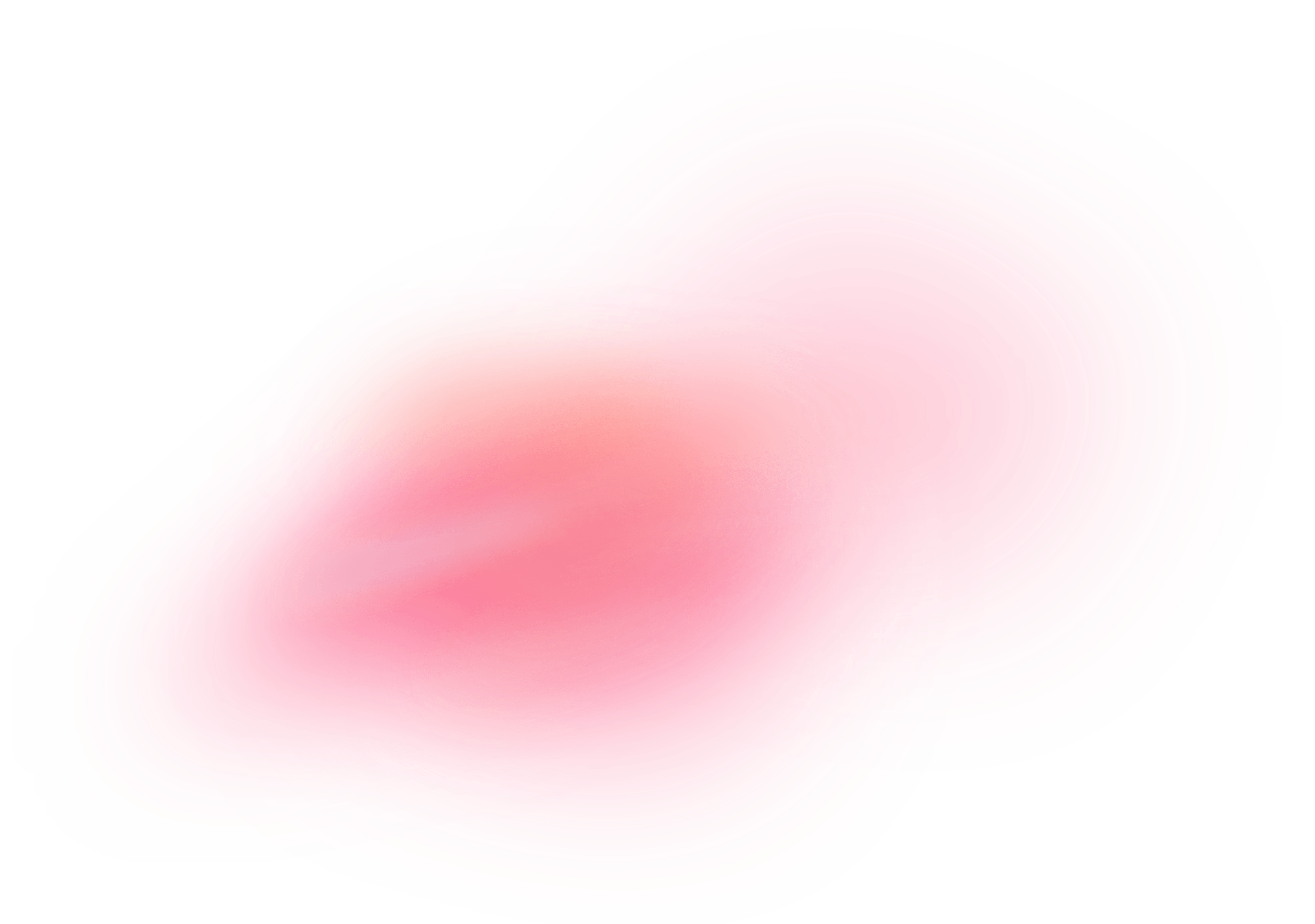