
Registering a user works just fine in my application... Except adding user data to the collection simply does not work. I'm hit with the error :
Registration error: AppwriteException: app....
@service.cloud.appwrite.io (role: applications) missing scope (account)
my code:
import { getLoggedInUser, createUserClient } from "@/lib/server/appwrite";
import { redirect } from "next/navigation";
import { ID } from "appwrite";
import RegisterForm from "@/app/register/registerform";
import { cookies } from "next/headers";
export default async function RegisterPage() {
const user = await getLoggedInUser();
if (user) redirect("/dashboard");
async function handleRegister(formData) {
"use server";
const { account } = await createUserClient();
const {
email,
password,
organization,
missionStatement,
organizationWebsite,
} = formData;
try {
await account.create(ID.unique(), email, password, organization);
const session = await account.createEmailPasswordSession(email, password);
cookies().set("my-custom-session", session.secret, {
path: "/",
httpOnly: true,
sameSite: "Strict",
secure: true,
});
const data = {
organization,
missionStatement,
organizationWebsite,
};
try {
const request = await fetch("/app/api/register", {
method: "POST",
body: data,
});
} catch (error) {
console.error(error);
}
// redirect("/dashboard");
} catch (error) {
console.error("Registration error:", error);
}
}
return <RegisterForm onRegister={handleRegister} />;
}

api route
import {
Client,
Databases,
Account,
ID,
Permission,
Role,
} from "node-appwrite";
import { cookies } from "next/headers";
export async function POST(request) {
try {
const cookie = cookies().get("my-custom-session");
const client = new Client()
.setEndpoint("https://cloud.appwrite.io/v1")
.setProject("...")
.setSession(cookie.value);
const account = new Account(client);
const databases = new Databases(client);
try {
const user = await account.get();
const userID = user.$id;
const data = await request.json();
const result = await databases.createDocument(
"...", // databaseId
"...", // collectionId
ID.unique(), // documentId
data,
[
Permission.read(Role.user(userID)),
Permission.write(Role.user(userID)),
],
);
console.log("Document created:", result);
return result;
} catch (err) {
console.log("Error in document creation:", err);
}
} catch (error) {
console.error("Error creating document:", error);
throw error;
}
}

missing scope (account)
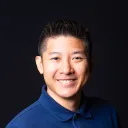
you're using an API key somehwere where you shouldn't

i'm using an api key here on the server sdk. What should i use instead?
export async function createUserClient() {
const client = new Client()
.setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT)
.setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT)
.setKey(process.env.NEXT_APPWRITE_KEY);
return {
client,
account: new Account(client),
};
}
Recommended threads
- Stuck in "deleting"
my parent element have relationship that doesnt exist and its stuck in "deleting", i cant delete it gives me error: Collection with the requested ID could not b...
- Help with 409 Error on Relationship Setu...
I ran into a 409 document_already_exists issue. with AppWrite so I tried to debug. Here's what I've set up: Collection A has 3 attributes and a two-way 1-to-m...
- Database Double Requesting Error.
I am getting error for creating new document in an collection with new ID.unique() then too getting error of existing document. When button is pressed one docum...
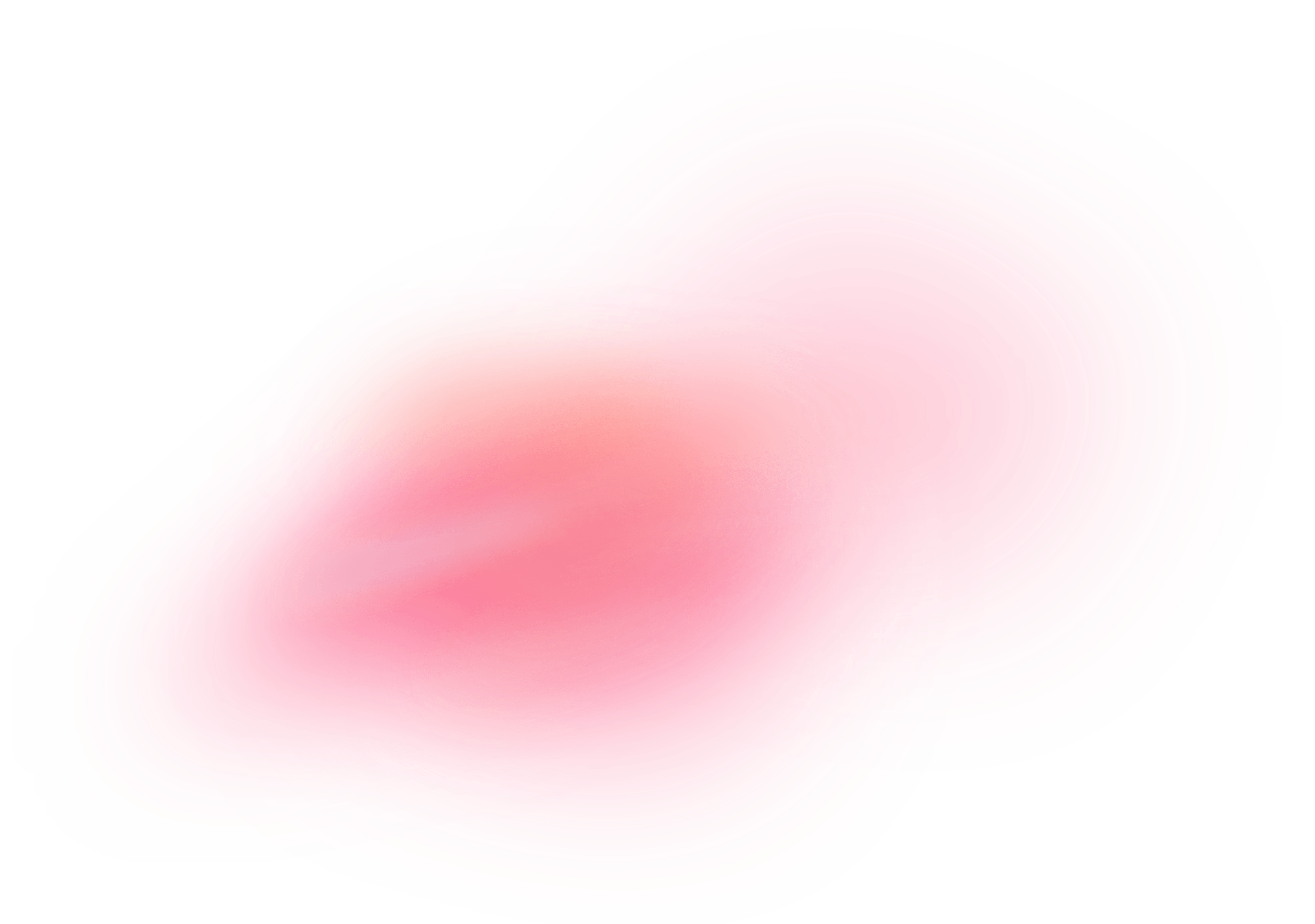