
I have created an account with appwrite then logged it. both of them are working fine. but when i'm trying to get the user by using this method:
export const getAccount = async () => {
try {
const user = await account.get();
return user;
} catch (error: any) {
console.error("An error occurred while fetching user:", error);
}
};
it's not getting any user info. how can i fix this issue?
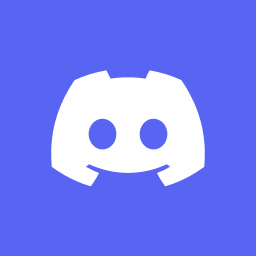
You're getting error?
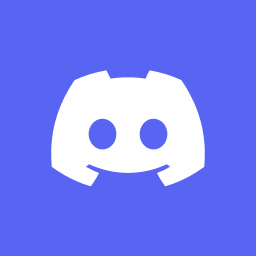
If so, it could be that the user is not logged in
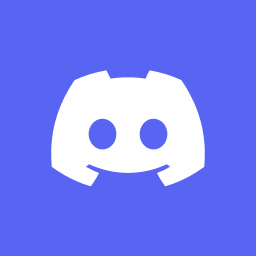
What error are you getting?

I am not getting any error. it's just not giving me the user info. here is my auth related code.
// Create APPWRITE ACCOUNT
export const createAccount = async (user: CreateAccountParams) => {
try {
const newUser = await account.create(
ID.unique(),
user.email,
user.password,
user.name,
);
await loginAccount({
email: user.email,
password: user.password
});
return parseStringify(newUser);
} catch (error: any) {
console.error("An error occurred while creating a new user:", error);
throw error; // Re-throw the error to handle it further up the call stack if needed
}
};
// Login APPWRITE ACCOUNT
export const loginAccount = async (user: LoginAccountParams) => {
try {
const loginUser = await account.createEmailPasswordSession(
user.email,
user.password
);
console.log(loginUser, "loginUser");
return parseStringify(loginUser);
} catch (error: any) {
console.error("An error occurred while fetching user:", error.message);
}
};
// GET APPWRITE Account
export const getAccount = async () => {
try {
const user = await account.get();
return user;
} catch (error: any) {
console.error("An error occurred while fetching user:", error);
}
};

I also check the cookies and localstorage. it's not saving my credential.

like secret key
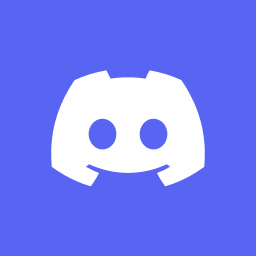
Are you using some web framework like NextJS?

next js. yes
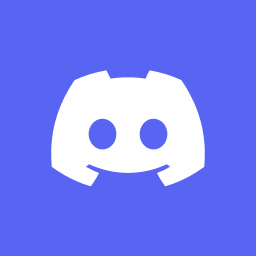
Then probably it's because of SSR. You will probably need to disble SSR or implement SSR login: https://appwrite.io/docs/tutorials/nextjs-ssr-auth/step-1
Recommended threads
- Please Help! Appwrite Sub Issue.
Project ID# 66045eb4ad78658d4b8e Good morning, My billing cycle ends on the 18th of every month and for the last 3 months I was on the Pro plan as an invitati...
- Paid Gig, Need quick help in intergratin...
Paid Gig, Need quick help in intergrating a react native project with expo and appwrite.
- Google Login Not working
when i use google login im not getting any secret/session anything to my success url that is http://localhost:3000/home The session is getting created i can see...
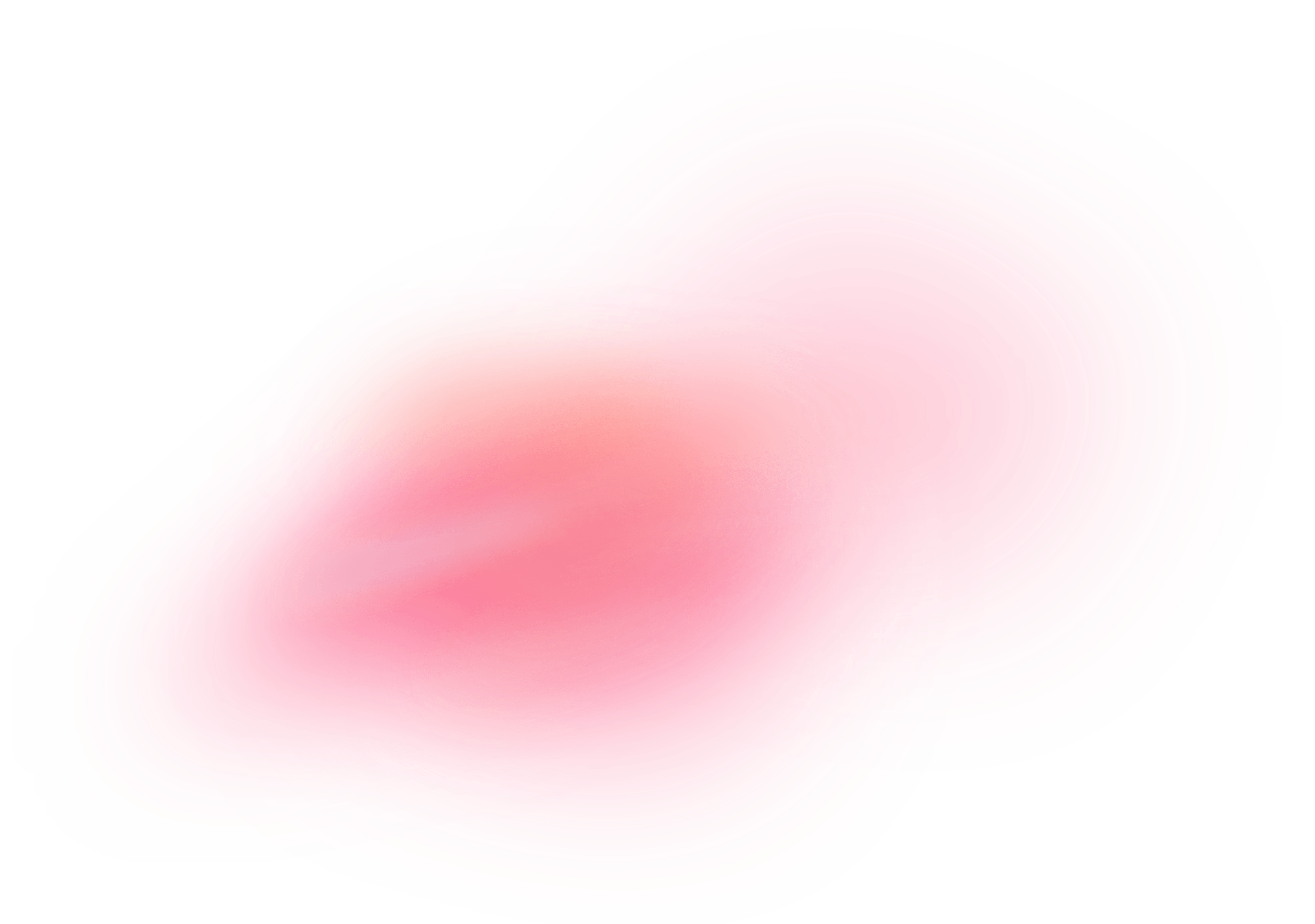