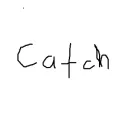
Hey there, I am trying to add an image upload feature to my ReactNative app which stores it within an Appwrite storage bucket.
Here is my current code for the image picker:
TypeScript
const pickImage = async () => {
let result = await ImagePicker.launchImageLibraryAsync({
mediaTypes: ImagePicker.MediaTypeOptions.All,
allowsEditing: true,
aspect: [4, 3],
quality: 1,
});
if (!result.canceled) {
console.log(await uploadImage(result.assets[0]));
}
};```
However when I try to console.log the result it is undefined and nothing gets created.
```js
export const uploadImage = async (file) => {
try {
const response = await storage.createFile(
appwriteConfig.storageId,
ID.unique(),
file
);
return response;
} catch (error) {
throw new Error(error);
}
}```
TL;DR
Developers are trying to upload images with React Native using fetch and blob but facing issues with null response. The solution provided is to change it to use `ImagePicker.launchImageLibraryAsync` and then pass the image to the `uploadImage` function. This should successfully upload the image to Appwrite storage bucket.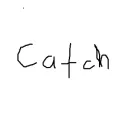
If I change it into uploading as a blob like:
TypeScript
export const uploadImage = async (image) => {
console.log("===========================")
const response = await fetch(image);
console.log(response);
const blob = await response.blob();
console.log(blob);
try {
const response = await storage.createFile(
appwriteConfig.storageId,
ID.unique(),
file
);
return response;
} catch (error) {
throw new Error(error);
}
}```
It prints the blob, however nothing happens with response (No error, no nothing, but the file isnt uploaded and response is null)
Recommended threads
- Get all Documents and Expanded Relations...
I have multiple (quite small) collections that have many-to-many relationships. How can we return all documents and expanded relationship for a specific collec...
- Functions Failing Due to Installed Depen...
Hi team! Before anything, I am on the so far while we're in the planning phase of the app, and will be upgrading to pro soon. If there's a possibility that this...
- The new Bulk API and Realtime updates
It seems that the new Bulk API doesn't actually send realtime updates which is making a huge problem for me. I also wanted to know different is it deleting mul...
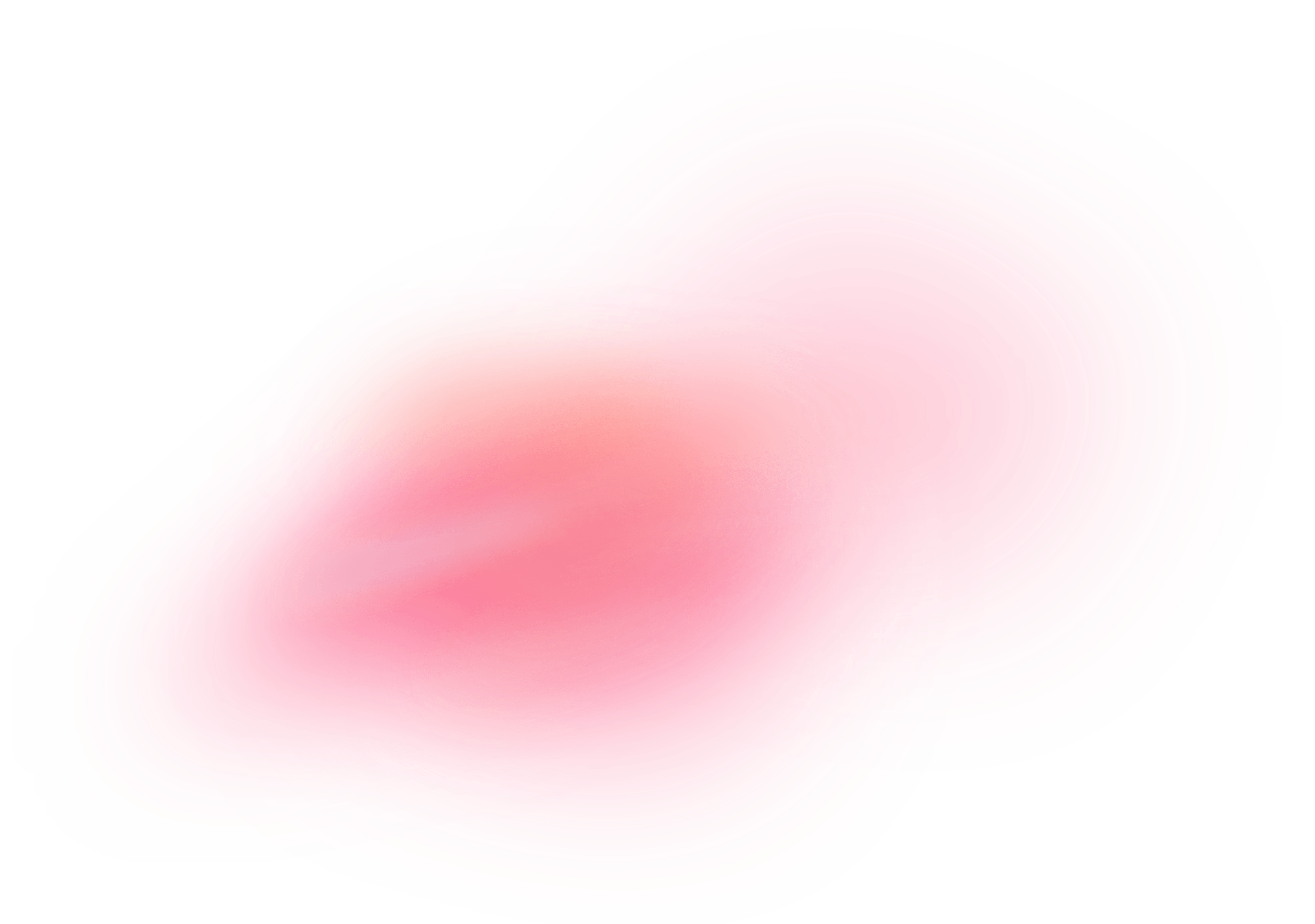