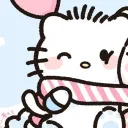
Hello everyone, I really hope someone can help me out here. I have been stuck on this issue since yesterday. So for my current project I am setting up a simple Login/ Registration workflow. For my app I'm using PyQt/PySide6 in combination with the Appwrite Python Server SDK to achieve this. I can without any issues create new users in the registration and in the login creating new sessions works without any issues at all using the create email password session function. My problems come now when I try to do any sort of session handling/management like getting a sessions list or updating a session as any other session operations other than creating one print this error. Has anyone experienced something similar and has maybe a solution? Also note that I'm specifically not using and dont want to use an API key on user authentication stuff for security reasons. But according to the docs an API key shouldn't be necessary anyways. I'll post the code down below as I'm running out of characters.
in raise_for_status raise HTTPError(http_error_msg, response=self) requests.exceptions.HTTPError: 401 Client Error: Unauthorized for url: https://cloud.appwrite.io/v1/account/sessions
During handling of the above exception, another exception occurred: raise AppwriteException(response.json()['message'], response.status_code, response.json().get('type'), response.json()) appwrite.exception.AppwriteException: User (role: guests) missing scope (account)
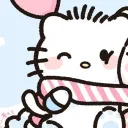
from PySide6.QtWidgets import QWidget, QPushButton,QVBoxLayout,QFormLayout,QLineEdit,QLabel, QMessageBox
from PySide6.QtGui import Qt
from Application.AccountManagement.accountManager import AccountSession
from appwrite.exception import AppwriteException
import json
class Login(QWidget):
def __init__(self):
super().__init__()
layout = QVBoxLayout()
self.session = AccountSession()
title = QLabel("Login")
title.setAlignment(Qt.AlignCenter)
layout.addWidget(title)
form_layout = QFormLayout()
self.username_input = QLineEdit()
self.password_input = QLineEdit()
self.password_input.setEchoMode(QLineEdit.Password)
form_layout.addRow("Username:", self.username_input)
form_layout.addRow("Password:", self.password_input)
layout.addLayout(form_layout)
login_button = QPushButton("Login")
login_button.clicked.connect(self.login)
layout.addWidget(login_button)
register_button = QPushButton("Register")
register_button.clicked.connect(self.show_register_page)
layout.addWidget(register_button)
self.setLayout(layout)
def login(self):
username = self.username_input.text()
password = self.password_input.text()
try:
active_session = self.session.account.create_email_password_session(email=username, password=password)
active_session_id = active_session["$id"]
sessions = self.session.account.list_sessions()
print(sessions)
with open('session.json', 'w') as f:
json.dump(active_session,f,indent=4)
QMessageBox.information(self, 'Success', 'Logged in successfully!')
self.parent().setCurrentIndex(2)
except AppwriteException as e:
QMessageBox.warning(self,'Error', f'Login failed: {e.message}')

Maybe I'm wrong but I think the issue is the sdk itself. The python sdk is supposed to handle server tasks
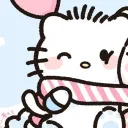
from appwrite.client import Client
from appwrite.services.account import Account
class AccountSession:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(AccountSession, cls).__new__(cls)
return cls._instance
def __init__(self):
if not hasattr(self,"_initialized"):
self._initialized = True
self._client = Client()
(self._client
.set_endpoint('endpoint')
.set_project('project id')
)
self.account = Account(self._client)
self._active_session = None
@property
def active_session(self):
return self._active_session
@active_session.setter
def active_session(self, value):
self._active_session = value

I don't think it is optimized for frontend stuff
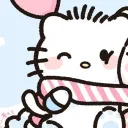
thats what I thought hmm its a big bummer
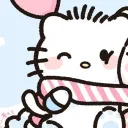
looks like I'll have to use the REST API and build the workflow myself

You could take the python server sdk as baseline and improve upon that
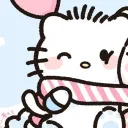
its such a missed opportunity on their side, not supporting it alongside Java and C++ for Qt. I have been trying everything haha
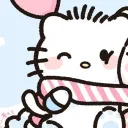
yeaah. I'll wait though maybe someone has a miracle solution😅

I think you have to fork the sdk there is no way around that. Since the sdk is supposed to handle server tasks it probably did not implement any session management

So if you login it does not persist the session

No session => User (role: guests) missing scope (account)
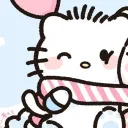
okok got it thanks

Yeah the demand wasn't that high for that I guess 😅
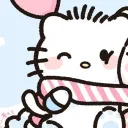
sorry if it's a dumb question but I'm still quite a noob xD how would I go about it if you dont mind explaining it?
Recommended threads
- SSO google apple not working anymore
We use Apple and Google sso in our react native app. Everything worked fine until we noticed today that we see general argument error. We did not change anythi...
- Is my approach for deleting registered u...
A few weeks ago, I was advised not to use the registered users' id in my web app. Instead, I store the publicly viewable information such as username and email ...
- Flutter OAuth2 Google does not return to...
When the flow starts, the browser opens, I select an account, and it keeps showing: """ Page not found The page you're looking for doesn't exist. `general_rout...
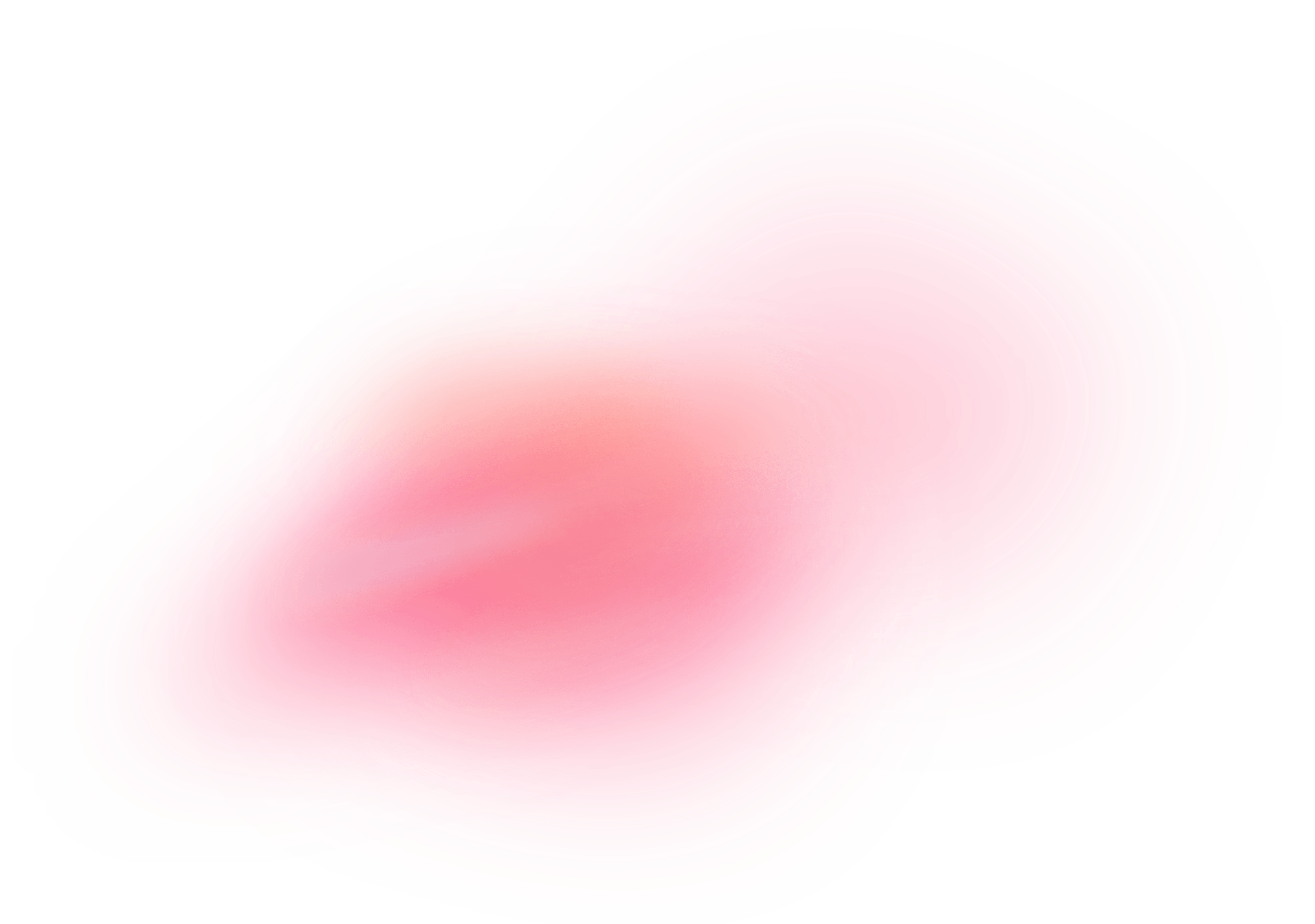