
I using Remix and Appwrite. I currently have a working Login Page that creates the SSR session, but when I try to forward the user agent to get more specific information on the Appwrite console (currently displays Windows 95), it fails. Since the problem is most likely due to my stupidity, I wonder how to forward the agent correctly? Any help is appreciated :)
My Code:
//signin.tsx
export async function action({ request }: ActionFunctionArgs) {
let formData = await request.formData();
let email = formData.get("email")?.toString();
let password = formData.get("password")?.toString();
if (!email || !password) {
return new Response("Bad Request", { status: 400 });
}
let userAgent = request.headers.get("User-Agent")?.toString() ||"";
let user = await createSession(email, password, userAgent);
if (user) {
return redirect("/", {
headers: {
"Set-Cookie": await authCookie.serialize(user?.secret),
},
});
}
}
//createSession.ts
export async function createSession(
email: string,
password: string,
userAgent: string
) {
const account = new Account(AdminClient);
try {
console.log("Creating Session");
const session = await account.createEmailPasswordSession(email, password);
console.log(userAgent);
AdminClient.setForwardedUserAgent(userAgent);
console.log(AdminClient.config);
return session;
} catch (error) {
console.error(error);
throw new Error("SignIn failed");
}
}
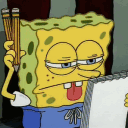
Do you have a specific error message? How are you initializing your AdminClient?

No, I don't get any error message. It just doesn't get sent. This my My AdminClient:
const AdminClient = new Client()
.setEndpoint("https://cloud.appwrite.io/v1") // Your API Endpoint
.setProject("My Project ID") // Your project ID
.setKey("My Key"); // Your secret API key

When logging the User Agent on my server, I receive the following string: "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/127.0.0.0 Safari/537.36"
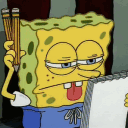
Try setting it prior to making your create session call. It looks like to me all this method does it add a new header (X-Forwarded-User-Agent) to the client.
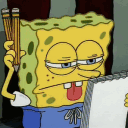

I will try that. Thank you for your help!

I am afraid that it doesn't seem to work. I tried to set it prior to the session call, but the result in the appwrite console is the same: Windows 95.
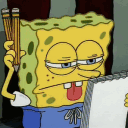
Hmm, I'm not quite sure then, sorry :(

All good. Thank you for your help. I will keep trying to find a solution.

I actually found the solution/ issue. @Kenny To my understanding "setForwardUserAgent()" is pretty useless, unless I am using it very wrong. As far as I understand the logic behind all this, the function adds the "X-Forwarded-User-Agent" Header but that has no effect, because it seems to me that the appwrite console uses the "user-agent" header, which depends on the server, not the actual user. However overwriting this very specific header seems to do the trick.
The workaround:
// ...
const account = new Account(AdminClient);
account.client.addHeader("user-agent", userAgent);
// create Session after this
account: {
headers: {
'x-sdk-name': 'Node.js',
...
// This needs to be overwritten
'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64)' AppleWebKit/537.36 (KHTML, like Gecko) Chrome/128.0.0.0 Safari/537.36',
'X-Appwrite-Response-Format': '1.5.0',
'X-Appwrite-Project': 'Id',
'X-Appwrite-Key': 'Key',
// Seems to have no effect
'X-Forwarded-User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/128.0.0.0 Safari/537.36'
}
} ```
Not sure if this is an actual issue or intended behavior though :/
Recommended threads
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- My account is blocked so please check an...
My account is blocked so please unblock my account because all the apps are closed due to which it is causing a lot of problems
- Applying free credits on Github Student ...
So this post is kind of related to my old post where i was charged 15usd by mistake. This happens when you are trying to apply free credits you got from somewh...
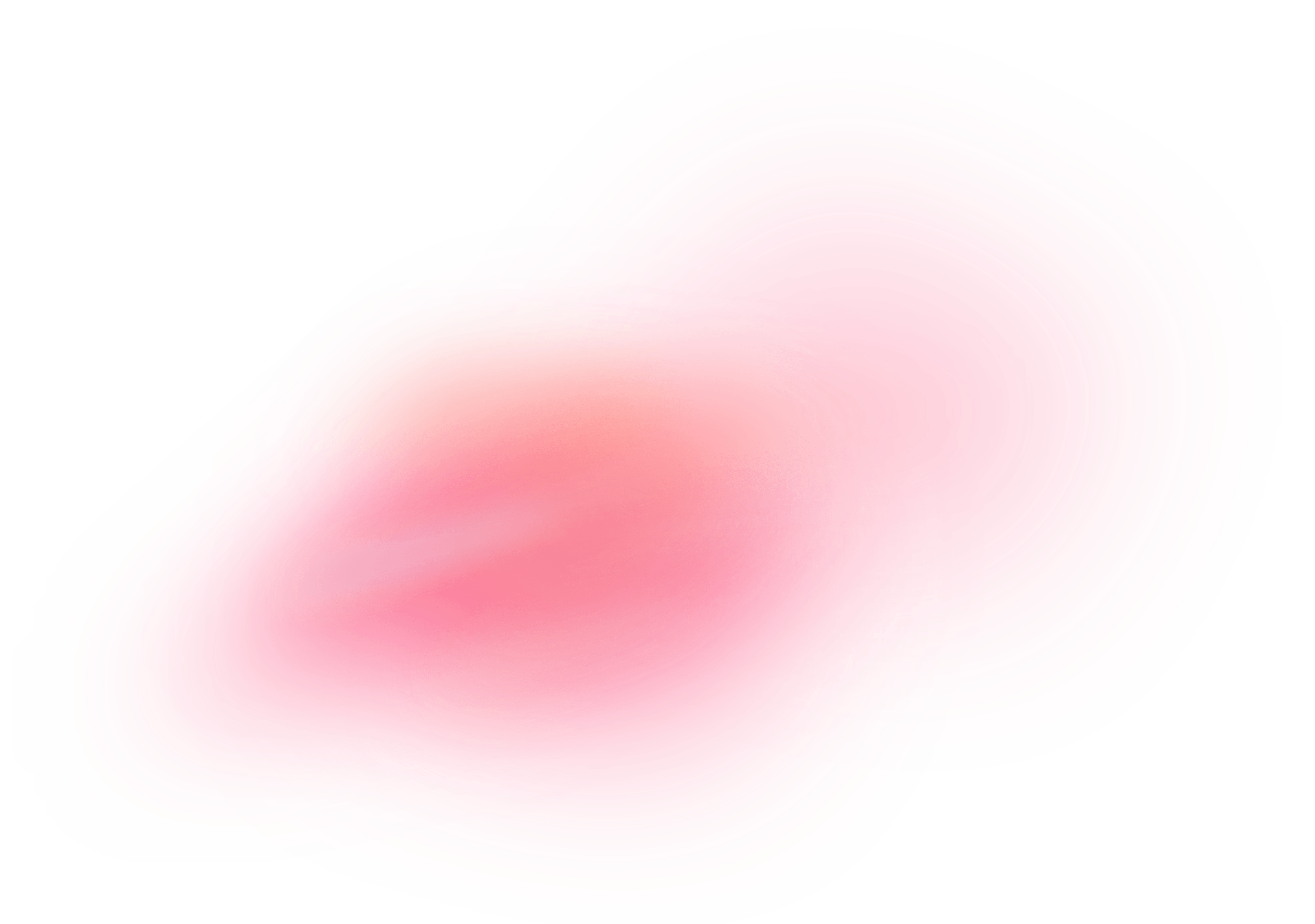