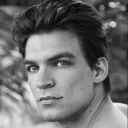
Using 1.5.X (latest, I think current is .7) and my self-hosted server has a lot of "fetch failed"'s happening. I can't tell why, or what's going on, but it's happening sometimes 3-4 times per instance e.g. I am fetching a list of items from the database. Each item pulls a "favorited" status from a favorites collection, but for whatever reason a lot of them are "fetch failed" and granted, it makes a good amount of requests to load each item, it needs the owner, the category, and other things, but. It still (IMO) shouldn't be taking this long with this many "fetch failed"'s
It's also causing my getDocument
to fail too
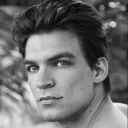
for example, this code keeps repeatedly failing to get the user's $id from it
async getOrCreateFavoriteForCurrentUser(): Promise<APIResponse<Favorites>> {
const user = await tryAwaitWithRetry(async () => await this.account.get());
return this.getOrCreateFavorite(user.$id);
}
/**
* Retrieves the current favorite of the user or creates a new one if it doesn't exist.
* @returns The current favorite document.
*/
async getOrCreateFavorite(userId: string): Promise<APIResponse<Favorites>> {
try {
const favorite = await tryAwaitWithRetry(
async () => await this.getFavorite(userId)
);
if (favorite.data) {
return {
status: 200,
data: favorite.data,
message: "Favorite retrieved successfully",
};
} else {
const createdFavorite = await this.databases.createDocument(
databaseId,
APPWRITE_COLL_FAVORITES,
userId,
{
nftIds: [],
userId: userId,
}
);
return {
status: 201,
data: FavoritesSchema.parse(createdFavorite),
message: "Favorite created successfully",
};
}
} catch (error: any) {
try {
const newFavorite = {
nftIds: [], // Initialize with an empty array or any default values
userId: userId,
};
const createdFavorite = await tryAwaitWithRetry(
async () =>
await this.databases.createDocument(
databaseId,
APPWRITE_COLL_FAVORITES,
userId,
newFavorite,
getDefaultPermissions(userId)
)
);
return {
status: 201,
data: FavoritesSchema.parse(createdFavorite),
message: "Favorite created successfully",
};
} catch (createError) {
if (import.meta.env.DEV) {
console.error(createError);
}
return {
status: 500,
message: "Error creating favorite",
};
}
}
}
Recommended threads
- Type Mismatch in AppwriteException
There is a discrepancy in the TypeScript type definitions for AppwriteException. The response property is defined as a string in the type definitions, but in pr...
- What Query's are valid for GetDocument?
Documentation shows that Queries are valid here, but doesn't explain which queries are valid. At first I presumed this to be a bug, but before creating a githu...
- Unauthorized Charge After Appwrite Pro F...
I was using Appwrite Pro credits worth $100, which were valid until November. During this period, I was exploring Appwrite's services. However, I recently notic...
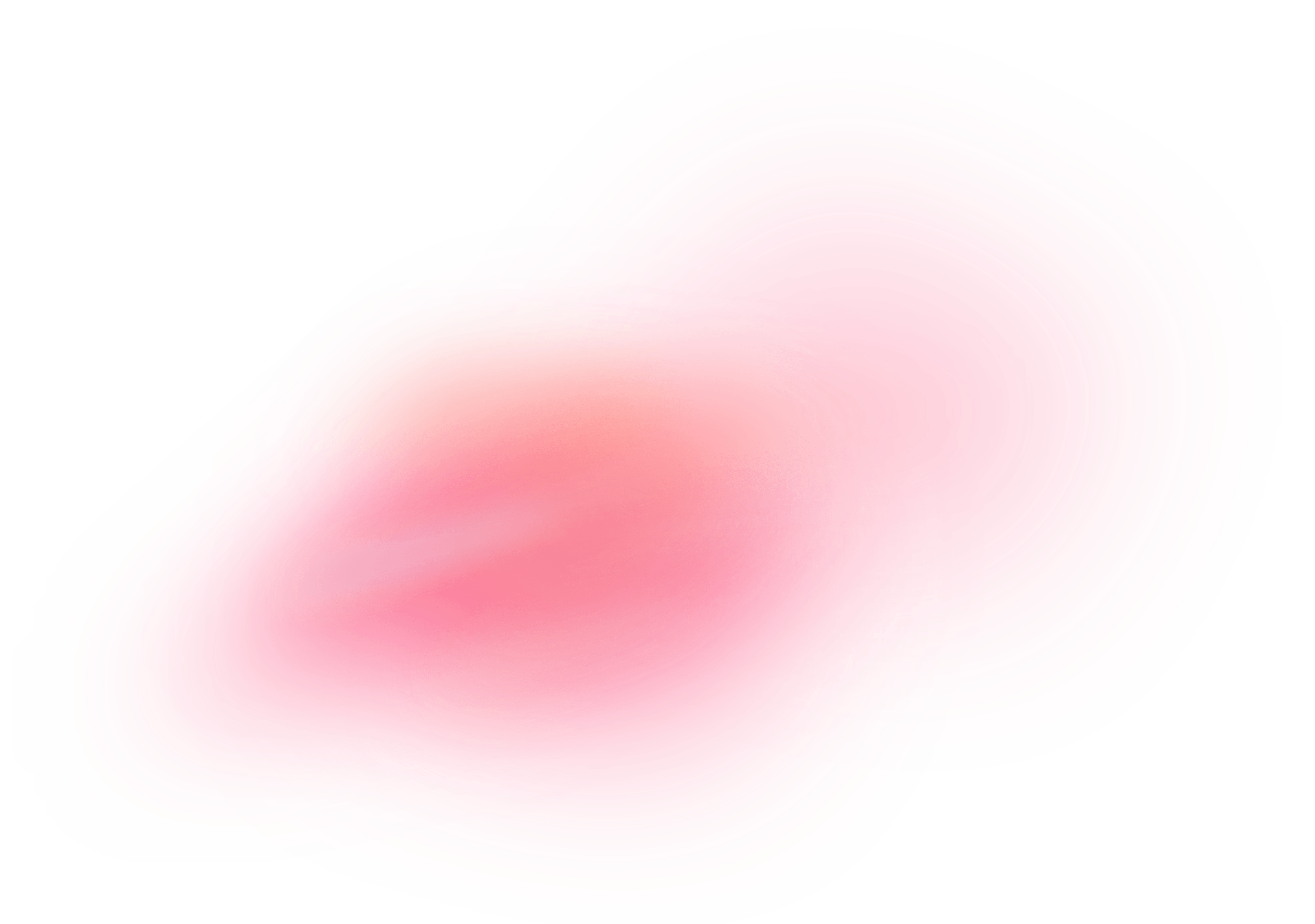