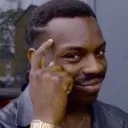
Hello all,
I'm following this documentation https://appwrite.io/docs/quick-starts/apple#step-5
I've created Appwrite.swift file and ContentView.swift fiile with exact same code providing from documentation
ContentView.swift :
import SwiftUI
class ViewModel: ObservableObject {
@Published var email: String = ""
@Published var password: String = ""
}
struct ContentView: View {
@ObservedObject var viewModel = ViewModel()
var body: some View {
VStack {
TextField(
"Email",
text: $viewModel.email
)
SecureField(
"Password",
text: $viewModel.password
)
Button(
action: { Task {
try await Appwrite.onRegister(
viewModel.email,
viewModel.password
)
}},
label: {
Text("Register")
}
)
Button(
action: { Task {
try! await Appwrite.onLogin(
viewModel.email,
viewModel.password
)
}},
label: {
Text("Login")
}
)
}
.padding()
}
}
#Preview {
ContentView()
}
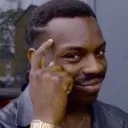
Appwrite.swift
import Foundation
import Appwrite
import JSONCodable
class Appwrite {
var client: Client
var account: Account
public init() {
self.client = Client()
.setEndpoint("https://cloud.appwrite.io/v1")
.setProject("6672009b002541899792")
.setSelfSigned(true)
self.account = Account(client)
}
public func onRegister(
_ email: String,
_ password: String
) async throws -> User<[String: AnyCodable]> {
try await account.create(
userId: ID.unique(),
email: email,
password: password
)
}
public func onLogin(
_ email: String,
_ password: String
) async throws -> Session {
try await account.createEmailPasswordSession(
email: email,
password: password
)
}
public func onLogout() async throws {
_ = try await account.deleteSession(
sessionId: "current"
)
}
}
Got the following error inside the ContentView.swift file :
Instance member 'onRegister' cannot be used on type 'Appwrite'; did you mean to use a value of this type instead?
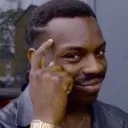
And this from the preview :
== DATE:
Wednesday 17 July 2024 at 16:32:06 Central European Summer Time
2024-07-17T14:32:06Z
== PREVIEW UPDATE ERROR:
SchemeBuildError: Failed to build the scheme “App”
instance member 'onRegister' cannot be used on type 'Appwrite'; did you mean to use a value of this type instead?
Compile ContentView.swift (x86_64):
/Users/stouf/Workspace/FamilyLove/FamilyLove/FamilyLove/ContentView.swift:30:31: error: instance member 'onRegister' cannot be used on type 'Appwrite'; did you mean to use a value of this type instead?
try await Appwrite.onRegister(
^~~~~~~~
/Users/stouf/Workspace/FamilyLove/FamilyLove/FamilyLove/ContentView.swift:41:32: error: instance member 'onLogin' cannot be used on type 'Appwrite'; did you mean to use a value of this type instead?
try! await Appwrite.onLogin(
^~~~~~~~
```

Appwrite
is a class so maybe try creating an instance of it like -
let appwrite = Appwrite()
appwrite.onLogin(...)
seems like issue with docs, @choir24 (Richard)
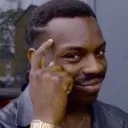
It is not working as well

whats the issue?
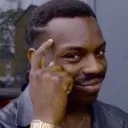
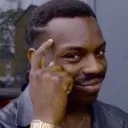
Type 'User<[String : AnyCodable]>' does not conform to the 'Sendable' protocol
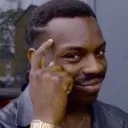
Non-sendable type 'User<[String : AnyCodable]>' returned by implicitly asynchronous call to nonisolated function cannot cross actor boundary
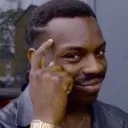
Same for onLogin

I see. atleast the error changed. I am away right now, gonna check this after I come back.
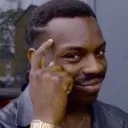
thanks a lot !!
Recommended threads
- Trouble with Anonymous Login on iOS
Ideally I would like to be able to sign in anonymously and persist this user even if they close and reopen the app. Is this possible? Here is what I currently h...
- Flutter OAuth2 Google does not return to...
When the flow starts, the browser opens, I select an account, and it keeps showing: """ Page not found The page you're looking for doesn't exist. `general_rout...
- Email Verification Email
Hi everyone, I’m currently experiencing an issue with the email verification functionality. When I trigger the verification, the request returns a valid respon...
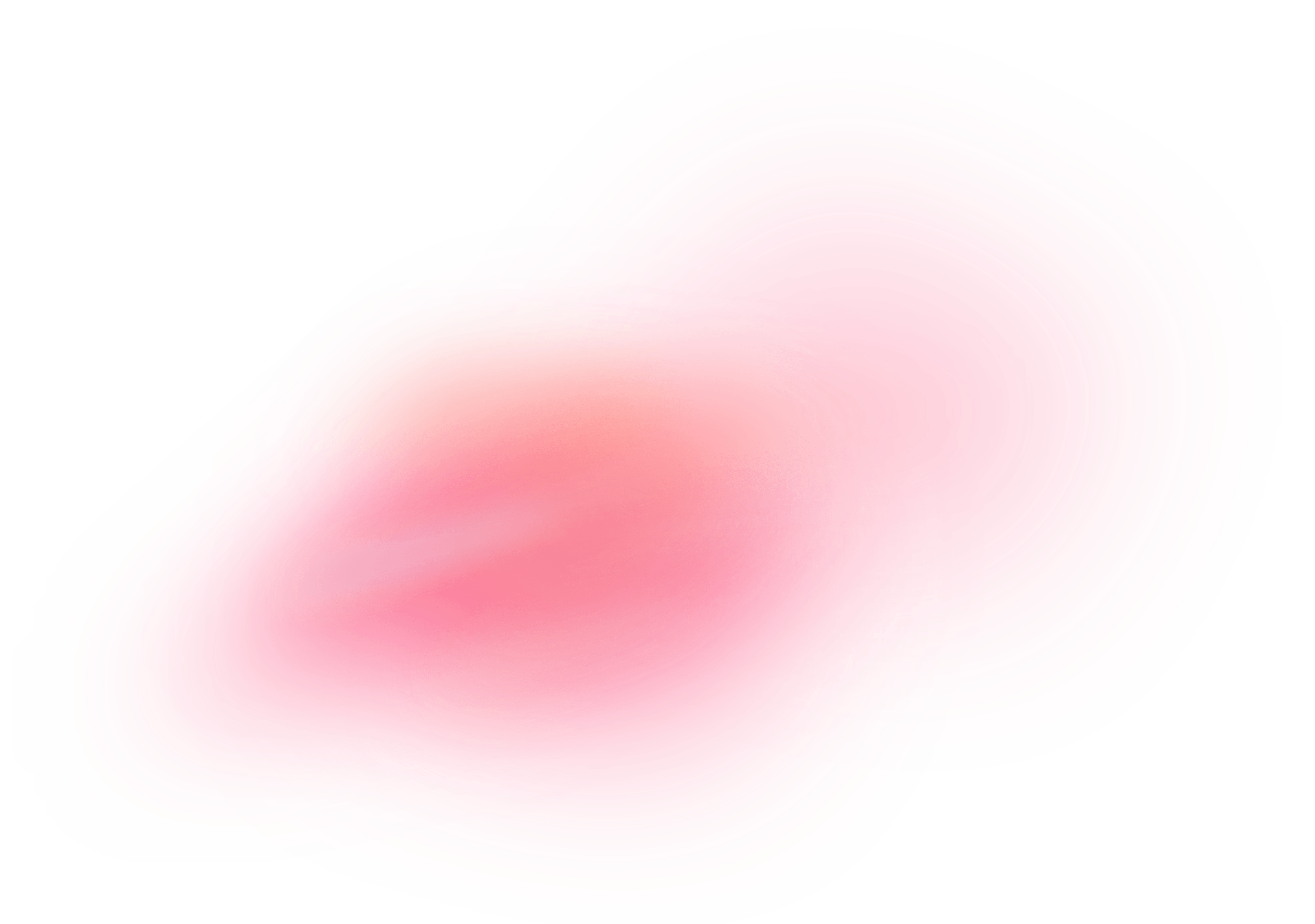