
Hey,
I'm trying to verify the user once they create their account. I'm running an expo project.
I thought that user authentication would be easy but it's turning out to be a headache and I'd really appreciate some guidance.
My question is why, based on the code below, do I get the error
LOG [AppwriteException: User (role: guests) missing scope (account)]
code:
const client = new Client();
client
.setEndpoint(config.endpoint) // Appwrite Endpoint
.setProject(config.projectId) // project ID
.setPlatform(config.platform) // application ID or bundle ID.
;
const account = new Account(client);
// signup
export const createUser = async (email, password) => {
try {
const newAccount = await account.create(ID.unique(), email, password);
if (!newAccount) throw Error('Error creating user');
// login the user
Login(email, password);
// Send verification email
const verify = await account.createVerification('BookQuest://(auth)/verify');
console.log(verify)
return newAccount;
} catch (error) {
console.log(error);
throw new Error(error);
}
}
// signin
export const Login = async (email, password) => {
try {
const session = await account.createEmailPasswordSession(email, password);
return session;
} catch (error) {
throw new Error(error)
}
}
I also need help understanding how the whole verification process should work with an expo app.
Thanks in advance
Recommended threads
- login backend and frontend
I'm using Remix, as my Backend (node). and react on my frontend. Until now, i did SSR login. saved the secret.. and it's fine. For many simpler requests i wish...
- Custom SMS providers for OTP
Is there is any way that we can add custom SMTP servers for sending OTPs. We are seeing issues with appwrite default provider, it doesn't send otps for some num...
- Custom Auth flows
Is there a way to create a user session with just the username from admin client ? We want to implement custom auth flow , once we verify every thing, we want ...
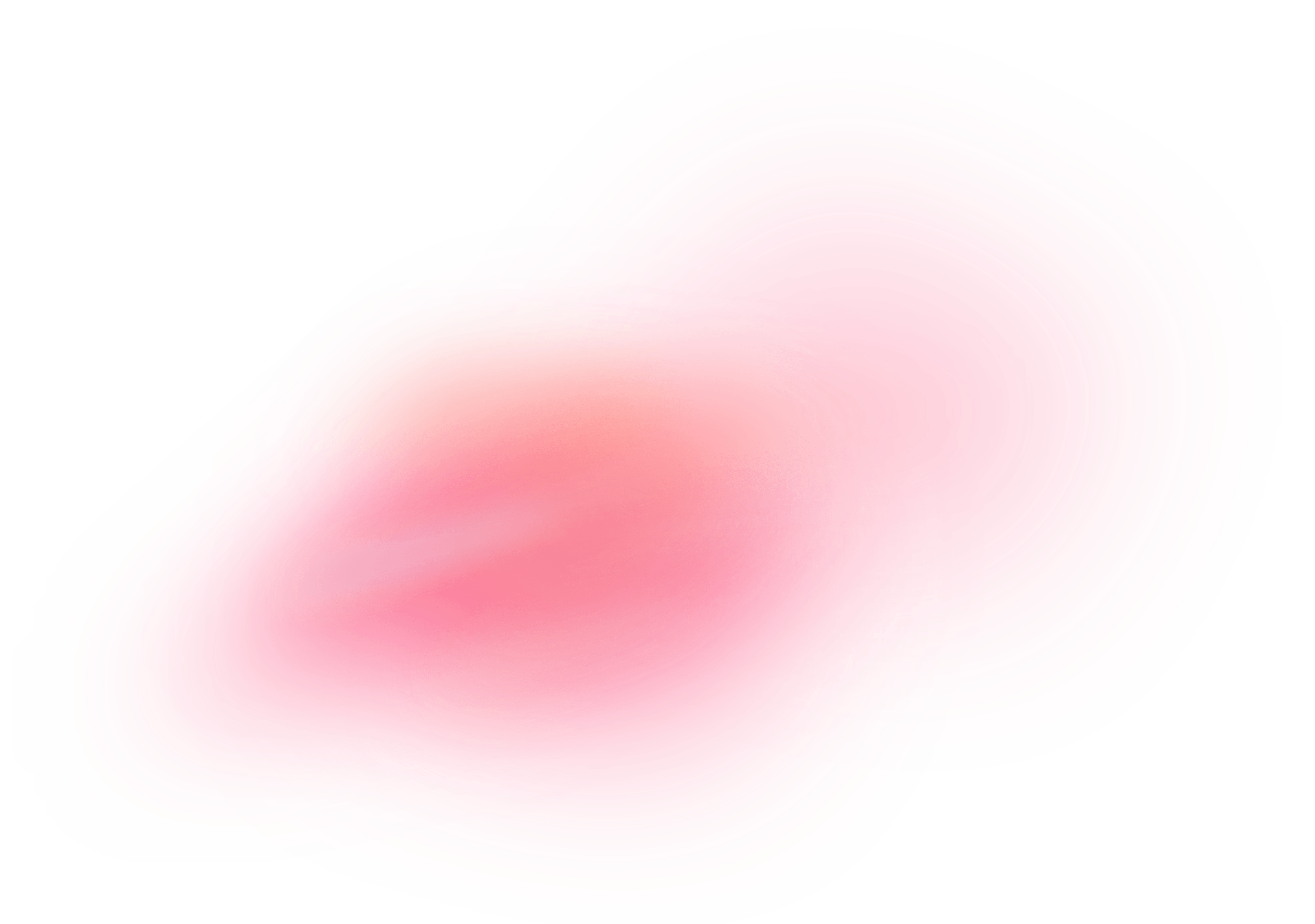