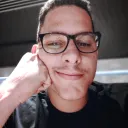
I am developing an SSR app using SvelteKit, in my app the user can create their account, or simply log in if they are already registered.
In both cases I follow the same implementation demonstrated in the SSR authentication tutorials provided in the documentation.
As in the example below 👇
import { SESSION_COOKIE, createAdminClient } from "$lib/server/appwrite.js"
import { redirect } from "@sveltejs/kit"
export const actions = {
login: async ({ request, cookies }) => {
const form = await request.formData()
const email = form.get("email") as string
const password = form.get("pass") as string
const { account } = createAdminClient()
const session = await account.createEmailPasswordSession(email, password)
cookies.set(SESSION_COOKIE, session.secret, {
sameSite: "strict",
expires: new Date(session.expire),
secure: true,
path: "/",
})
redirect(301, "/admin/meu-negocio")
},
}
The cookie that is stored during the login or account creation process is used to create a session client.
export function createSessionClient(cookies: Cookies) {
const session = cookies.get(SESSION_COOKIE)
if (!session) {
throw new Error("Não existe uma sessão válida")
}
const client = new Client()
.setEndpoint(variables.APPWRITE_ENDPOINT)
.setProject(variables.APPWRITE_PROJECT)
.setSession(session)
return {
get account() {
return new Account(client)
},
get databases() {
return new Databases(client)
},
}
}
After the first access everything works perfectly, in the middleware after instantiating the client I can access the logged in user, through the session client, but when I spend some time without using the app and return it is as if the session is no longer valid and I get the following error 👇
{
code: 401,
type: 'general_unauthorized_scope',
response: {
message: 'User (role: guests) missing scope (account)',
code: 401,
type: 'general_unauthorized_scope',
version: '1.5.7'
}
}
I've looked everywhere for a solution to this, or at least to understand what's happening, and I can't find it.
When I check my console, the user still has the session registered in the app and the sessions were configured to last 1 year. Which in this case already comes by default in Appwrite.
Could anyone help me with this?
Recommended threads
- The current user is not authorized to pe...
I want to create a document associated with user after log in with OAuth. The user were logged in, but Appwrite said user is unauthorized. User is logged in wi...
- self-hosted auth: /v1/account 404 on saf...
Project created in React/Next.js, Appwrite version 1.6.0. Authentication works in all browsers except Safari (ios), where an attempt to connect to {endpoint}/v1...
- Having issues with login via CLI
``` ~/appwrite appwrite login --endpoint https://localhost/v1 --verbose ? Enter your email myvalidemai...
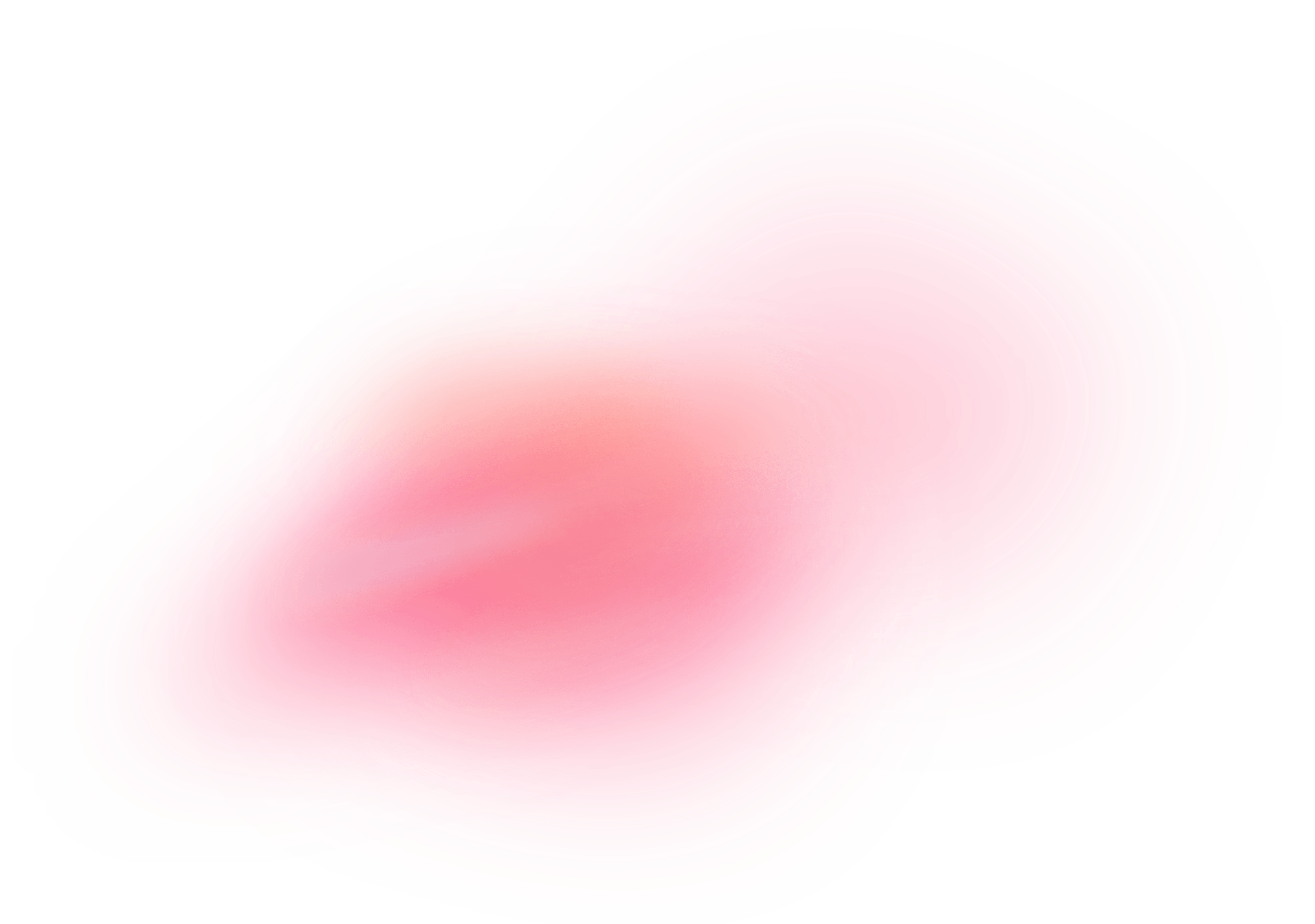