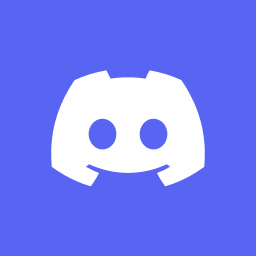
I set up a webhook that will receive image metadata that contains image URL. I convert it to a image buffer and try to upload it to Appwrite Storage, but I'm encountering errors. I upload it using the Appwrite SDK.
The Error happens at this line of code : "const inputFile = InputFile.fromBuffer(buffer, imageName);" TypeError: Cannot read properties of undefined (reading 'fromBuffer')
const express = require('express');
const bodyParser = require('body-parser');
require('dotenv').config();
const sdk = require('node-appwrite');
const { InputFile } = require('node-appwrite');
const https = require('https');
app.use(bodyParser.json({ limit: '50mb' }));
const client = new sdk.Client()
.setEndpoint(process.env.APPWRITE_API_ENDPOINT)
.setProject(process.env.APPWRITE_PROJECT_ID)
.setKey(process.env.APPWRITE_API_KEY);
const storage = new sdk.Storage(client);
const databases = new sdk.Databases(client);
function fetchImage(url) {
return new Promise((resolve, reject) => {
https.get(url, (response) => {
if (response.statusCode !== 200) {
reject(new Error(`Failed to fetch image: ${response.statusCode}`));
return;
}
const data = [];
response.on('data', (chunk) => {
data.push(chunk);
});
response.on('end', () => {
resolve(Buffer.concat(data));
});
}).on('error', reject);
});
}
async function uploadImage(imageUrl, imageName, bucketId = process.env.BUCKET_ID) {
try {
const buffer = await fetchImage(imageUrl);
console.log('Image fetched, buffer size:', buffer.length);
const inputFile = InputFile.fromBuffer(buffer, imageName);
const result = await storage.createFile(bucketId, sdk.ID.unique(), inputFile);
return result;
} catch (error) {
console.error("Error uploading image:", error);
throw error;
}
}
Recommended threads
- Appwrite Fra Cloud Custom Domains Issue
I’m trying to configure my custom domain appwrite.qnarweb.com (CNAME pointing to fra.cloud.appwrite.io with Cloudflare proxy disabled) but encountering a TLS ce...
- Appwrite service :: getCurrentUser :: Us...
Getting this error while creating a react app can someone please help me solve the error
- Storage & Database is not allowing.
Storage & Database is not allowing to CRUD after i have logged in ? Using web SDK with next.js without any SSR or node-sdk.
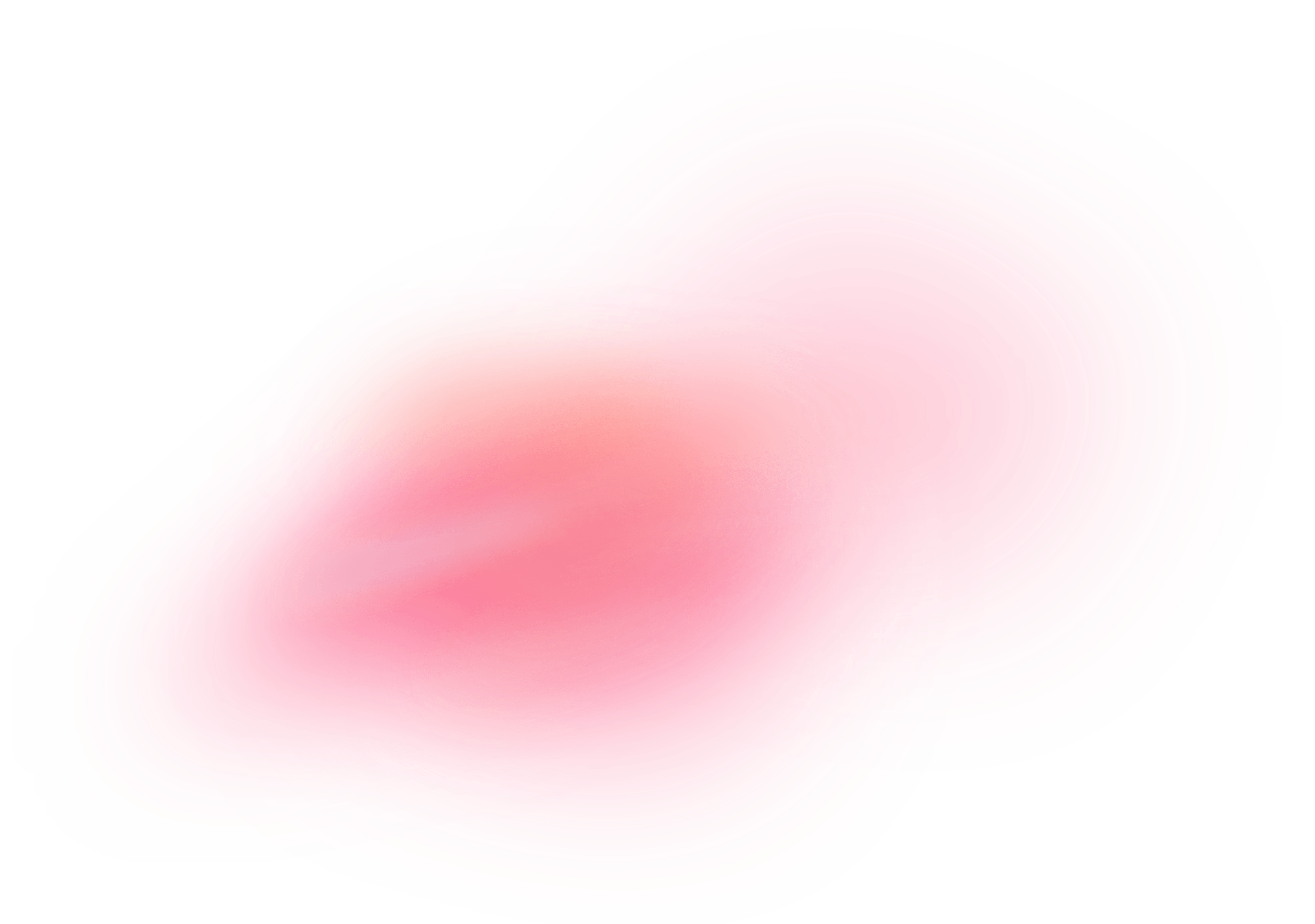