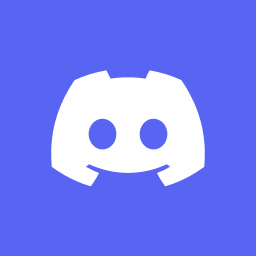
Hey everyone, I am trying to implement Magic URL into my NextJS project. I am having serious difficulty and I don't know what to do anymore.
In my api/ folder I have a function that looks like this:
export async function getMagicUrlToken(): Promise<Models.Token | Error> {
try {
return await account.createMagicURLToken(
ID.unique(),
'alexappleget2014@gmail.com',
'http://localhost:3000/auth/magicUrl',
);
} catch (error) {
console.error(error);
throw new Error('Error creating token');
}
}
This function is then imported into my App/Login folder inside the page.tsx file. For simple testing i made a simple button:
import {getMagicUrlToken} from '@/api/apiFunctions';
<button onClick={getMagicUrlToken}>Test Magic Url</button>
Up to this point it works fine. I click the button and get the email with the link.
Since in my api function i put 'http://localhost:3000/auth/magicUrl'
I went into my App/Auth/ folder and made another magicUrl/ folder with a file named route.ts. This is to handle the email link. Here is my code:
export async function GET(request: Request): Promise<Response> {
try {
const requestUrl = new URL(request.url);
const userId = requestUrl.searchParams.get('userId');
const secret = requestUrl.searchParams.get('secret');
if (!userId || !secret) {
return new NextResponse('Invalid URL parameters', { status: 400 });
}
const session = await account.updateMagicURLSession(userId, secret);
return NextResponse.redirect(requestUrl.origin);
} catch (error) {
console.error('Error processing request:', error);
return new NextResponse(
JSON.stringify({ error: 'Internal Server Error.' }),
{ status: 500 },
);
}
}
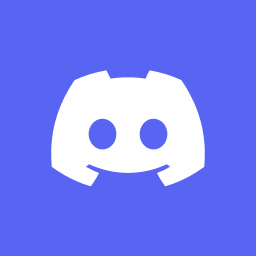
When I click the link it just takes me to my /Login page and fails. I have no idea why it's not grabbing the URL and the userId/secret values from the URL to create a session.
Oh also in my Api/ folder I have a config.ts file with this code:
import { Client, Account, Databases, ID } from 'appwrite';
const URL = process.env.NEXT_PUBLIC_APPWRITE_API_URL as string;
const PROJECT_ID = process.env.NEXT_PUBLIC_APPWRITE_PROJECT_ID as string;
const DATABASE_ID = process.env.NEXT_PUBLIC_APPWRITE_DATABASE_ID as string;
export const appwriteConfig = {
url: URL,
projectId: PROJECT_ID,
databaseId: DATABASE_ID,
};
export const client = new Client();
client.setEndpoint(appwriteConfig.url);
client.setProject(appwriteConfig.projectId);
export const account = new Account(client);
export const databases = new Databases(client);
export { ID };
Any help is much needed and thank you for helping 🙂
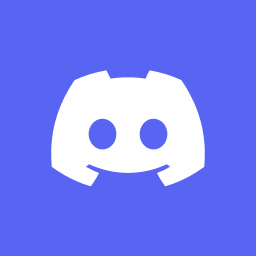
I also changed
return NextResponse.redirect(requestUrl.origin);
from my route.ts file to:
return NextResponse.redirect(`${requestUrl.origin}/auth/magicUrl`);
and I get a black screen that displays {"error":"Internal Server Error."}
in white letters in the top left so I know for sure the function is failing.
Recommended threads
- Email Verification Email
Hi everyone, I’m currently experiencing an issue with the email verification functionality. When I trigger the verification, the request returns a valid respon...
- Appwrite Cloud Custom Domains Issue
I’m trying to configure my custom domain api.kondri.lt (CNAME pointing to appwrite.network., also tried fra.cloud.appwrite.io with no luck ) but encountering a ...
- Persistent 401 Unauthorized on all authe...
Hello, I'm facing a critical 401 Unauthorized error on my admin panel app and have exhausted all debugging options. The Problem: When my React app on localhos...
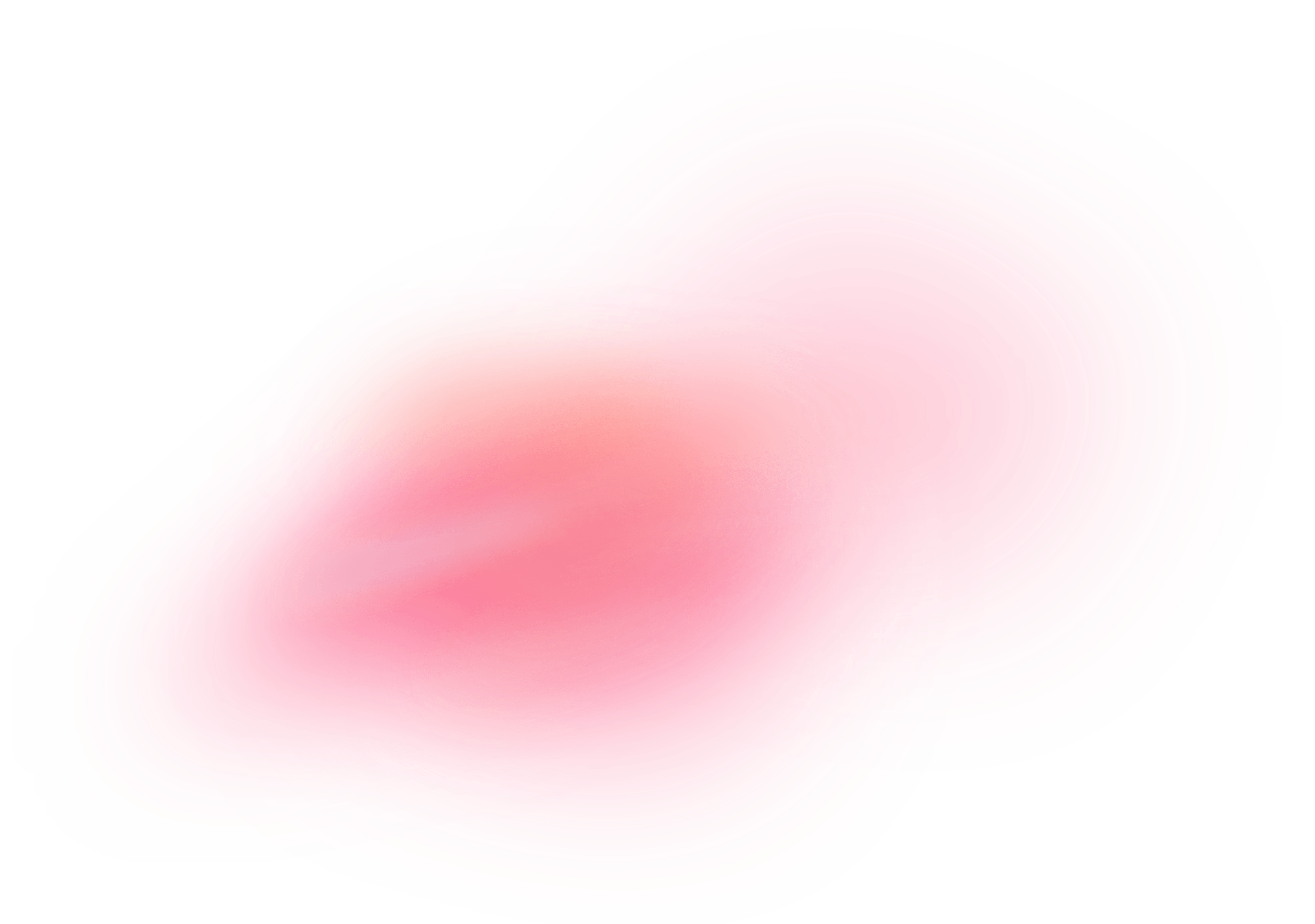