
AppwriteException: Invalid userId
param: Parameter must contain at most 36 chars. Valid chars are a-z, A-Z, 0-9, period, hyphen, and underscore. Can't start with a special char

import { Client, Account, ID, Avatars, Databases } from "react-native-appwrite";
export const config = {
// All my tokens here
}
// Init your React Native SDK
const client = new Client();
client
.setEndpoint(config.endpoint) // Your Appwrite Endpoint
.setProject(config.projectId) // Your project ID
.setPlatform(config.platform); // Your application ID or bundle ID.
const account = new Account(client);
const avatars = new Avatars(client);
const databases = new Databases(client);
export const signIn = async (email, password) => {
try {
const session = await account.createSession(email, password);
return session;
} catch (error) {
console.error(error);
throw new Error(error);
}
};
export const createUser = async (email, password, username) => {
console.log({ id: ID.unique() });
try {
const newAccount = await account.create(
ID.unique(),
email,
password,
username
);
if (!newAccount) {
throw new Error("Account creation failed");
}
const avatarUrl = avatars.getInitials(username);
await signIn(email, password);
const newUser = await databases.createDocument(
config.databaseId,
config.userCollectionId,
ID.unique(),
{
accountId: newAccount.$id,
email,
username,
avatar: avatarUrl,
}
);
return newUser;
} catch (error) {
console.error(error);
throw new Error(error);
}
};

what does the log
print? An id
or empty string?

{"id": "667141550036c56bf019"}

The ID is correct, and when I check the dashboard the user is created but it still give this exception
Recommended threads
- Login redirect going to http not https
Hi yall, I'm having an issue where the redirect URL is going to http, instead of https. I think this is a bug. ``` https://accounts.google.com/o/oauth2/v2/auth...
- Realtime got disconnected. Reconnect wil...
Can I please get assistance with this error. I looked at similar issues but none lead to a concrete solution. I am using Expo and this is my code.
- Google OAuth
I am having trouble using the google log in in my app. Getting error: Error 400 Invalid redirect URL for OAuth success. project_invalid_success_url I have tryi...
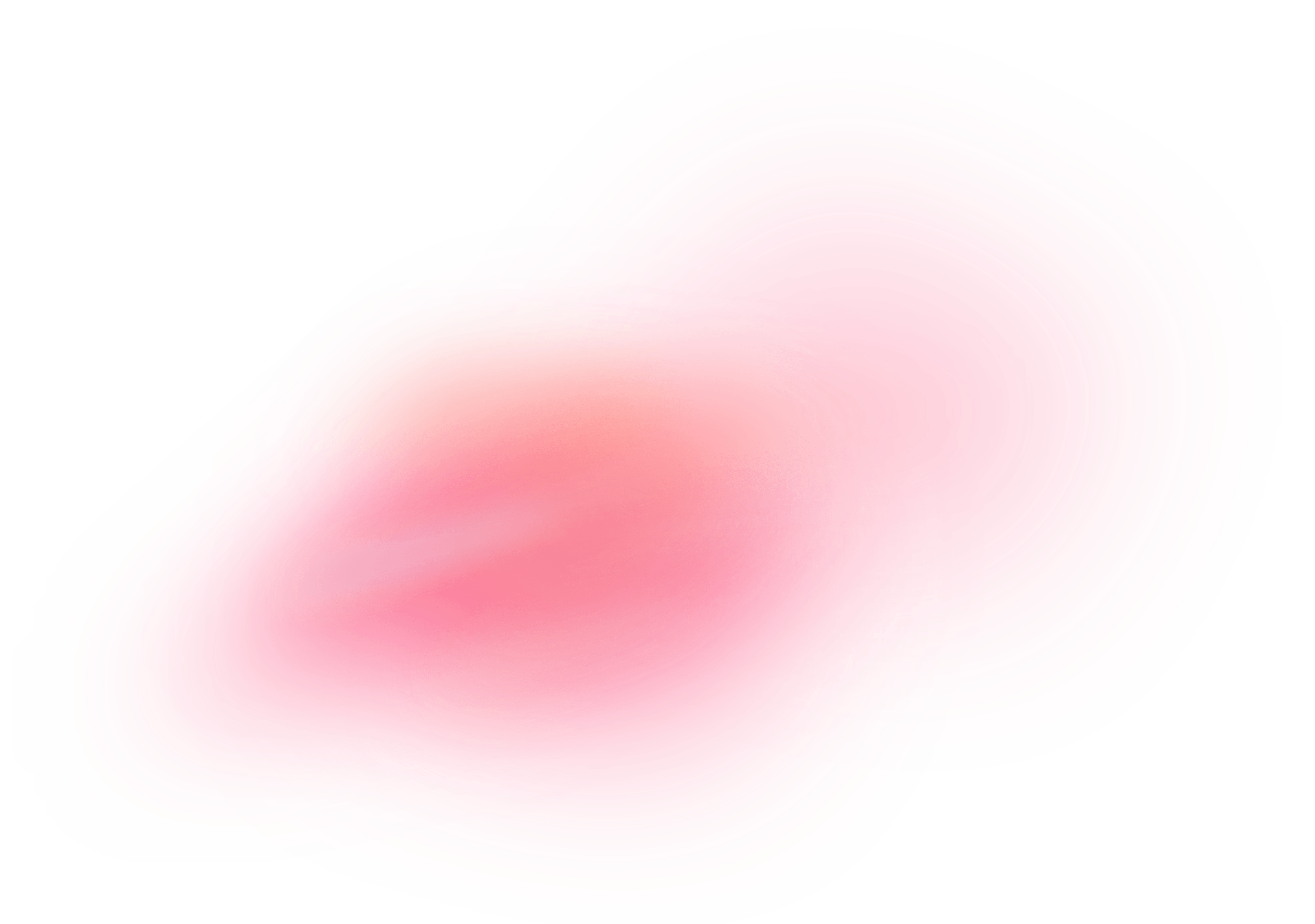