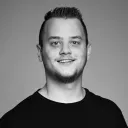
Hey there, thanks for reading this Q&A and trying to help!
Currently trying to develop a Fleet/Event Management application. For this there exists some domain entities which I am unsure how to properly model.
Basically there is an entity called "Event" and an "Order". An events contains e.g. an title, a short description about the event etc. Orders on the other hand contain information about what items are needed for that event and combines these items with a specific car.
So I have a relationship between an Event, which can contain many orders, and the other way around (an Order is related to an event).
I used Appwrite relationships to realize this (one-to-many, bidirectional). When fetching an Event, I get a list of bookings but obviously the "event" property of these bookings is not present/null. And the other way around - when fetching an order, the event's bookings dont contain the fetched order. From database/api level this make sense because otherwise we would have an endless loop but how should I define my models then?
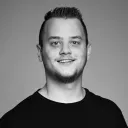
class Order with _$Order {
const Order._();
/// Default constructor for a new order.
const factory Order({
/// The unique identifier of the order.
@JsonKey(name: "\$id", includeToJson: false) String? id,
/// The status of the order.
@Default(OrderStatus.pending) OrderStatus status,
/// The event the order is for.
required Event event,
/// The list of items in the order.
@Default([]) List<OrderItem> items,
/// The messages regarding the order.
@Default([]) List<OrderMessage> messages,
/// The date when the order is scheduled to be loaded.
DateTime? scheduledLoadingDate,
/// The date when the order is scheduled to be offloaded.
DateTime? scheduledOffloadDate,
}) = _Order;
/// .....
}
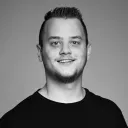
@freezed
class Event with _$Event {
const Event._();
factory Event({
/// The unique identifier of the event.
String? id,
/// The title of the event.
@Default("") String title,
/// The description of the event.
@Default("") String description,
/// The start date of the event.
required DateTime start,
/// The end date of the event.
required DateTime end,
/// The list of orders for the event.
@Default([]) List<Order> orders,
}) = _Event;
/// .....
}
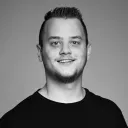
The problem is now that when fetching an Event, the fromJson is called of the Order Model to parse it but since the order is fetched as part of the event, its the orders' event property is missing and this leads to a parsing error.
Should i just make the "event" property nullable or is there a better way?
Recommended threads
- My account is blocked so please check an...
My account is blocked so please unblock my account because all the apps are closed due to which it is causing a lot of problems
- Applying free credits on Github Student ...
So this post is kind of related to my old post where i was charged 15usd by mistake. This happens when you are trying to apply free credits you got from somewh...
- Attributes Confusion
```import 'package:appwrite/models.dart'; class OrdersModel { String id, email, name, phone, status, user_id, address; int discount, total, created_at; L...
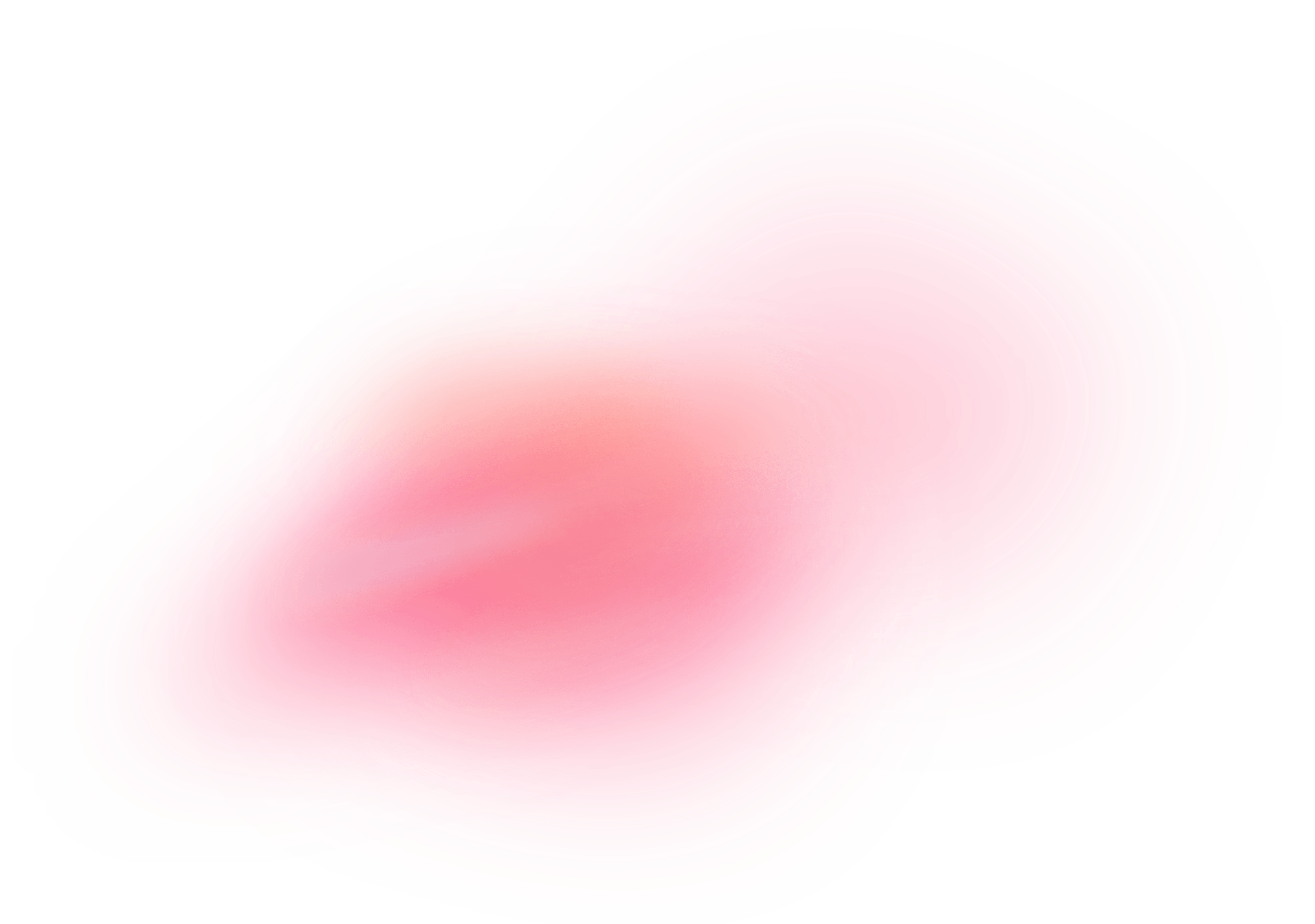