Having error " AppwriteException: Creation of a session is prohibited when a session is active. "
- 0
- Web
- Cloud
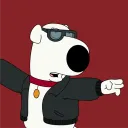
Im following a youtube tutorial to build a social media app with react js and appwrite, im at around the 2:02:00 time mark of this video: https://youtu.be/_W3R2VwRyF4?si=3ryU0cXS_laEBBAz When i try to sign up a new user im getting an error " AppwriteException: Creation of a session is prohibited when a session is active. at Client.<anonymous> (http://localhost:5173/node_modules/.vite/deps/appwrite.js?v=64b17d2a:893:17) at Generator.next (<anonymous>) at fulfilled (http://localhost:5173/node_modules/.vite/deps/appwrite.js?v=64b17d2a:488:24) " I have tried deleting all users in the appwrite to make sure im not resigning in with old account info, ive also tried clearing all of my cookies and cache data and signing up again and it still wont work. I will post code and more info in comments below
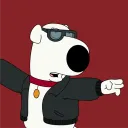
My Sign up form file
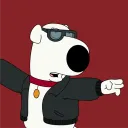
My queries and mutations file
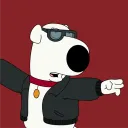
useQuery,
useMutation,
useQueryClient,
useInfiniteQuery,
} from '@tanstack/react-query'
import { createUserAccount, signInAccount } from '../appwrite/api'
import { INewUser } from '@/types'
export const useCreateUserAccount = () => {
return useMutation({
mutationFn: (user: INewUser) => createUserAccount(user)
})
}
export const useSignInAccount = () => {
return useMutation({
mutationFn: (user: {
email: string;
password: string;
}) => signInAccount(user),
})
} ```
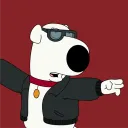
My Authcontext file
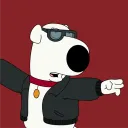
import { IContextType, IUser } from '@/types';
import { createContext, useContext, useEffect, useState } from 'react'
import { useNavigate } from 'react-router-dom';
export const INITIAL_USER = {
id: '',
name: '',
username: '',
email: '',
imageUrl: '',
bio: ''
};
const INITIAL_STATE = {
user: INITIAL_USER,
isLoading: false,
isAuthenticated: false,
setUser: () => {},
setIsAuthenticated: () => {},
checkAuthUser: async () => false as boolean,
}
const AuthContext = createContext<IContextType>(INITIAL_STATE);
const AuthProvider = ({ children }: { children: React.ReactNode }) => {
const [user, setUser] = useState<IUser>(INITIAL_USER);
const[isLoading, setisLoading] = useState(false);
const [isAuthenticated, setIsAuthenticated] = useState(false);
const navigate = useNavigate();
const checkAuthUser = async () => {
try {
const currentAccount = await getCurrentUser();
if(currentAccount) {
setUser({
id: currentAccount.$id,
name: currentAccount.name,
username: currentAccount.username,
email: currentAccount.email,
imageUrl: currentAccount.imageUrl,
bio: currentAccount.bio
})
setIsAuthenticated(true);
return true;
}
return false;
} catch (error) {
console.log(error);
return false;
} finally {
setisLoading(false);
}
};
useEffect(() => {
if(
localStorage.getItem('cookieFallback') === '[]' ||
localStorage.getItem('cookieFallback') === null
) navigate('/sign-in')
checkAuthUser();
}, []);
const value = {
user,
setUser,
isLoading,
isAuthenticated,
setIsAuthenticated,
checkAuthUser,
}
return (
<AuthContext.Provider value={value}>
{children}
</AuthContext.Provider>
)
}
export default AuthProvider
export const useUserContext = () => useContext(AuthContext); ```
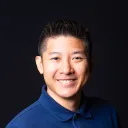
btw, from your console logs, it looks like you're using cloud and not self-hosting Appwrite.
you deleted users, but the sessions are deleted in the background (not right away) so they may still be around.
I would recommend checking if you have cookies still and also checking if you have a fallback cookie in local storage
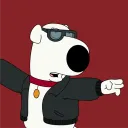
This is my first coding project so i apologize in advance if this is a stupid question, but how would i go about checking if i have cookies or a fallback cookie in local storage?
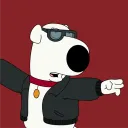
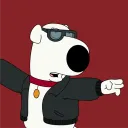
is this what you meant?
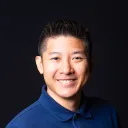
3rd party cookies is something else
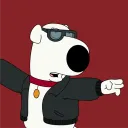
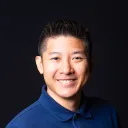
- go to cloud.appwrite.io
- open your browser's dev tools
- switch to the application tab
- click on the dropdown for Cookies
- click on
https://cloud.appwrite.io
- look for the cookie with name
a_session_<YOUR PROJECT ID>
- delete the cookie from step 6
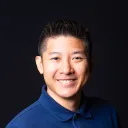
cookies are tied to the domain on the cookie so you won't see it here under the domain for your app
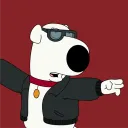
I followed all of those steps and am still receiving error
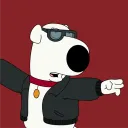
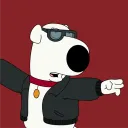
I deleted the one with my project ID as the number, a_session_6650fe91000729591588 is a different one.
Recommended threads
- Collection Permission issue
I am facing issue in my Pro account. "Add" button is disabled while adding permission in DB collection settings.
- Opened my website after long time and Ba...
I built a website around a year back and and used appwrite for making the backend. At that time the website was working fine but now when i open it the images a...
- Is it possible to cancel an ongoing file...
When uploading a file to storage, is there a way to cancel the upload in progress so the file is not saved or partially stored?
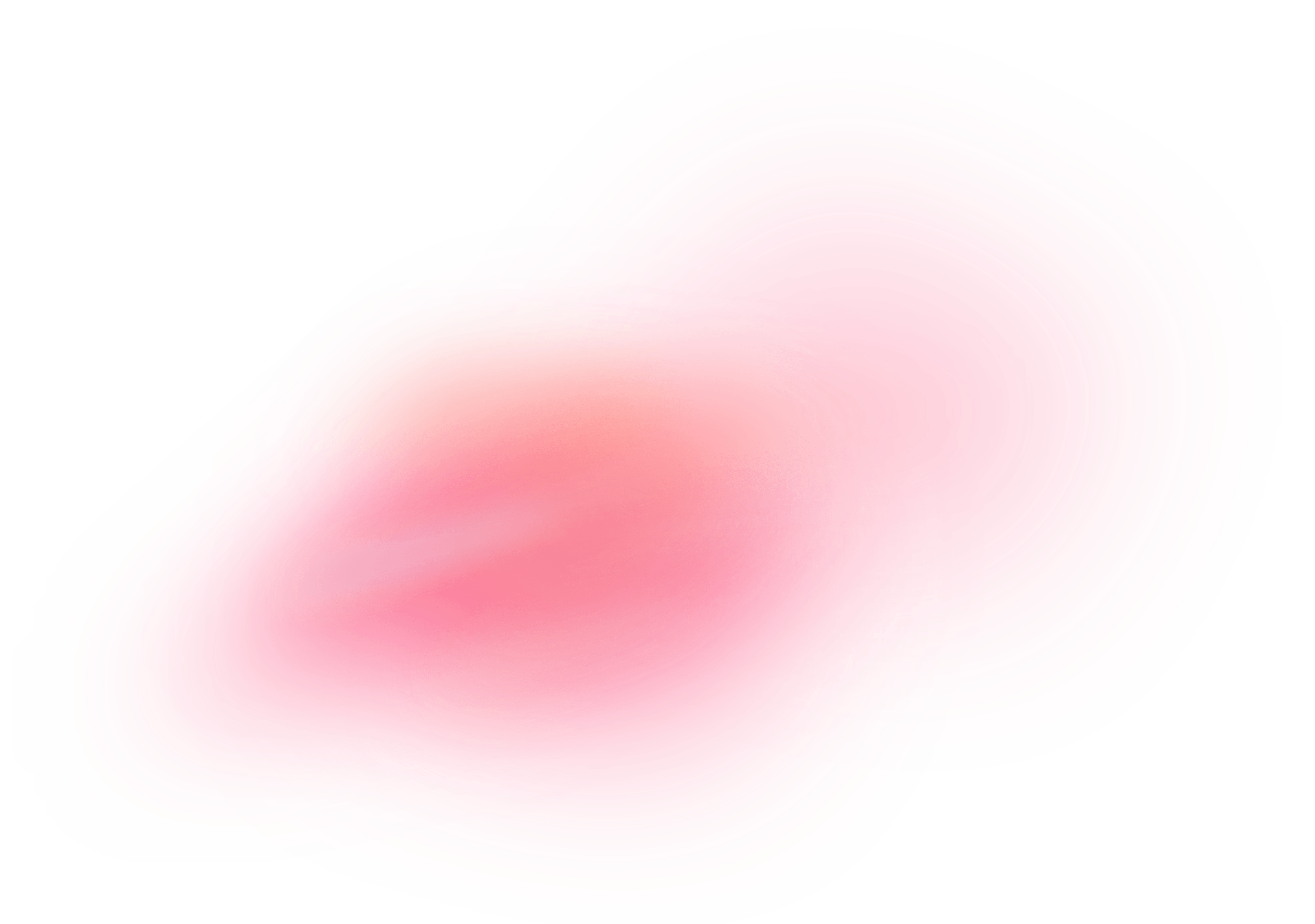