
Im trying to grab a 2GB file from my storage using the API and Fetch.
const response = await fetch(`/api/download?fileID=664dfbbf6b74a086ac89`);
if (!response.ok) {
console.error("Failed to download file");
setIsDownloading(false);
return;
}
const contentLength = response.headers.get("content-length");
const total = parseInt(contentLength, 10);
let loaded = 0;
const reader = response.body.getReader();
const stream = new ReadableStream({
start(controller) {
function push() {
reader.read().then(({ done, value }) => {
if (done) {
controller.close();
setDownloadCompleted(true);
setIsDownloading(false);
return;
}
loaded += value.length;
setProgress((loaded / total) * 100);
controller.enqueue(value);
push();
});
}
push();
},
});
const newResponse = new Response(stream);
const blob = await newResponse.blob();
const url = window.URL.createObjectURL(blob);
const a = document.createElement("a");
a.href = url;
a.download = "world.rar"; // Set the filename here
document.body.appendChild(a);
a.click();
a.remove();
};

API:
import { Storage } from "node-appwrite";
import { createAdminClient } from "@/utils/node-appwrite";
import { NextResponse } from 'next/server';
export async function GET(req) {
const storage = new Storage(await createAdminClient());
const url = new URL(req.url, `http://${req.headers.get('host')}`);
const fileID = url.searchParams.get("fileID");
try {
const file = await storage.getFileView(process.env.NEXT_PUBLIC_WORLD_STORAGE, fileID);
const headers = new Headers();
headers.set('Content-Disposition', `attachment; filename="world.rar"`);
headers.set('Content-Type', 'application/octet-stream');
return new NextResponse(file, { headers });
} catch (e) {
console.error(e);
return new NextResponse(JSON.stringify({ error: "Failed to download file" }), { status: 500 });
}
}

It works but using storage.getFileView takes almost 2 minutes before it even starts downloading. Is there a way i can limit the download rate in app but raise the speed at which the file shows up in the download manager of the browser?
Recommended threads
- Origin error after changing default port...
Hi! I need some help regarding an issue I’m facing with Appwrite after changing the default ports. I have a self-hosted Appwrite instance running on my VPS. I ...
- Opened my website after long time and Ba...
I built a website around a year back and and used appwrite for making the backend. At that time the website was working fine but now when i open it the images a...
- Is it possible to cancel an ongoing file...
When uploading a file to storage, is there a way to cancel the upload in progress so the file is not saved or partially stored?
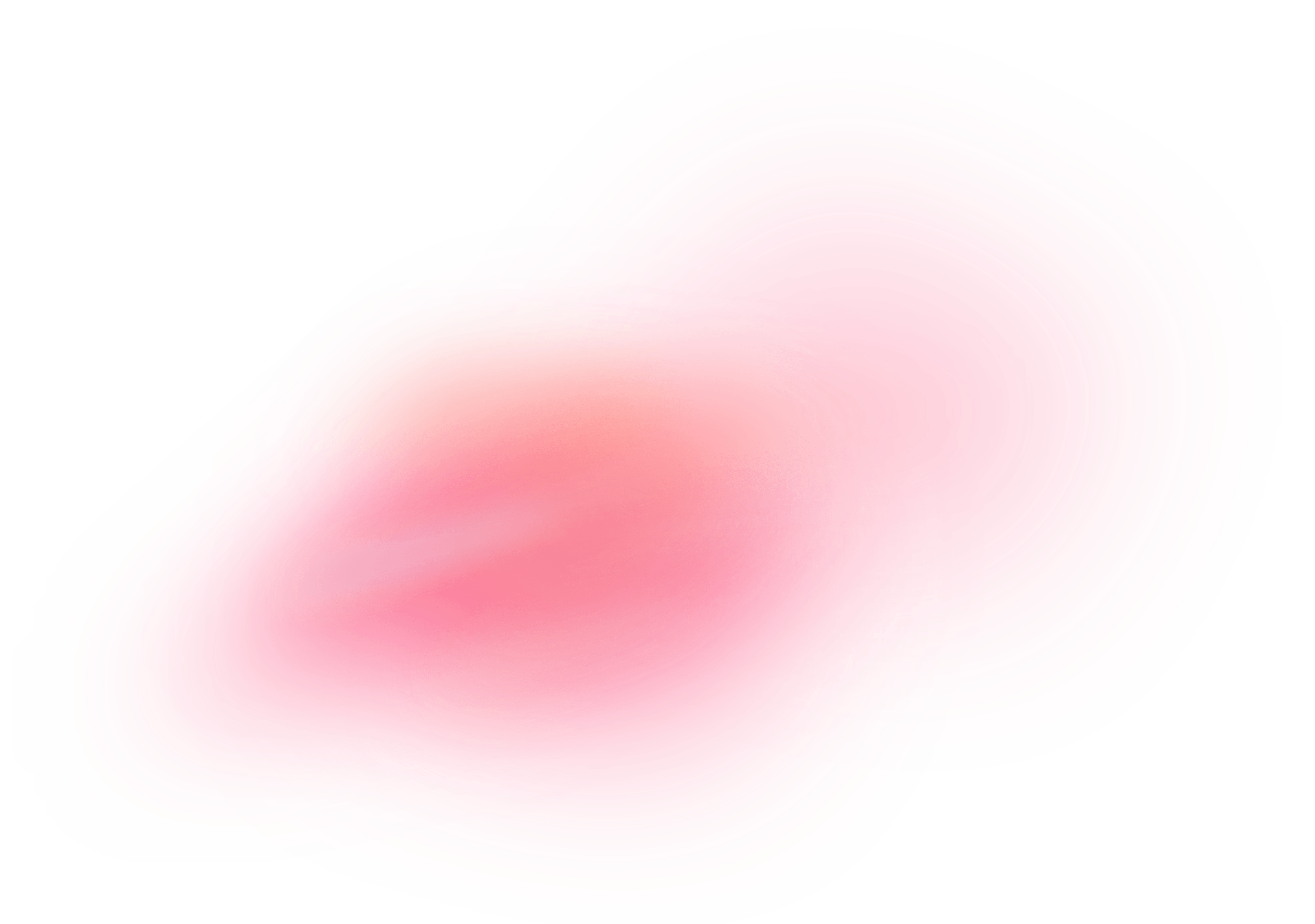