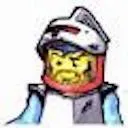
Appwrite 1.5.5
Goal of function: See if computer_id and action_id exist in completed collection. Issue: If computer_id or action_id is anywhere in any of the docs, it will return all docs. I was using relationships before for the action and device but had the same issue. Changed to string to see if issue continued and it did.
Computer1: aaa Computer2: bbb
Action1: 111 Action2: 222
Completed Docs: Doc1: deviceAction: aaa_111 Doc2: deviceAction: bbb_222
Function runs with aaa_111, returns Doc1 Function runs with aaa_222, returns Doc1 and Doc2
let device_action_value = format!("{}_{}", computer_id, action_id);
let query = json!({
"values": [
{
"method": "equal",
"attribute": "deviceAction",
"values": device_action_value
}
]
});
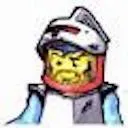
Function: pub async fn check_completed( computer_id: &str, action_id: &str, ) -> Result<bool, Box<dyn std::error::Error>> { let client = Client::new();
let device_action_value = format!("{}_{}", computer_id, action_id);
let query = json!({
"values": [
{
"method": "contains",
"attribute": "deviceAction",
"values": device_action_value
}
]
});
println!("Constructed Query: {}", query);
let request_url = format!(
"{}/databases/{}/collections/{}/documents",
URL, DATABASE_ID_ACTIONS, COLLECTION_ID_ACTIONS
);
let response = client
.get(&request_url)
.header("X-Appwrite-Project", PROJECT_ID)
.header("X-Appwrite-Key", API_KEY)
.header("Content-Type", "application/json")
.json(&query)
.send()
.await?;
let status = response.status();
let response_text = response.text().await?;
println!("Response Status: {}", status);
println!("Response Text: {}", response_text);
if status.is_success() {
let completed_data: serde_json::Value = serde_json::from_str(&response_text)?;
if let Some(documents) = completed_data["documents"].as_array() {
println!("Number of Documents Found: {:?}", documents.len());
return Ok(!documents.is_empty());
}
Ok(false)
} else {
Err(format!("Failed to fetch documents: {} - {}", status, response_text).into())
}
}
Recommended threads
- apple exchange code to token
hello guys, im new here π I have created a project and enabled apple oauth, filled all data (client id, key id, p8 file itself etc). I generate oauth code form...
- Send Email Verification With REST
I am using REST to create a user on the server side after receiving form data from the client. After the account is successfully created i wanted to send the v...
- Use different email hosts for different ...
Hello, I have 2 projects and i want to be able to set up email templates in the projects. Both projects will have different email host configurations. I see ...
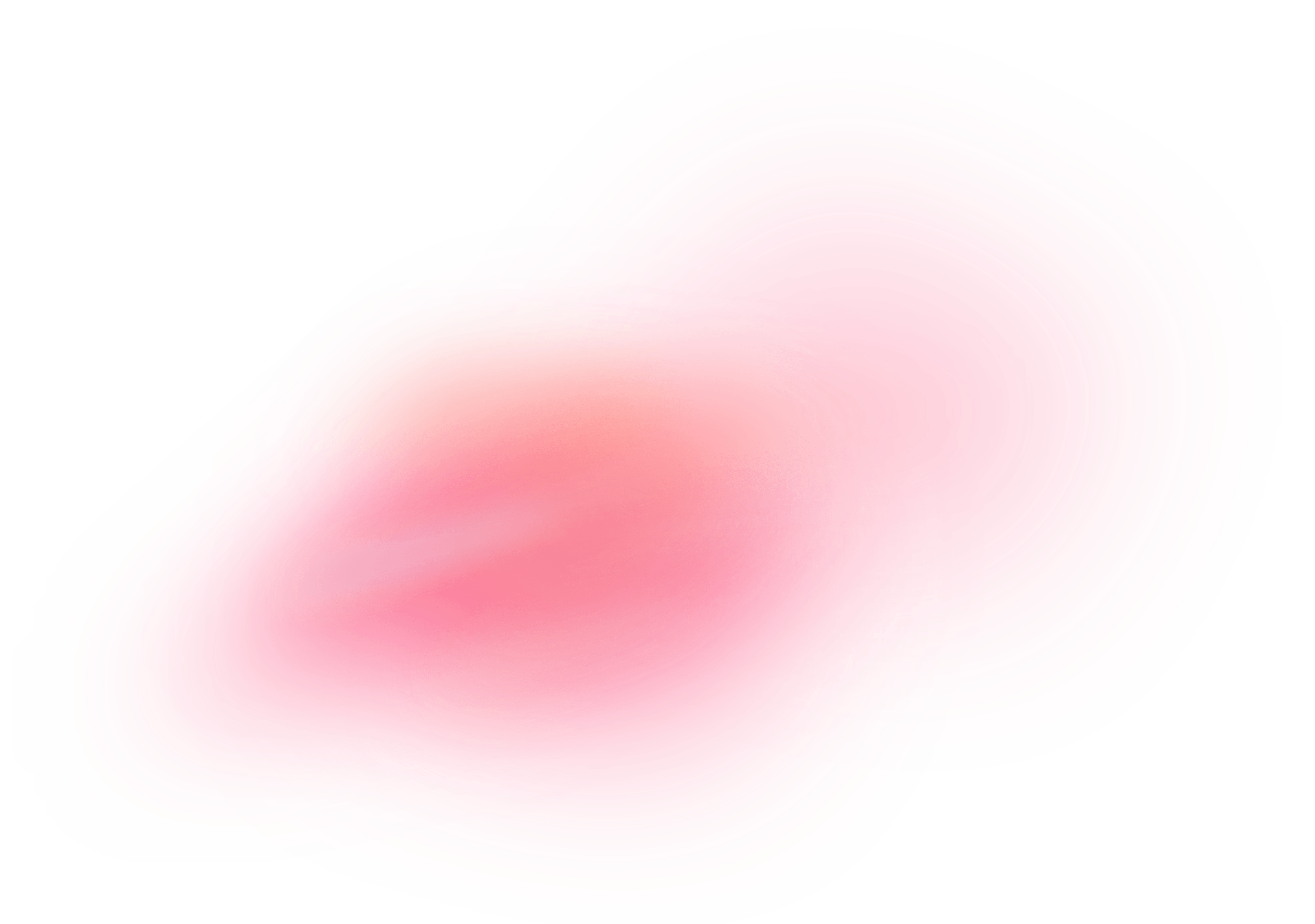