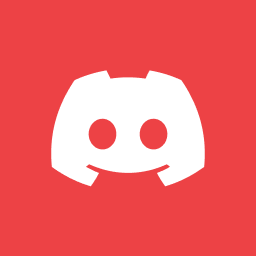
I have an app that starts with users signing up and verifying their account. For some reason, only on the first "sign up" by a new user after the app is installed the permissions do not update after calling the updateVerification and getting the account. I have an appwrite function that adds a new user to a team on the verification event. I know this has something to do with permissions because I have changed the permissions through the appwrite console to allow guests to read and at step 3 I can get the documents.
A simplified outline of the process:
- App installed
- User signs up and verifies account
- Appwrite function adds new user to team
- AppwriteException: document_not_found
- Delete user from appwrite from console
- Sign up again and verify account
- Appwrite function adds new user to team
- Can read documents from step 3 (as should be with permissions set)
Here is the code (Flutter)
Future<void> setUser() async {
try {
this._currentUser.value = await this.account.get();
this._currentUser.notifyListeners();
authState.value = AuthState.authenticated;
}
catch (e) {
this._currentUser.value = null;
authState.value = AuthState.unauthenticated;
}
authState.notifyListeners();
}
Future<bool> confirmEmailVerification({required String userId, required String secret, required String dbid}) async {
try{
await this.account.updateVerification(userId: userId, secret: secret);
await this.setUser();
if (this._currentUser.value == null){
throw Exception("No current user");
}
return true;
}
catch (e) {
print("Error updating verification: " + e.toString());
return false;
}
}
Appwrite function
# Create client (endpoint, project, apikey)...
teams = Teams(client)
teams.create_membership(
team_id = os.environ['TEAM_ID'],
roles = [os.environ['USER_ROLE']],
user_id = context.req.body[os.environ['USER_ID']]
)
Any insights/thoughts are greatly appreciated
Recommended threads
- Storage & Database is not allowing.
Storage & Database is not allowing to CRUD after i have logged in ? Using web SDK with next.js without any SSR or node-sdk.
- API Endpoint to Verify Password.
I have 2 use cases where i need to verify a users password outside of login, e.g. Updating user account data (such as name, or prefs, or data in a users databa...
- login backend and frontend
I'm using Remix, as my Backend (node). and react on my frontend. Until now, i did SSR login. saved the secret.. and it's fine. For many simpler requests i wish...
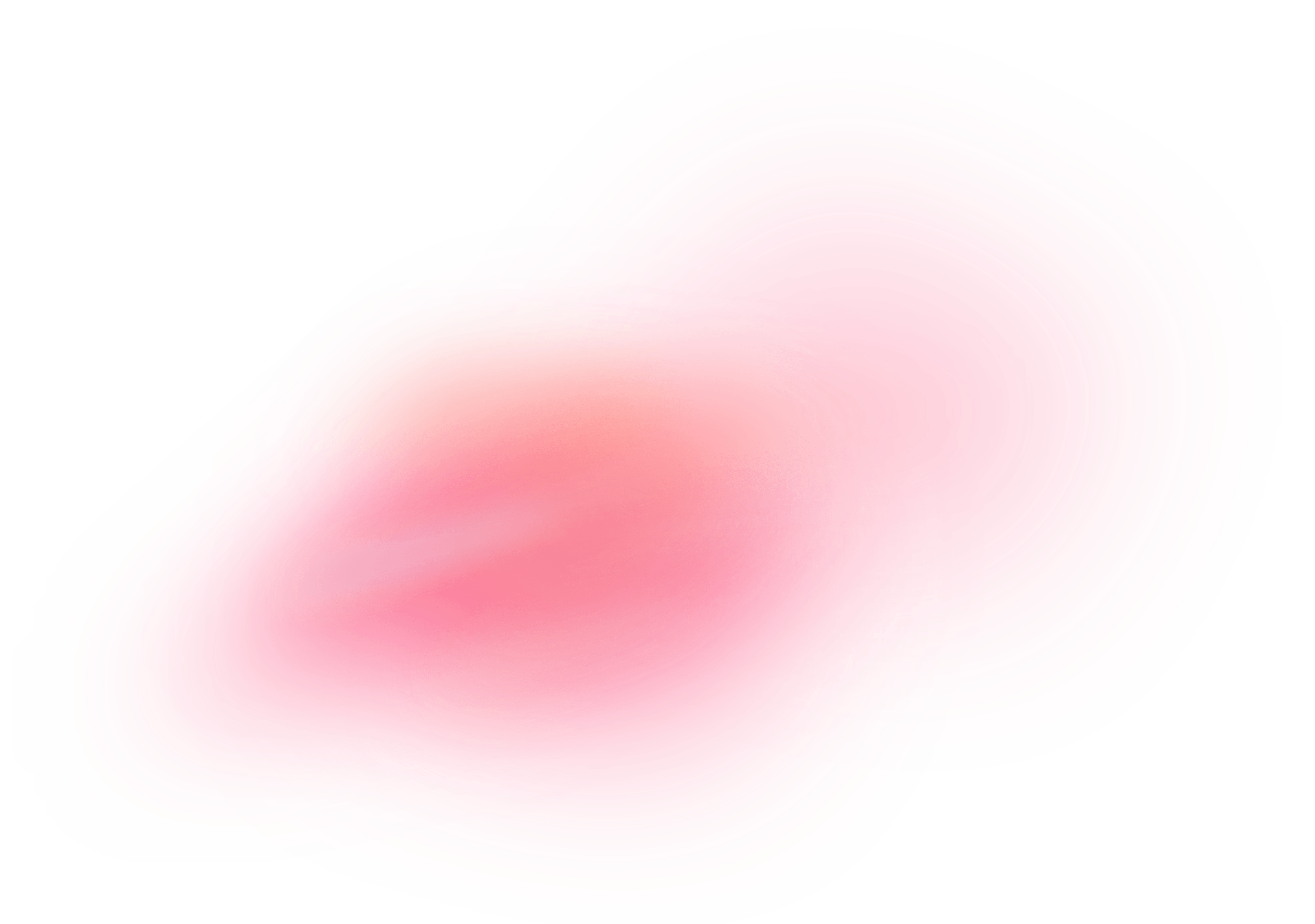