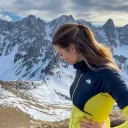
Hello, I'm trying to implement Magic Link Auth on a Flutter App, and I have two fundamental questions:
- How can I test the magic link on Android Emulator (I don't have a physical Android device)? The link is being sent to the email, but then I have the screen "Missing redirect URL". I've implemented deep linking and routing (will send the routing in the next message otherwise I exceed the 2000 chars limit):
<meta-data android:name="flutter_deeplinking_enabled" android:value="true" />
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<data
android:scheme="https"
android:host="cloud.appwrite.io"
android:pathPrefix="/auth/magic-url" />
</intent-filter>
- I've implemented Deep Linking on iOS as well, but both on the Simulator and my iPhone I also receive see the "Missing redirect URL" screen. To implement Deel Linking, I followed the instructions on docs.flutter.dev for universal links for iOS:
- I've added
FlutterDeepLinkingEnabled
with a Boolean valueYES
in Xcode. - I've set
applinks:cloud.appwrite.io
as an associated domain in Xcode.
What am I missing? 🫠Thanks!
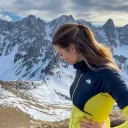
Routing & sending over the magic link:
// router implementation
final _router = GoRouter(debugLogDiagnostics: true, routes: [
GoRoute(
path: '/',
builder: (BuildContext context, GoRouterState state) {
return const SplashScreen();
},
routes: [
GoRoute(
path: 'auth/magic-url',
builder: (context, GoRouterState state) {
return OnboardingScreen(
userId: state.uri.queryParameters['userId'] ?? '',
secret: state.uri.queryParameters['secret'] ?? '',
);
},
)
],
)
]);
@override
Widget build(BuildContext context) {
return MaterialApp.router(
theme: RemindrTheme.themeData,
routerConfig: _router,
);
}
// creating Magic Link
void _submit() async {
try {
await account.createMagicURLSession(
userId: ID.unique(),
email: _enteredEmail,
);
_openConfirmationModal();
} catch (e) {
print(e);
}
return;
}
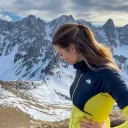
Ok, will try implementing it for Android, but still not sure if it's testable on emulator.
From what I saw in the other issues here, it's not, but I'm already very confused.
What about iPhone? I added in Info.plist this:
<array>
<string>appwrite-callback-[my project id]</string>
</array>
and I still get the same message.
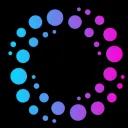
<key>CFBundleURLSchemes</key>
<array>
<string>appwrite-callback-auth-[PROJECT_ID]</string>
</array>
added the key?
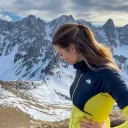
yes
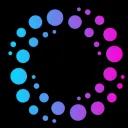
https://www.appwriters.dev/blog/flutter-password-less-authentication-with-appwrite-magic-url refer this for ios if you don't have the url then do not insert url parameter
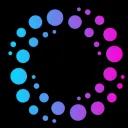
and how did you written the project id
appwrite-callback-auth-6r774dfbfggf67676
this is the correct way
many people write it in wrong way like this
appwrite-callback-auth-[6r774dfbfggf67676]
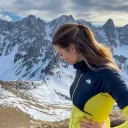
I followed the guide, and I continue having the same problem. I tried:
- on Android emulator;
- on iOS simulator;
- in debug mode on my iPhone;
- through TestFlight on my iPhone.
From what I've read in other similar issues, I have to implement deep linking - and I tried to do it (I created this issue after trying it), but it was causing the exact same problem.
Appwrite just seems not to be done for mobile developers... I don't know what else to do.
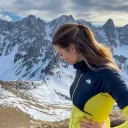
Here is a similar issue: https://discord.com/channels/564160730845151244/1147562701007098007... I tried to follow that steps, but still nothing.
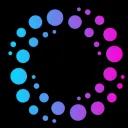
Then repost the problem mentioning @D5
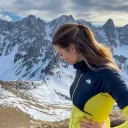
Thank you @Saurabh Actually, it was @D5 who suggested me Appwrite on the Flutter server 😅
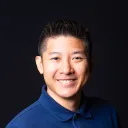
This might be outdated
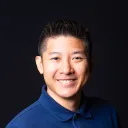
You must pass a url that will link to your route in the app that takes the secret and user id and calls the update method
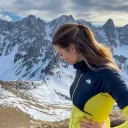
I don't have my own domain. What should I write then?
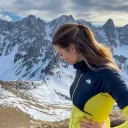
Also, in the documentation it is written If you are on a mobile device you can leave the URL parameter empty, so that the login completion will be handled by your Appwrite instance by default.
Is it outdated as well?
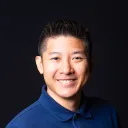
You might need to use a custom url scheme. Look online
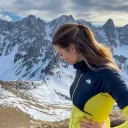
To sum up: you have an outdated documentation, you don’t know what people with no domain should do to use your Magic Link Auth (btw, on Firebase it took me just an hour to implement it - with Appwrite I’m about to hit the third day), and I should find a solution online? 😅
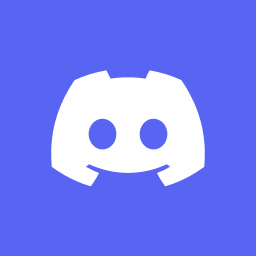
You can use appwrite functions for the deep link URL
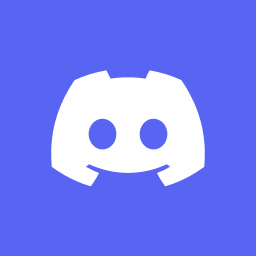
Basically you can get the same as firebase dynamic links: https://github.com/appwrite/dynamic-links
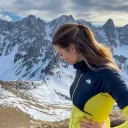
Ok, will give it a try, thank you
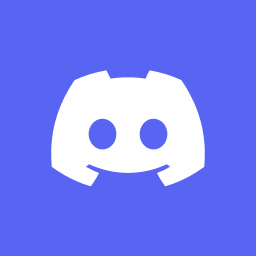
You're welcome. I can build you an example if needed so it's easier to understand 🙂
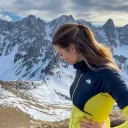
If it’s not a problem for you, I will really appreciate it, thank you.
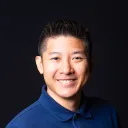
On firebase, are you using Firebase dynamic links?
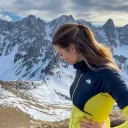
Yes.
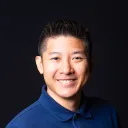
Did you see the deprecated notice?
https://firebase.google.com/docs/dynamic-links/flutter/receive
Recommended threads
- Appwrite documents and Swift codable
The object I use to create a document is different than the object I receive when I list documents. Do I create an object that’s used to create a document and a...
- Redirect URL sends HTTP instead of HTTPS...
I am not sure since when this issue is present, but my Google and Apple redirect URI are no longer pointing to the HTTPS redirect URI when I try to use OAuth. ...
- Console is not opening
Plz solve this issue console is not opening
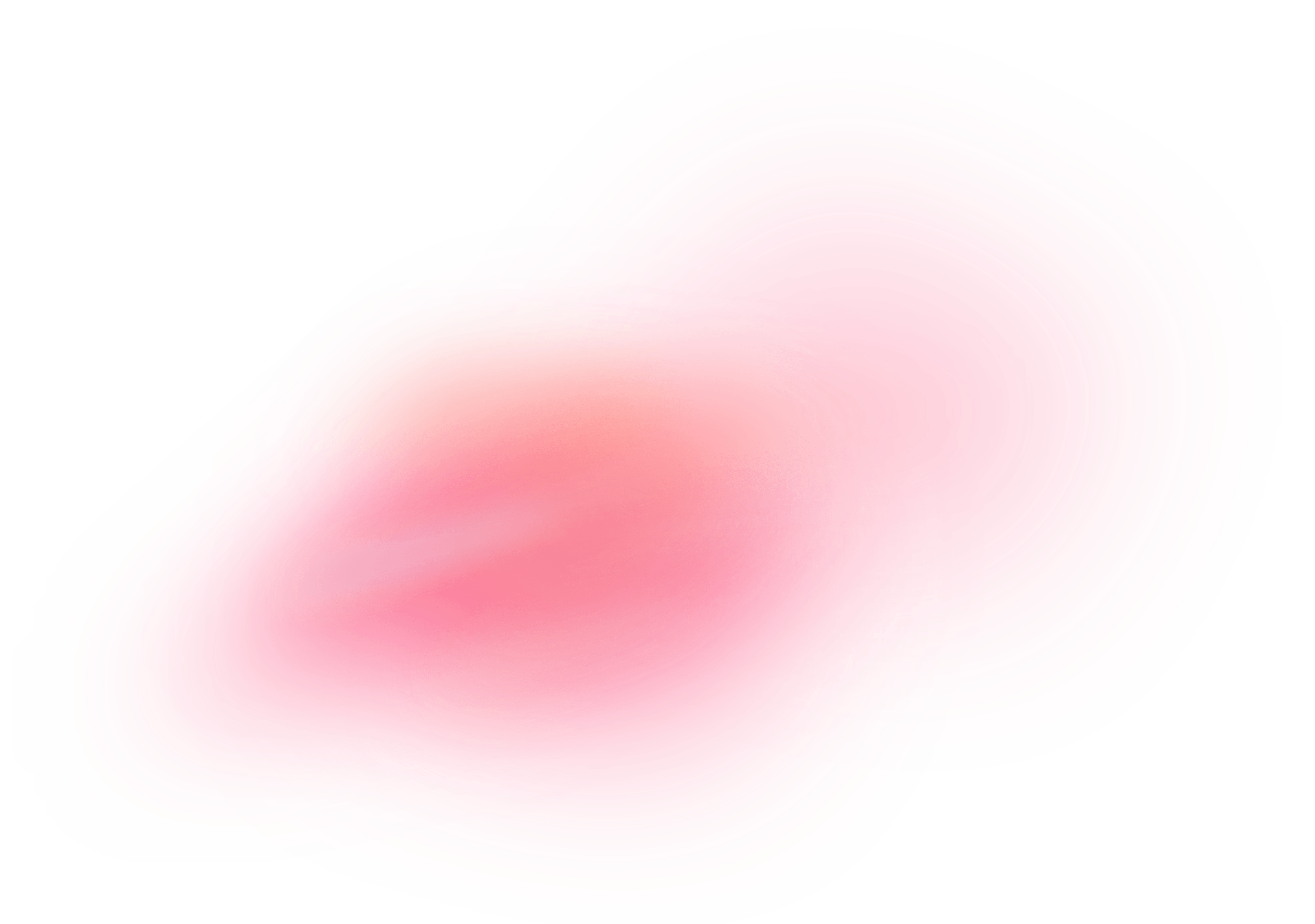