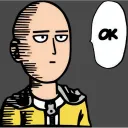
Hello fellas, I am trying to use Appwrite with nextjs middleware to check the auth status. giving a weird error
Module build failed: UnhandledSchemeError: Reading from "node:stream" is not handled by plugins (Unhandled scheme).
Webpack supports "data:" and "file:" URIs by default.
You may need an additional plugin to handle "node:" URIs.
my middleware
import { NextRequest, NextResponse } from 'next/server'
import { getLoggedInUser } from './lib/auth'
// 1. Specify protected and public routes
const protectedRoutes = ['/dashboard']
const publicRoutes = ['/login', '/signup', '/']
export default async function middleware(req: NextRequest) {
// 2. Check if the current route is protected or public
const path = req.nextUrl.pathname
const isProtectedRoute = protectedRoutes.includes(path)
const isPublicRoute = publicRoutes.includes(path)
// 3. Decrypt the session from the cookie
const session = await getLoggedInUser()
// 5. Redirect to /login if the user is not authenticated
if (isProtectedRoute && !session?.$id) {
return NextResponse.redirect(new URL('/login', req.nextUrl))
}
// 6. Redirect to /dashboard if the user is authenticated
if (
isPublicRoute &&
session?.$id &&
!req.nextUrl.pathname.startsWith('/dashboard')
) {
return NextResponse.redirect(new URL('/dashboard', req.nextUrl))
}
return NextResponse.next()
}
// Routes Middleware should not run on
export const config = {
matcher: ['/((?!api|_next/static|_next/image|.*\\.png$).*)'],
}```
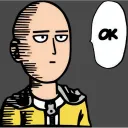
the auth functions
"use server";
import { Client, Account } from "node-appwrite";
import { cookies } from "next/headers";
export async function createSessionClient() {
const client = new Client()
// @ts-ignore
.setEndpoint(process.env.NEXT_PUBLIC_APPWRITE_ENDPOINT)
// @ts-ignore
.setProject(process.env.NEXT_PUBLIC_APPWRITE_PROJECT);
const session = cookies().get("my-custom-session");
if (!session || !session.value) {
throw new Error("No session");
}
client.setSession(session.value);
return {
get account() {
return new Account(client);
},
};
}
export async function getLoggedInUser() {
try {
const { account } = await createSessionClient();
return await account.get();
} catch (error) {
return null;
}
}
Recommended threads
- Expo-web session issue
In web I created a session using oauth. I run the app logged in but closed the terminal and ran again opened the app(I have now 2 running app in different...
- Self hosting password reset flow V1.7.4
Hi all, I am having trouble making a password reset flow that works for self hosted. I know it has the fuunctionality but I keep getting errors: The probl...
- Import Css glitsch?
Hey, Seems to be not only my computer, but some other i know - Seems that there's a css error When migrating, the input checkbox, and selectbox are.. out of st...
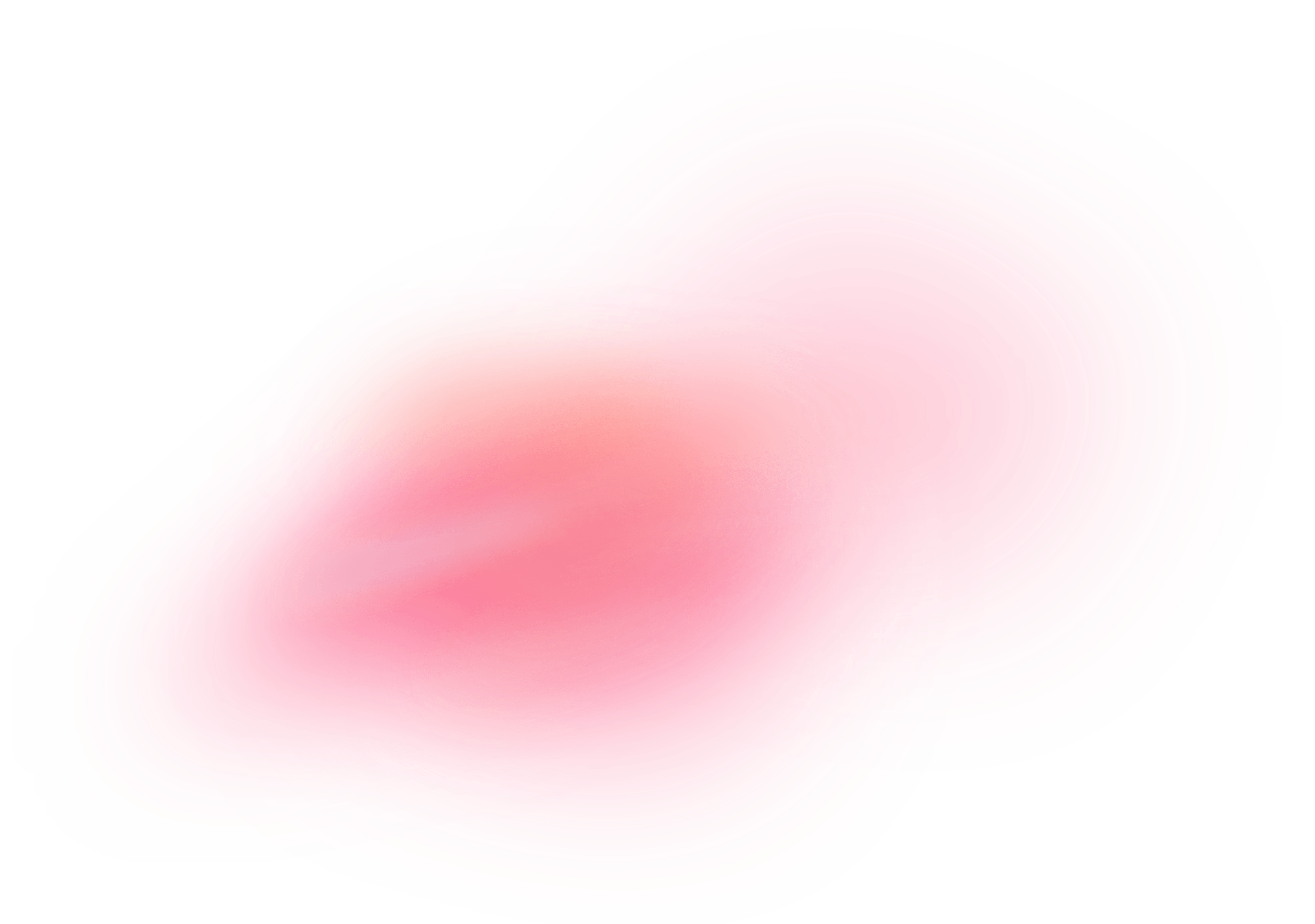